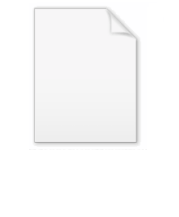
Concurrent computing
Encyclopedia
Concurrent computing is a form of computing
in which programs
are designed as collections of interacting computational processes that may be executed in parallel
. Concurrent
programs can be executed sequentially on a single processor
by interleaving the execution steps of each computational process, or executed in parallel by assigning each computational process to one of a set of processors that may be close
or distributed
across a network. The main challenges in designing concurrent programs are ensuring the correct sequencing of the interactions or communications between different computational processes, and coordinating access to resources that are shared among processes. A number of different methods can be used to implement concurrent programs, such as implementing each computational process as an operating system process, or implementing the computational processes as a set of threads
within a single operating system process.
Pioneers in the field of concurrent computing include Edsger Dijkstra
, Per Brinch Hansen
, and C.A.R. Hoare.
), while in others it must be handled explicitly. Explicit communication can be divided into two classes:
Shared memory
communication : Concurrent components communicate by altering the contents of shared memory locations (exemplified by Java
and C#). This style of concurrent programming usually requires the application of some form of locking (e.g., mutexes
, semaphores
, or monitors
) to coordinate between threads.
Message passing
communication : Concurrent components communicate by exchanging messages (exemplified by Scala
, Erlang
and occam). The exchange of messages may be carried out asynchronously, or may use a rendezvous style in which the sender blocks until the message is received. Asynchronous message passing may be reliable or unreliable (sometimes referred to as "send and pray"). Message-passing concurrency tends to be far easier to reason about than shared-memory concurrency, and is typically considered a more robust form of concurrent programming. A wide variety of mathematical theories for understanding and analyzing message-passing systems are available, including the Actor model
, and various process calculi. Message passing can be efficiently implemented on symmetric multiprocessors
, with or without shared coherent memory
.
Shared memory and message passing concurrency have different performance characteristics; typically (although not always), the per-process memory overhead and task switching overhead is lower in a message passing system, but the overhead of message passing itself is greater than for a procedure call. These differences are often overwhelmed by other performance factors.
Suppose
, or non-blocking algorithms.
Because concurrent systems rely on the use of shared resources (including communication media), concurrent computing in general requires the use of some form of arbiter
somewhere in the implementation to mediate access to these resources.
Unfortunately, while many solutions exist to the problem of a conflict over one resource, many of those "solutions" have their own concurrency problems such as deadlock
when more than one resource is involved.
, support for distributed computing
, message passing, shared resources
(including shared memory
) or futures
(known also as promises). Such languages are sometimes described as Concurrency Oriented Languages or Concurrency Oriented Programming Languages (COPL).
Today, the most commonly used programming languages that have specific constructs for concurrency are Java
and C#. Both of these languages fundamentally use a shared-memory concurrency model, with locking provided by monitors
(although message-passing models can and have been implemented on top of the underlying shared-memory model). Of the languages that use a message-passing concurrency model, Erlang
is probably the most widely used in industry at present.
Many concurrent programming languages have been developed more as research languages (e.g. Pict
) rather than as languages for production use. However, languages such as Erlang
, Limbo
, and occam have seen industrial use at various times in the last 20 years. Languages in which concurrency plays an important role include:
Many other languages provide support for concurrency in the form of libraries (on level roughly comparable with the above list).
Computing
Computing is usually defined as the activity of using and improving computer hardware and software. It is the computer-specific part of information technology...
in which programs
Computer program
A computer program is a sequence of instructions written to perform a specified task with a computer. A computer requires programs to function, typically executing the program's instructions in a central processor. The program has an executable form that the computer can use directly to execute...
are designed as collections of interacting computational processes that may be executed in parallel
Parallel computing
Parallel computing is a form of computation in which many calculations are carried out simultaneously, operating on the principle that large problems can often be divided into smaller ones, which are then solved concurrently . There are several different forms of parallel computing: bit-level,...
