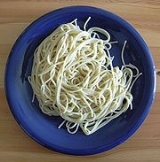
Spaghetti code
Encyclopedia
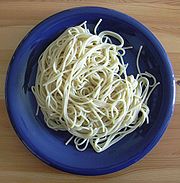
Pejorative
Pejoratives , including name slurs, are words or grammatical forms that connote negativity and express contempt or distaste. A term can be regarded as pejorative in some social groups but not in others, e.g., hacker is a term used for computer criminals as well as quick and clever computer experts...
term for source code
Source code
In computer science, source code is text written using the format and syntax of the programming language that it is being written in. Such a language is specially designed to facilitate the work of computer programmers, who specify the actions to be performed by a computer mostly by writing source...
that has a complex and tangled control structure, especially one using many GOTO
Goto
goto is a statement found in many computer programming languages. It is a combination of the English words go and to. It performs a one-way transfer of control to another line of code; in contrast a function call normally returns control...
s, exceptions, threads, or other "unstructured" branching
Branch (computer science)
A branch is sequence of code in a computer program which is conditionally executed depending on whether the flow of control is altered or not . The term can be used when referring to programs in high level languages as well as program written in machine code or assembly language...
constructs. It is named such because program flow tends to look like a bowl of spaghetti, i.e. twisted and tangled. Spaghetti code can be caused by several factors, including inexperienced programmers and a complex program which has been continuously modified over a long life cycle. Structured programming
Structured programming
Structured programming is a programming paradigm aimed on improving the clarity, quality, and development time of a computer program by making extensive use of subroutines, block structures and for and while loops - in contrast to using simple tests and jumps such as the goto statement which could...
greatly decreased the incidence of spaghetti code.
Examples
Below is what would be considered a trivial example of spaghetti code in BASIC. The program prints the numbers 1 to 10 to the screen along with their square. Notice that indentation is not needed and that the program'sGOTOGotogoto is a statement found in many computer programming languages. It is a combination of the English words go and to. It performs a one-way transfer of control to another line of code; in contrast a function call normally returns control...
statements create a reliance on line numberLine number
In computing, a line number is a method used to specify a particular sequence of characters in a text file. The most common method of assigning numbers to lines is to assign every line a unique number, starting at 1 for the first line, and incrementing by 1 for each successive line.In the C...
s. Also observe the unpredictable way the flow of execution jumps from one area to another. Real-world occurrences of spaghetti code are more complex and can add greatly to a program's maintenance costs.
10 i = 0
20 i = i + 1
30 PRINT i; " squared = "; i * i
40 IF i >= 10 THEN GOTO 60
50 GOTO 20
60 PRINT "Program Completed."
70 END
Here is the same code written in a structured programming
Structured programming
Structured programming is a programming paradigm aimed on improving the clarity, quality, and development time of a computer program by making extensive use of subroutines, block structures and for and while loops - in contrast to using simple tests and jumps such as the goto statement which could...
style:
FOR i = 1 TO 10
PRINT i; " squared = "; i * i
NEXT i
PRINT "Program Completed."
END
The program jumps from one area to another but this jumping is predictable and formal. This is because using for loop
For loop
In computer science a for loop is a programming language statement which allows code to be repeatedly executed. A for loop is classified as an iteration statement....
s and functions
Subroutine
In computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....
are standard ways of providing flow control
Control flow
In computer science, control flow refers to the order in which the individual statements, instructions, or function calls of an imperative or a declarative program are executed or evaluated....
whereas the goto statement encourages arbitrary flow control. Though this example is small, real world programs are composed of many lines of code and are difficult to maintain when written in a spaghetti code fashion.
Assembly and script languages
When using the many forms of assembly languageAssembly language
An assembly language is a low-level programming language for computers, microprocessors, microcontrollers, and other programmable devices. It implements a symbolic representation of the machine codes and other constants needed to program a given CPU architecture...
(and also the underlying machine code
Machine code
Machine code or machine language is a system of impartible instructions executed directly by a computer's central processing unit. Each instruction performs a very specific task, typically either an operation on a unit of data Machine code or machine language is a system of impartible instructions...
) the danger of writing spaghetti code is especially great.
This is because they are low-level programming language
Low-level programming language
In computer science, a low-level programming language is a programming language that provides little or no abstraction from a computer's instruction set architecture. Generally this refers to either machine code or assembly language...
s where equivalents for structured control flow statements such as for loop
For loop
In computer science a for loop is a programming language statement which allows code to be repeatedly executed. A for loop is classified as an iteration statement....
s and while loop
While loop
In most computer programming languages, a while loop is a control flow statement that allows code to be executed repeatedly based on a given boolean condition. The while loop can be thought of as a repeating if statement....
s exist, but are often poorly understood by inexperienced programmers.
