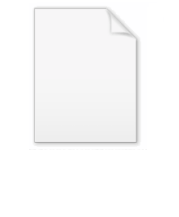
Longitudinal redundancy check
Encyclopedia
In telecommunication, a longitudinal redundancy check (LRC) or horizontal redundancy check is a form of redundancy check that is applied independently to each of a parallel group of bit streams. The data must be divided into transmission block
s, to which the additional check data is added.
The term usually applies to a single parity bit
per bit stream, although it could also be used to refer to a larger Hamming code
. While simple longitudinal parity
can only detect errors, it can be combined with additional error control coding, such as a transverse redundancy check
, to correct errors.
Telecom standard ISO 1155 states that a longitudinal redundancy check for a sequence of bytes may be computed in software by the following algorithm:
Set LRC = 0
For each byte b in the buffer
do
Set LRC = (LRC + b) AND 0xFF
end do
Set LRC = (((LRC XOR
0xFF) + 1) AND 0xFF)
An 8-bit LRC such as this is equivalent to a cyclic redundancy check
using the polynomial x8+1, but the independence of the bit streams is less clear when looked at that way.
Many protocols use such an XOR-based longitudinal redundancy check byte (often called Block Check Character or BCC), including the IEC 62056
-21 standard for electrical meter reading, smart cards as defined in ISO 7816
, and the ACCESS.bus
protocol.
/// Longitudinal Redundancy Check (LRC) calculator for a byte array. Warning: This code has no warranty. Check it, and fix it before using.
///
public static byte calculateLRC(byte[] bytes)
{
/* https://gist.github.com/953550
* http://en.wikipedia.org/wiki/Longitudinal_redundancy_check
*/
byte LRC = (byte)0;
for (int i = 0; i < bytes.Length; i++)
{
LRC ^= bytes[i];
}
return LRC;
}
* Calculates the checksum in compliance with the ISO 1155 standard
* More info: http://en.wikipedia.org/wiki/Longitudinal_redundancy_check
* @param data array to calculate checksum for
* @return returns the calculated checksum in byte format
*/
public byte calculateLRC(byte[] data) {
byte checksum = 0;
for (int i = 0; i <= data.length - 1; i++) {
checksum = (byte) ((checksum + data[i]) & 0xFF);
}
checksum = (byte) (((checksum ^ 0xFF) + 1) & 0xFF);
return checksum;
}
Transmission block
In telecommunication, the term transmission block has the following meanings:#A group of bits or characters transmitted as a unit and usually containing an encoding procedure for error control purposes....
s, to which the additional check data is added.
The term usually applies to a single parity bit
Parity bit
A parity bit is a bit that is added to ensure that the number of bits with the value one in a set of bits is even or odd. Parity bits are used as the simplest form of error detecting code....
per bit stream, although it could also be used to refer to a larger Hamming code
Hamming code
In telecommunication, Hamming codes are a family of linear error-correcting codes that generalize the Hamming-code invented by Richard Hamming in 1950. Hamming codes can detect up to two and correct up to one bit errors. By contrast, the simple parity code cannot correct errors, and can detect only...
. While simple longitudinal parity
Parity bit
A parity bit is a bit that is added to ensure that the number of bits with the value one in a set of bits is even or odd. Parity bits are used as the simplest form of error detecting code....
can only detect errors, it can be combined with additional error control coding, such as a transverse redundancy check
Transverse redundancy check
In telecommunications, a transverse redundancy check or vertical redundancy check is a redundancy check for synchronized parallel bits applied once per bit time, across the bit streams...
, to correct errors.
Telecom standard ISO 1155 states that a longitudinal redundancy check for a sequence of bytes may be computed in software by the following algorithm:
Set LRC = 0
For each byte b in the buffer
do
Set LRC = (LRC + b) AND 0xFF
end do
Set LRC = (((LRC XOR
Exclusive disjunction
The logical operation exclusive disjunction, also called exclusive or , is a type of logical disjunction on two operands that results in a value of true if exactly one of the operands has a value of true...
0xFF) + 1) AND 0xFF)
An 8-bit LRC such as this is equivalent to a cyclic redundancy check
Cyclic redundancy check
A cyclic redundancy check is an error-detecting code commonly used in digital networks and storage devices to detect accidental changes to raw data...
using the polynomial x8+1, but the independence of the bit streams is less clear when looked at that way.
Many protocols use such an XOR-based longitudinal redundancy check byte (often called Block Check Character or BCC), including the IEC 62056
IEC 62056
DLMS or Device Language Message Specification , is the suite of standards developed and maintained by the DLMS User Association and has been co-opted by the IEC TC13 WG14 into the IEC 62056 series of standards.COSEM or Companion Specification for Energy Metering, includes a set of specifications...
-21 standard for electrical meter reading, smart cards as defined in ISO 7816
ISO 7816
ISO/IEC 7816 is an international standard related to electronic identification cards with contacts, especially smart cards, managed jointly by the International Organization for Standardization and the International Electrotechnical Commission ....
, and the ACCESS.bus
ACCESS.bus
ACCESS.bus is a peripheral-interconnect computer bus developed by Philips in the early 1990s. It is similar in purpose to USB, in that it allows low-speed devices to be added or removed from a computer on the fly...
protocol.
C#
////// Longitudinal Redundancy Check (LRC) calculator for a byte array. Warning: This code has no warranty. Check it, and fix it before using.
///
public static byte calculateLRC(byte[] bytes)
{
/* https://gist.github.com/953550
* http://en.wikipedia.org/wiki/Longitudinal_redundancy_check
*/
byte LRC = (byte)0;
for (int i = 0; i < bytes.Length; i++)
{
LRC ^= bytes[i];
}
return LRC;
}
Java
/*** Calculates the checksum in compliance with the ISO 1155 standard
* More info: http://en.wikipedia.org/wiki/Longitudinal_redundancy_check
* @param data array to calculate checksum for
* @return returns the calculated checksum in byte format
*/
public byte calculateLRC(byte[] data) {
byte checksum = 0;
for (int i = 0; i <= data.length - 1; i++) {
checksum = (byte) ((checksum + data[i]) & 0xFF);
}
checksum = (byte) (((checksum ^ 0xFF) + 1) & 0xFF);
return checksum;
}