. Concurrent
Concurrency (computer science)
In computer science, concurrency is a property of systems in which several computations are executing simultaneously, and potentially interacting with each other...
programs can be executed sequentially on a single processor
Central processing unit
The central processing unit is the portion of a computer system that carries out the instructions of a computer program, to perform the basic arithmetical, logical, and input/output operations of the system. The CPU plays a role somewhat analogous to the brain in the computer. The term has been in...
by interleaving the execution steps of each computational process, or executed in parallel by assigning each computational process to one of a set of processors that may be close
Multiprocessing
Multiprocessing is the use of two or more central processing units within a single computer system. The term also refers to the ability of a system to support more than one processor and/or the ability to allocate tasks between them...
or distributed
Distributed computing
Distributed computing is a field of computer science that studies distributed systems. A distributed system consists of multiple autonomous computers that communicate through a computer network. The computers interact with each other in order to achieve a common goal...
across a network. The main challenges in designing concurrent programs are ensuring the correct sequencing of the interactions or communications between different computational processes, and coordinating access to resources that are shared among processes. A number of different methods can be used to implement concurrent programs, such as implementing each computational process as an operating system process, or implementing the computational processes as a set of threads
Thread (computer science)
In computer science, a thread of execution is the smallest unit of processing that can be scheduled by an operating system. The implementation of threads and processes differs from one operating system to another, but in most cases, a thread is contained inside a process...
within a single operating system process.
Pioneers in the field of concurrent computing include Edsger Dijkstra
Edsger Dijkstra
Edsger Wybe Dijkstra ; ) was a Dutch computer scientist. He received the 1972 Turing Award for fundamental contributions to developing programming languages, and was the Schlumberger Centennial Chair of Computer Sciences at The University of Texas at Austin from 1984 until 2000.Shortly before his...
, Per Brinch Hansen
Per Brinch Hansen
Per Brinch Hansen was a Danish-American computer scientist known for concurrent programming theory.-Biography:He was born in Frederiksberg, in Copenhagen, Denmark....
, and C.A.R. Hoare.
Concurrent interaction and communication
In some concurrent computing systems, communication between the concurrent components is hidden from the programmer (e.g., by using futuresFuture (programming)
In computer science, future, promise, and delay refer to constructs used for synchronization in some concurrent programming languages. They describe an object that acts as a proxy for a result that is initially not known, usually because the computation of its value has not yet completed.The term...
), while in others it must be handled explicitly. Explicit communication can be divided into two classes:
Shared memory
Shared memory
In computing, shared memory is memory that may be simultaneously accessed by multiple programs with an intent to provide communication among them or avoid redundant copies. Depending on context, programs may run on a single processor or on multiple separate processors...
communication : Concurrent components communicate by altering the contents of shared memory locations (exemplified by Java
Java (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
and C#). This style of concurrent programming usually requires the application of some form of locking (e.g., mutexes
Mutual exclusion
Mutual exclusion algorithms are used in concurrent programming to avoid the simultaneous use of a common resource, such as a global variable, by pieces of computer code called critical sections. A critical section is a piece of code in which a process or thread accesses a common resource...
, semaphores
Semaphore (programming)
In computer science, a semaphore is a variable or abstract data type that provides a simple but useful abstraction for controlling access by multiple processes to a common resource in a parallel programming environment....
, or monitors
Monitor (synchronization)
In concurrent programming, a monitor is an object or module intended to be used safely by more than one thread. The defining characteristic of a monitor is that its methods are executed with mutual exclusion. That is, at each point in time, at most one thread may be executing any of its methods...
) to coordinate between threads.
Message passing
Message passing
Message passing in computer science is a form of communication used in parallel computing, object-oriented programming, and interprocess communication. In this model, processes or objects can send and receive messages to other processes...
communication : Concurrent components communicate by exchanging messages (exemplified by Scala
Scala programming language
Scala is a multi-paradigm programming language designed to integrate features of object-oriented programming and functional programming. The name Scala is a portmanteau of "scalable" and "language", signifying that it is designed to grow with the demands of its users...
, Erlang
Erlang programming language
Erlang is a general-purpose concurrent, garbage-collected programming language and runtime system. The sequential subset of Erlang is a functional language, with strict evaluation, single assignment, and dynamic typing. For concurrency it follows the Actor model. It was designed by Ericsson to...
and occam). The exchange of messages may be carried out asynchronously, or may use a rendezvous style in which the sender blocks until the message is received. Asynchronous message passing may be reliable or unreliable (sometimes referred to as "send and pray"). Message-passing concurrency tends to be far easier to reason about than shared-memory concurrency, and is typically considered a more robust form of concurrent programming. A wide variety of mathematical theories for understanding and analyzing message-passing systems are available, including the Actor model
Actor model
In computer science, the Actor model is a mathematical model of concurrent computation that treats "actors" as the universal primitives of concurrent digital computation: in response to a message that it receives, an actor can make local decisions, create more actors, send more messages, and...