Many scripting languages have the same deficiencies: this applies to the batch scripting language of DOS
DOS
DOS, short for "Disk Operating System", is an acronym for several closely related operating systems that dominated the IBM PC compatible market between 1981 and 1995, or until about 2000 if one includes the partially DOS-based Microsoft Windows versions 95, 98, and Millennium Edition.Related...
and DCL
DIGITAL Command Language
DCL, the DIGITAL Command Language, is the standard command languageadopted by most of the operating systems that were sold by the former Digital Equipment Corporation...
on VMS
OpenVMS
OpenVMS , previously known as VAX-11/VMS, VAX/VMS or VMS, is a computer server operating system that runs on VAX, Alpha and Itanium-based families of computers. Contrary to what its name suggests, OpenVMS is not open source software; however, the source listings are available for purchase...
.
Nonetheless, adopting the same discipline as in structured programming
Structured programming
Structured programming is a programming paradigm aimed on improving the clarity, quality, and development time of a computer program by making extensive use of subroutines, block structures and for and while loops - in contrast to using simple tests and jumps such as the goto statement which could...
can greatly improve the readability and maintainability of such code.
This may take the form of conventions limiting the use of
goto
to correspond to the standard structures, or use of a set of assembler macros for if
and loop
constructs.Most assembly languages also provide a function stack, and function
Subroutine
In computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....
call mechanisms
which can be used to gain the advantages of procedural programming
Procedural programming
Procedural programming can sometimes be used as a synonym for imperative programming , but can also refer to a programming paradigm, derived from structured programming, based upon the concept of the procedure call...
. Macros can again be used to support a standardized form of parameter passing, to avoid ad hoc global variable
Global variable
In computer programming, a global variable is a variable that is accessible in every scope . Interaction mechanisms with global variables are called global environment mechanisms...
s and the action at a distance
Action at a distance (computer science)
Action at a distance is an anti-pattern in which behavior in one part of a program varies wildly based on difficult or impossible to identify operations in another part of the program....
anti-pattern.
Programs written in higher-level languages
High-level programming language
A high-level programming language is a programming language with strong abstraction from the details of the computer. In comparison to low-level programming languages, it may use natural language elements, be easier to use, or be from the specification of the program, making the process of...
with high-level constructs such as for loops (as in the second example above) are often compiled into assembly or machine code. When this process occurs, the high-level constructs are translated into low-level "spaghetti code" which may resemble the first example above in terms of control flow. But because compilers must be faithful to high-level constructs in the source code, the problems that plague relatively unstructured languages like BASIC do not haunt higher-level languages. It does, however, mean that debugging even mildly optimized code with a source-level debugger can be surprisingly confusing.
Other related terms
The term "spaghetti code" has inspired the coinage of other terms that similarly compare program structure to styles of pastaPasta
Pasta is a staple food of traditional Italian cuisine, now of worldwide renown. It takes the form of unleavened dough, made in Italy, mostly of durum wheat , water and sometimes eggs. Pasta comes in a variety of different shapes that serve for both decoration and to act as a carrier for the...
.
Ravioli code
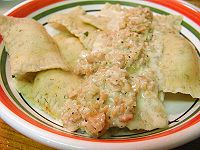
Loose coupling
In computing and systems design a loosely coupled system is one where each of its components has, or makes use of, little or no knowledge of the definitions of other separate components. The notion was introduced into organizational studies by Karl Weick...
software components. The term stems from the analogy of ravioli
Ravioli
Ravioli are a traditional type of Italian filled pasta. They are composed of a filling sealed between two layers of thin egg pasta dough and are served either in broth or with a pasta sauce. The word ravioli is reminiscent of the Italian verb riavvolgere , though the two words are not...
(small pasta pouches containing cheese, meat, or vegetables) to object
Object (computer science)
In computer science, an object is any entity that can be manipulated by the commands of a programming language, such as a value, variable, function, or data structure...
s (which ideally are encapsulated modules consisting of both code and data).
Lasagna code
Lasagna code is a type of program structure, characterized by several well-defined and separable layers, where each layer of code accesses services in the layers below through well-defined interfaceInterface (computer science)
In the field of computer science, an interface is a tool and concept that refers to a point of interaction between components, and is applicable at the level of both hardware and software...
s. The analogy stems from the layered structure of a plate of lasagna
Lasagna
Lasagna is a wide and flat type of pasta and possibly one of the oldest shapes. As with most other pasta shapes, the word is generally used in its plural form lasagne in Italy and the U.K. Traditionally, the dough was prepared in Southern Italy with semolina and water and in the northern regions,...
, where different ingredients (meat, sauce, vegetables, or cheese) are each separated by strips of pasta.
One common instance of lasagna code occurs at the interface between different subsystems, such as between web application code, business logic
Business logic
Business logic, or domain logic, is a non-technical term generally used to describe the functional algorithms that handle information exchange between a database and a user interface.- Scope of business logic :Business logic:...
, and a relational database
Relational database
A relational database is a database that conforms to relational model theory. The software used in a relational database is called a relational database management system . Colloquial use of the term "relational database" may refer to the RDBMS software, or the relational database itself...