, and various process calculi. Message passing can be efficiently implemented on symmetric multiprocessors
Symmetric multiprocessing
In computing, symmetric multiprocessing involves a multiprocessor computer hardware architecture where two or more identical processors are connected to a single shared main memory and are controlled by a single OS instance. Most common multiprocessor systems today use an SMP architecture...
, with or without shared coherent memory
Cache coherency
In computing, cache coherence refers to the consistency of data stored in local caches of a shared resource.When clients in a system maintain caches of a common memory resource, problems may arise with inconsistent data. This is particularly true of CPUs in a multiprocessing system...
.
Shared memory and message passing concurrency have different performance characteristics; typically (although not always), the per-process memory overhead and task switching overhead is lower in a message passing system, but the overhead of message passing itself is greater than for a procedure call. These differences are often overwhelmed by other performance factors.
Coordinating access to resources
One of the major issues in concurrent computing is preventing concurrent processes from interfering with each other. For example, consider the following algorithm for making withdrawals from a checking account represented by the shared resourcebalance
:
1 bool withdraw( int withdrawal )
2 {
3 if ( balance >= withdrawal )
4 {
5 balance -= withdrawal;
6 return true;
7 }
8 return false;
9 }
Suppose
balance=500
, and two concurrent processes make the calls withdraw(300)
and withdraw(350)
. If line 3 in both operations executes before line 5 both operations will find that balance > withdrawal
evaluates to true
, and execution will proceed to subtracting the withdrawal amount. However, since both processes perform their withdrawals, the total amount withdrawn will end up being more than the original balance. These sorts of problems with shared resources require the use of concurrency controlConcurrency control
In information technology and computer science, especially in the fields of computer programming , operating systems , multiprocessors, and databases, concurrency control ensures that correct results for concurrent operations are generated, while getting those results as quickly as possible.Computer...
, or non-blocking algorithms.
Because concurrent systems rely on the use of shared resources (including communication media), concurrent computing in general requires the use of some form of arbiter
Metastability in electronics
Metastability in electronics is the ability of a digital electronic system to persist for an unbounded time in an unstable equilibrium or metastable state....
somewhere in the implementation to mediate access to these resources.
Unfortunately, while many solutions exist to the problem of a conflict over one resource, many of those "solutions" have their own concurrency problems such as deadlock
Deadlock
A deadlock is a situation where in two or more competing actions are each waiting for the other to finish, and thus neither ever does. It is often seen in a paradox like the "chicken or the egg"...
when more than one resource is involved.
Advantages
- Increased application throughput – parallel execution of a concurrent program allows the number of tasks completed in certain time period to increase.
- High responsiveness for input/output – input/output-intensive applications mostly wait for input or output operations to complete. Concurrent programming allows the time that would be spent waiting to be used for another task.
- More appropriate program structure – some problems and problem domains are well-suited to representation as concurrent tasks or processes.
Concurrent programming languages
Concurrent programming languages are programming languages that use language constructs for concurrency. These constructs may involve multi-threadingThread (computer science)
In computer science, a thread of execution is the smallest unit of processing that can be scheduled by an operating system. The implementation of threads and processes differs from one operating system to another, but in most cases, a thread is contained inside a process...
, support for distributed computing
Distributed computing
Distributed computing is a field of computer science that studies distributed systems. A distributed system consists of multiple autonomous computers that communicate through a computer network. The computers interact with each other in order to achieve a common goal...
, message passing, shared resources
Sharing
Sharing the joint use of a resource or space. In its narrow sense, it refers to joint or alternating use of an inherently finite good, such as a common pasture or a shared residence. It is also the process of dividing and distributing. Apart from obvious instances, which we can observe in human...
(including shared memory
Parallel Random Access Machine
In computer science, Parallel Random Access Machine is a shared memory abstract machine. As its name indicates, the PRAM was intended as the parallel computing analogy to the random access machine...