. Another common programming technique, alternate hard and soft layers (use of different programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
s at different levels of the program architecture), tends to produce lasagna code. In general, client–server applications are frequently lasagna code, with well-defined interfaces between client and server.
Lasagna code generally enforces encapsulation between the different "layers", as the subsystems in question may have no means of communication other than through a well-defined mechanism, such as Structured Query Language, a foreign function interface
Foreign function interface
A foreign function interface is a mechanism by which a program written in one programming language can call routines or make use of services written in another. The term comes from the specification for Common Lisp, which explicitly refers to the language features for inter-language calls as...
, or Remote Procedure Call
Remote procedure call
In computer science, a remote procedure call is an inter-process communication that allows a computer program to cause a subroutine or procedure to execute in another address space without the programmer explicitly coding the details for this remote interaction...
. However, individual layers in the system may be highly unstructured or disorganized.
The term was coined by database guru
Guru
A guru is one who is regarded as having great knowledge, wisdom, and authority in a certain area, and who uses it to guide others . Other forms of manifestation of this principle can include parents, school teachers, non-human objects and even one's own intellectual discipline, if the...
Joe Celko
Joe Celko
Joe Celko is an American relational database expert from Austin, Texas. He has participated on the ANSI X3H2 Database Standards Committee, and helped write the SQL-89 and SQL-92 standards. He is the author of a Morgan-Kaufmann series of books on SQL, and over 1000 published articles on SQL and...
in 1982.
A similar layering may be seen in communication stacks, where a protocol (such as the OSI model
OSI model
The Open Systems Interconnection model is a product of the Open Systems Interconnection effort at the International Organization for Standardization. It is a prescription of characterizing and standardizing the functions of a communications system in terms of abstraction layers. Similar...
) is divided into layers (in this case 7), with each layer performing a limited and well defined function and communicating with other layers using specific and standardized methods. Such a design eases the evolutionary improvement of the entire stack though layer-specific improvements.
Spaghetti with meatballs
The term "spaghetti with meatballs" is a pejorative term used in computer science to describe loosely constructed object-oriented programmingObject-oriented programming
Object-oriented programming is a programming paradigm using "objects" – data structures consisting of data fields and methods together with their interactions – to design applications and computer programs. Programming techniques may include features such as data abstraction,...
(OOP) that remains dependent on procedural code. It may be the result of a system whose development has transitioned over a long life-cycle, language constraints, micro-optimization theatre, or a lack of coherent coding standards
Programming style
Programming style is a set of rules or guidelines used when writing the source code for a computer program. It is often claimed that following a particular programming style will help programmers to read and understand source code conforming to the style, and help to avoid introducing errors.A...
.
In some languages, OOP features are only available in later specifications. Notable examples of this include Visual Basic
Visual Basic
Visual Basic is the third-generation event-driven programming language and integrated development environment from Microsoft for its COM programming model...
, and PHP
PHP
PHP is a general-purpose server-side scripting language originally designed for web development to produce dynamic web pages. For this purpose, PHP code is embedded into the HTML source document and interpreted by a web server with a PHP processor module, which generates the web page document...
. Other languages, like C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
, rely on function pointers
Function pointer
A function pointer is a type of pointer in C, C++, D, and other C-like programming languages, and Fortran 2003. When dereferenced, a function pointer can be used to invoke a function and pass it arguments just like a normal function...
to simulate OOP — still requiring the underlying procedural code to which they point.
Using OOP does not necessarily prevent the class' code from becoming spaghetti-like. In this parlance, "spaghetti" describes twisted, tangled and unstructured code, while "meatballs" denotes the use of class structures (i.e. objects).
See also
- Structured programmingStructured programmingStructured programming is a programming paradigm aimed on improving the clarity, quality, and development time of a computer program by making extensive use of subroutines, block structures and for and while loops - in contrast to using simple tests and jumps such as the goto statement which could...
Coding withoutgoto
, e.g. using only loop, sequence and alternate structures. - International Obfuscated C Code ContestInternational Obfuscated C Code ContestThe International Obfuscated C Code Contest is a programming contest for the most creatively obfuscated C code. It was held annually between 1984 and 1996, and thereafter in 1998, 2000, 2001, 2004, 2005 and 2006....
A competition to produce pleasingly obscure C code.
External links
- Go To Statement Considered Harmful. The classic repudiation of spaghetti code by Edsger DijkstraEdsger DijkstraEdsger Wybe Dijkstra ; ) was a Dutch computer scientist. He received the 1972 Turing Award for fundamental contributions to developing programming languages, and was the Schlumberger Centennial Chair of Computer Sciences at The University of Texas at Austin from 1984 until 2000.Shortly before his...
. - We don't know where to GOTO if we don't know where we've COME FROM by R. Lawrence Clark from DATAMATION, December, 1973
- Refactoring Java spaghetti code into Java bento code separating out a bowl full of code from one class into seven classes
- Objects and Frameworks - Taking a Step Back by Brian Rinaldi