) or futures
Future (programming)
In computer science, future, promise, and delay refer to constructs used for synchronization in some concurrent programming languages. They describe an object that acts as a proxy for a result that is initially not known, usually because the computation of its value has not yet completed.The term...
(known also as promises). Such languages are sometimes described as Concurrency Oriented Languages or Concurrency Oriented Programming Languages (COPL).
Today, the most commonly used programming languages that have specific constructs for concurrency are Java
Java (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
and C#. Both of these languages fundamentally use a shared-memory concurrency model, with locking provided by monitors
Monitor (synchronization)
In concurrent programming, a monitor is an object or module intended to be used safely by more than one thread. The defining characteristic of a monitor is that its methods are executed with mutual exclusion. That is, at each point in time, at most one thread may be executing any of its methods...
(although message-passing models can and have been implemented on top of the underlying shared-memory model). Of the languages that use a message-passing concurrency model, Erlang
Erlang programming language
Erlang is a general-purpose concurrent, garbage-collected programming language and runtime system. The sequential subset of Erlang is a functional language, with strict evaluation, single assignment, and dynamic typing. For concurrency it follows the Actor model. It was designed by Ericsson to...
is probably the most widely used in industry at present.
Many concurrent programming languages have been developed more as research languages (e.g. Pict
Pict programming language
Pict is a statically typed programming language, one of the very few based on the Π-calculus. Work on the language began at the University of Edinburgh in 1992. The language is still at an experimental stage.-Sources:*-External links:...
) rather than as languages for production use. However, languages such as Erlang
Erlang programming language
Erlang is a general-purpose concurrent, garbage-collected programming language and runtime system. The sequential subset of Erlang is a functional language, with strict evaluation, single assignment, and dynamic typing. For concurrency it follows the Actor model. It was designed by Ericsson to...
, Limbo
Limbo programming language
Limbo is a programming language for writing distributed systems and is the language used to write applications for the Inferno operating system...
, and occam have seen industrial use at various times in the last 20 years. Languages in which concurrency plays an important role include:
- ActorScript – theoretical purely actor-based language defined in terms of itself
- Ada
- Afnix – concurrent access to data is protected automatically (previously called Aleph, but unrelated to Alef)
- AlefAlef (programming language)The Alef programming language was designed as part of the Plan 9 operating system by Phil Winterbottom of Bell Labs.In a February 2000 slideshow, Rob Pike noted: "…although Alef was a fruitful language, it proved too difficult to maintain a variant language across multiple architectures, so we took...
– concurrent language with threads and message passing, used for systems programming in early versions of Plan 9 from Bell LabsPlan 9 from Bell LabsPlan 9 from Bell Labs is a distributed operating system. It was developed primarily for research purposes as the successor to Unix by the Computing Sciences Research Center at Bell Labs between the mid-1980s and 2002... - AliceAlice (programming language)Alice ML is a functional programming language designed by the at Saarland University. It is a dialect of Standard ML, augmented with support for lazy evaluation, concurrency and constraint programming.-Overview:Alice extends Standard ML in a number of ways that distinguish it from its predecessor...
– extension to Standard MLStandard MLStandard ML is a general-purpose, modular, functional programming language with compile-time type checking and type inference. It is popular among compiler writers and programming language researchers, as well as in the development of theorem provers.SML is a modern descendant of the ML...
, adds support for concurrency via futures. - Ateji PXAteji PXAteji PX is an object-oriented programming language extension for Java. It is intended to facilliate parallel computing on multi-core processors, GPU, Grid and Cloud....
– an extension to JavaJava (programming language)Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
with parallel primitives inspired from pi-calculusPi-calculusIn theoretical computer science, the π-calculus is a process calculus originally developed by Robin Milner, and David Walker as a continuation of work on the process calculus CCS... - AxumAxum (programming language)Axum is a domain specific concurrent programming language, based on the Actor model, being developed by Microsoft...
– domain specific concurrent programming language, based on the Actor model and on the .NET Common Language Runtime using a C-like syntax. - Chapel – a parallel programming language being developed by Cray Inc.
- Charm++Charm++Charm++ is a parallel object-oriented programming language based on C++ and developed in the Parallel Programming Laboratory at the University of Illinois. Charm++ is designed with the goal of enhancing programmer productivity by providing a high-level abstraction of a parallel program while at the...
– C++C++C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
-like language for thousands of processors. - CilkCilkCilk is a general-purpose programming language designed for multithreaded parallel computing. The commercial instantiation is Intel Cilk Plus.-Design:...
– a concurrent CC (programming language)C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system.... - Cω – C Omega, a research language extending C#, uses asynchronous communication
- ClojureClojureClojure |closure]]") is a recent dialect of the Lisp programming language created by Rich Hickey. It is a general-purpose language supporting interactive development that encourages a functional programming style, and simplifies multithreaded programming....
– a modern Lisp targeting the JVM - Concurrent Clean – a functional programming language, similar to HaskellHaskell (programming language)Haskell is a standardized, general-purpose purely functional programming language, with non-strict semantics and strong static typing. It is named after logician Haskell Curry. In Haskell, "a function is a first-class citizen" of the programming language. As a functional programming language, the...
- Concurrent HaskellConcurrent HaskellConcurrent Haskell extends Haskell 98 with explicit concurrency. The two main concepts underpinning Concurrent Haskell are:* A primitive type MVar α implementing a bounded/single-place asynchronous channel, which is either empty or holds a value of type α....
– lazy, pure functional language operating concurrent processes on shared memory - Concurrent MLConcurrent MLConcurrent ML is a concurrent extension of the Standard ML programming language.-Sample Code:Here is sample code to print "hello, world" to the console. It spawns a thread which creates a channel for strings. This thread then spawns another thread which prints the first string that is received...
– a concurrent extension of Standard MLStandard MLStandard ML is a general-purpose, modular, functional programming language with compile-time type checking and type inference. It is popular among compiler writers and programming language researchers, as well as in the development of theorem provers.SML is a modern descendant of the ML... - Concurrent PascalConcurrent PascalConcurrent Pascal was designed by Per Brinch Hansen for writing concurrent computing programs such asoperating systems and real-time monitoring systems on shared memorycomputers....
– by Per Brinch HansenPer Brinch HansenPer Brinch Hansen was a Danish-American computer scientist known for concurrent programming theory.-Biography:He was born in Frederiksberg, in Copenhagen, Denmark.... - CurryCurry programming languageCurry is an experimental functional logic programming language, based on the Haskell language. It merges elements of functional and logic programming, including constraint programming integration....
- E – uses promises, ensures deadlocks cannot occur
- EiffelEiffel (programming language)Eiffel is an ISO-standardized, object-oriented programming language designed by Bertrand Meyer and Eiffel Software. The design of the language is closely connected with the Eiffel programming method...
– through its SCOOPSCOOP (software)SCOOP stands for Simple Concurrent Object Oriented Programming. It is a concurrency model designed for the Eiffel programming language, conceived by Eiffel's creator and designer, Bertrand Meyer....
mechanism based on the concepts of Design by Contract - ErlangErlang programming languageErlang is a general-purpose concurrent, garbage-collected programming language and runtime system. The sequential subset of Erlang is a functional language, with strict evaluation, single assignment, and dynamic typing. For concurrency it follows the Actor model. It was designed by Ericsson to...
– uses asynchronous message passing with nothing shared - FaustFAUST (programming language)FAUST, that stands for Functional AUdio STream, is a programming language that provides a purely functional approach to signal processing while offering a high level of performance...
– Realtime functional programming language for signal processing. The Faust compiler provides automatic parallelization using either OpenMPOpenMPOpenMP is an API that supports multi-platform shared memory multiprocessing programming in C, C++, and Fortran, on most processor architectures and operating systems, including Linux, Unix, AIX, Solaris, Mac OS X, and Microsoft Windows platforms...
or a specific work-stealing scheduler. - FortranFortranFortran is a general-purpose, procedural, imperative programming language that is especially suited to numeric computation and scientific computing...
– CoarraysCo-array FortranCo-array Fortran , formerly known as F--, is an extension of Fortran 95/2003 for parallel processing created by Robert Numrich and John Reid in 1990s...
are part of Fortran 2008 standard - GoGo (programming language)Go is a compiled, garbage-collected, concurrent programming language developed by Google Inc.The initial design of Go was started in September 2007 by Robert Griesemer, Rob Pike, and Ken Thompson. Go was officially announced in November 2009. In May 2010, Rob Pike publicly stated that Go was being...
– systems programming language with explicit support for concurrent programming - IoIo (programming language)Io is a pure object-oriented programming language inspired by Smalltalk, Self, Lua, Lisp, Act1, and NewtonScript. Io has a prototype-based object model similar to the ones in Self and NewtonScript, eliminating the distinction between instance and class. Like Smalltalk, everything is an object and...
– actor-based concurrency - Janus features distinct "askers" and "tellers" to logical variables, bag channels; is purely declarative
- JoCamlJoCamlJoCaml is an experimental functional programming language derived from OCaml. It integrates the primitives of the join-calculus to enable flexible, type-checked concurrent and distributed programming.- External links :* *...
- Join JavaJoin JavaJoin Java is a programming language that extends the standard Java programming language with the join semantics of the join-calculus. It was written at the University of South Australia within the Reconfigurable Computing Lab by Dr...
– concurrent language based on the Java programming languageJava (programming language)Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities... - Joule – dataflow language, communicates by message passing
- JoyceJoyce (programming language)Joyce is a secure, concurrent programming language designed by Per Brinch Hansen in the 1980s. It is based on the sequential language Pascal and the principles of Communicating Sequential Processes...
– a concurrent teaching language built on Concurrent PascalConcurrent PascalConcurrent Pascal was designed by Per Brinch Hansen for writing concurrent computing programs such asoperating systems and real-time monitoring systems on shared memorycomputers....
with features from CSPCommunicating sequential processesIn computer science, Communicating Sequential Processes is a formal language for describing patterns of interaction in concurrent systems. It is a member of the family of mathematical theories of concurrency known as process algebras, or process calculi...
by Per Brinch HansenPer Brinch HansenPer Brinch Hansen was a Danish-American computer scientist known for concurrent programming theory.-Biography:He was born in Frederiksberg, in Copenhagen, Denmark.... - LabVIEWLabVIEWLabVIEW is a system design platform and development environment for a visual programming language from National Instruments. LabVIEW provides engineers and scientists with the tools needed to create and deploy measurement and control systems.The graphical language is named "G"...
– graphical, dataflow programming language, in which functions are nodes in a graph and data is wires between those nodes. Includes object oriented language extensions. - LimboLimbo programming languageLimbo is a programming language for writing distributed systems and is the language used to write applications for the Inferno operating system...
– relative of AlefAlef (programming language)The Alef programming language was designed as part of the Plan 9 operating system by Phil Winterbottom of Bell Labs.In a February 2000 slideshow, Rob Pike noted: "…although Alef was a fruitful language, it proved too difficult to maintain a variant language across multiple architectures, so we took...
, used for systems programming in Inferno (operating system)Inferno (operating system)Inferno is a distributed operating system started at Bell Labs, but is now developed and maintained by Vita Nuova Holdings as free software. Inferno was based on the experience gained with Plan 9 from Bell Labs, and the further research of Bell Labs into operating systems, languages, on-the-fly... - MultiLispMultiLispMultiLisp is a functional programming language and dialect of the Lisp dialect Scheme, extended with constructs for parallel execution and shared memory; MultiLisp is implemented in Interlisp. These extensions involve side effects, rendering MultiLisp non-deterministic...
– Scheme variant extended to support parallelism - Modula-3Modula-3In computer science, Modula-3 is a programming language conceived as a successor to an upgraded version of Modula-2 known as Modula-2+. While it has been influential in research circles it has not been adopted widely in industry...
– modern language in Algol family with extensive support for threads, mutexes, condition variables. - NewsqueakNewsqueakNewsqueak is a concurrent programming language for writing application software for windowing systems. It was designed at Bell Labs by Rob Pike in the late 1980s....
– research language with channels as first-class values; predecessor of AlefAlef (programming language)The Alef programming language was designed as part of the Plan 9 operating system by Phil Winterbottom of Bell Labs.In a February 2000 slideshow, Rob Pike noted: "…although Alef was a fruitful language, it proved too difficult to maintain a variant language across multiple architectures, so we took... - occam – influenced heavily by Communicating Sequential ProcessesCommunicating sequential processesIn computer science, Communicating Sequential Processes is a formal language for describing patterns of interaction in concurrent systems. It is a member of the family of mathematical theories of concurrency known as process algebras, or process calculi...
(CSP).- occam-π – a modern variant of occam, which incorporates ideas from Milner's π-calculusPi-calculusIn theoretical computer science, the π-calculus is a process calculus originally developed by Robin Milner, and David Walker as a continuation of work on the process calculus CCS...
- occam-π – a modern variant of occam, which incorporates ideas from Milner's π-calculus
- Orc – a heavily concurrent, nondeterministic language based on Kleene algebraKleene algebraIn mathematics, a Kleene algebra is either of two different things:* A bounded distributive lattice with an involution satisfying De Morgan's laws , additionally satisfying the inequality x∧−x ≤ y∨−y. Kleene algebras are subclasses of Ockham algebras...
. - Oz – multiparadigm language, supports shared-state and message-passing concurrency, and futures
- Mozart Programming SystemMozart Programming SystemThe Mozart Programming System is a multiplatform implementation of the Oz programming language developed by an international group, the Mozart Consortium, which originally consisted of Saarland University, the Swedish Institute of Computer Science, and the Université catholique de Louvain.Mozart...
– multiplatform Oz
- Mozart Programming System
- PictPict programming languagePict is a statically typed programming language, one of the very few based on the Π-calculus. Work on the language began at the University of Edinburgh in 1992. The language is still at an experimental stage.-Sources:*-External links:...
– essentially an executable implementation of Milner's π-calculusPi-calculusIn theoretical computer science, the π-calculus is a process calculus originally developed by Robin Milner, and David Walker as a continuation of work on the process calculus CCS... - Perl with AnyEvent and Coro
- Python with greenlet and gevent.
- ReiaReia (programming language)Reia is a general-purpose concurrent object-oriented programming language for the Erlang virtual machine.Reia supports multiple programming paradigms including imperative, functional, declarative, object oriented, and concurrent. It uses the actor model for concurrency in a manner that works...
– uses asynchronous message passing between shared-nothing objects - SALSASALSA programming languageThe SALSA programming language is an actor-oriented programming language that uses concurrency primitives beyond asynchronous message passing, including token-passing, join, and first-class continuations...
– actor language with token-passing, join, and first-class continuations for distributed computing over the Internet - Scala – a general purpose programming language designed to express common programming patterns in a concise, elegant, and type-safe way
- SR – research language
- Stackless PythonStackless PythonStackless Python, or Stackless, is a Python programming language interpreter, so named because it avoids depending on the C call stack for its own stack. The most prominent feature of Stackless is microthreads, which avoid much of the overhead associated with usual operating system threads...
- StratifiedJS – a combinator-based concurrency language based on JavaScriptJavaScriptJavaScript is a prototype-based scripting language that is dynamic, weakly typed and has first-class functions. It is a multi-paradigm language, supporting object-oriented, imperative, and functional programming styles....
- SuperPascalSuperPascalSuper Pascal is an imperative, concurrent computing programming language developed by Brinch Hansen. It was designed as a publication language: a thinking tool to enable the clear and concise expression of concepts in parallel programming. This is in contrast with implementation languages which are...
– a concurrent teaching language built on Concurrent PascalConcurrent PascalConcurrent Pascal was designed by Per Brinch Hansen for writing concurrent computing programs such asoperating systems and real-time monitoring systems on shared memorycomputers....
and JoyceJoyce (programming language)Joyce is a secure, concurrent programming language designed by Per Brinch Hansen in the 1980s. It is based on the sequential language Pascal and the principles of Communicating Sequential Processes...
by Per Brinch HansenPer Brinch HansenPer Brinch Hansen was a Danish-American computer scientist known for concurrent programming theory.-Biography:He was born in Frederiksberg, in Copenhagen, Denmark.... - UniconUnicon programming languageUnicon is a programming language designed by American computer scientist Clint Jeffery. Unicon descended from Icon and its preprocessor, IDOL, that offers better access to the operating system as well as support for object-oriented programming...
– Research language. - Termite Scheme adds Erlang-like concurrency to Scheme
- TNSDLTNSDLTNSDL stands for TeleNokia Specification and Description Language. TNSDL is based on ITU-T SDL-88 language. It is used exclusively at Nokia Siemens Networks for developing applications for telephone exchanges.-Purpose:...
– a language used at developing telecommunication exchanges, uses asynchronous message passing - VHDL – VHSIC Hardware Description Language, aka IEEE STD-1076
- XCXC Programming LanguageThe XC Programming Language is a computer programming language developed by XMOS.XC is an imperative programming language with a computational framework based on C. XC programs consist of functions that execute statements that act upon values stored in variables...
– a concurrency-extended subset of the C programming language developed by XMOSXMOSXMOS is a fabless semiconductor company that develops multi-core multi-threaded processors designed to execute several real-time tasks, DSP, and control flow all at once.-Company history:...
based on Communicating Sequential ProcessesCommunicating sequential processesIn computer science, Communicating Sequential Processes is a formal language for describing patterns of interaction in concurrent systems. It is a member of the family of mathematical theories of concurrency known as process algebras, or process calculi...
. The language also offers built-in constructs for programmable I/O.
Many other languages provide support for concurrency in the form of libraries (on level roughly comparable with the above list).
Models of concurrency
There are several models of concurrent computing, which can be used to understand and analyze concurrent systems. These models include:- Actor modelActor modelIn computer science, the Actor model is a mathematical model of concurrent computation that treats "actors" as the universal primitives of concurrent digital computation: in response to a message that it receives, an actor can make local decisions, create more actors, send more messages, and...
- Object-capability modelObject-capability modelThe object-capability model is a computer security model based on the Actor model of computation. The name "object-capability model" is due to the idea that the capability to perform an operation can be obtained by the following combination:...
for security
- Object-capability model
- Petri netPetri netA Petri net is one of several mathematical modeling languages for the description of distributed systems. A Petri net is a directed bipartite graph, in which the nodes represent transitions and places...
s - Process calculiProcess calculusIn computer science, the process calculi are a diverse family of related approaches for formally modelling concurrent systems. Process calculi provide a tool for the high-level description of interactions, communications, and synchronizations between a collection of independent agents or processes...
such as- Ambient calculusAmbient calculusIn computer science, the ambient calculus is a process calculus devised by Luca Cardelli and Andrew D. Gordon in 1998, and used to describe and theorise about concurrent systems that include mobility...
- Calculus of Communicating SystemsCalculus of Communicating SystemsThe Calculus of Communicating Systems is a process calculus introduced by Robin Milner around 1980 and the title of a book describing the calculus. Its actions model indivisible communications between exactly two participants. The formal language includes primitives for describing parallel...
(CCS) - Communicating Sequential ProcessesCommunicating sequential processesIn computer science, Communicating Sequential Processes is a formal language for describing patterns of interaction in concurrent systems. It is a member of the family of mathematical theories of concurrency known as process algebras, or process calculi...
(CSP) - π-calculus
- Ambient calculus
See also
- List of important publications in concurrent, parallel, and distributed computing
- Ptolemy Project
- Race condition
- Critical sectionCritical sectionIn concurrent programming a critical section is a piece of code that accesses a shared resource that must not be concurrently accessed by more than one thread of execution. A critical section will usually terminate in fixed time, and a thread, task or process will have to wait a fixed time to...
- Transaction processingTransaction processingIn computer science, transaction processing is information processing that is divided into individual, indivisible operations, called transactions. Each transaction must succeed or fail as a complete unit; it cannot remain in an intermediate state...
- Chu spaceChu spaceChu spaces generalize the notion of topological space by dropping the requirements that the set of open sets be closed under union and finite intersection, that the open sets be extensional, and that the membership predicate be two-valued...
- Sheaf (mathematics)Sheaf (mathematics)In mathematics, a sheaf is a tool for systematically tracking locally defined data attached to the open sets of a topological space. The data can be restricted to smaller open sets, and the data assigned to an open set is equivalent to all collections of compatible data assigned to collections of...
- Software transactional memorySoftware transactional memoryIn computer science, software transactional memory is a concurrency control mechanism analogous to database transactions for controlling access to shared memory in concurrent computing. It is an alternative to lock-based synchronization. A transaction in this context is a piece of code that...
- Flow-based programmingFlow-based programmingIn computer science, flow-based programming is a programming paradigm that defines applications as networks of "black box" processes, which exchange data across predefined connections by message passing, where the connections are specified externally to the processes...