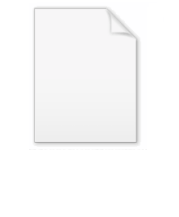
Refactoring
Encyclopedia
Code refactoring is "disciplined technique for restructuring an existing body of code, altering its internal structure without changing its external behavior", undertaken in order to improve some of the nonfunctional attributes of the software.
Typically, this is done by applying series of "refactorings", each of which is a (usually) tiny change in a computer program
's source code
that does not modify its functional requirements. Advantages include improved code readability
and reduced complexity
to improve the maintainability
of the source code, as well as a more expressive internal architecture
or object model
to improve extensibility
.
. For example the method at hand may be very long, or it may be a near duplicate of another nearby method. Once recognized, such problems can be addressed by refactoring the source code
, or transforming it into a new form that behaves the same as before but that no longer "smells
". For a long routine, extract one or more smaller subroutines. Or for duplicate routines, remove the duplication and utilize one shared function in their place. Failure to perform refactoring can result in accumulating technical debt
.
There are two general categories of benefits to the activity of refactoring.
Before refactoring a section of code, a solid set of automatic unit tests is needed. The tests should demonstrate in a few seconds that the behavior of the module is correct. The process is then an iterative cycle of making a small program transformation
, testing it to ensure correctness, and making another small transformation. If at any point a test fails, you undo your last small change and try again, possibly in a different way. Through many small steps the program moves from where it was to where you want it to be. Proponents of extreme programming
and other agile methodologies
describe this activity as an integral part of the software development cycle
.
s (HDLs) has also been refactored. The term hardware refactoring is used as a shorthand term for refactoring of code in hardware description languages. Since HDLs are not considered to be programming language
s by most hardware engineers, hardware refactoring is to be considered a separate field from traditional code refactoring.
Automated refactoring of analog hardware descriptions (in VHDL-AMS
) has been proposed by Zeng and Huss. In their approach, refactoring preserves the simulated behavior of a hardware design. The non-functional measurement that improves is that refactored code can be processed by standard synthesis tools, while the original code cannot.
Refactoring of digital HDLs, albeit manual refactoring, has also been investigated by Synopsys
fellow
Mike Keating. His target is to make complex systems easier to understand, which increases
the designers' productivity.
In the summer of 2008, there was an intense discussion about refactoring of VHDL code on the news://comp.lang.vhdl newsgroup
. The discussion revolved around a specific manual refactoring performed by one engineer, and the question to whether or not automated tools for such refactoring exist.
As of late 2009, Sigasi is offering automated tool support for VHDL refactoring.
AMIQ DVT, an IDE for hardware design and verification, provides refactoring capabilities for e (verification language)
, SystemVerilog
, Verilog
and VHDL.
Although refactoring code has been done informally for years, William Opdyke
's 1992 Ph.D. dissertation is the first known paper to specifically examine refactoring, although all the theory and machinery have long been available as program transformation
systems. All of these resources provide a catalog of common methods for refactoring; a refactoring method has a description of how to apply the method
and indicator
s for when you should (or should not) apply the method.
Martin Fowler
's book Refactoring: Improving the Design of Existing Code is the canonical reference.
The first known use of the term "refactoring" in the published literature was in a September, 1990 article by William F. Opdyke and Ralph E. Johnson.
Opdyke's Ph.D. thesis, published in 1992, also used this term.
The term "factoring" has been used in the Forth community since at least the early 1980s. Chapter Six of Leo Brodie's book Thinking Forth (1984) is dedicated to the subject.
In extreme programming, the Extract Method refactoring technique has essentially the same meaning as factoring in Forth; to break down a "word" (or function) into smaller, more easily maintained functions.
and IDEs
have automated refactoring support. Here is a list of a few of these editors, or so-called refactoring browsers.
Typically, this is done by applying series of "refactorings", each of which is a (usually) tiny change in a computer program
Computer program
A computer program is a sequence of instructions written to perform a specified task with a computer. A computer requires programs to function, typically executing the program's instructions in a central processor. The program has an executable form that the computer can use directly to execute...
's source code
Source code
In computer science, source code is text written using the format and syntax of the programming language that it is being written in. Such a language is specially designed to facilitate the work of computer programmers, who specify the actions to be performed by a computer mostly by writing source...
that does not modify its functional requirements. Advantages include improved code readability
Readability
Readability is the ease in which text can be read and understood. Various factors to measure readability have been used, such as "speed of perception," "perceptibility at a distance," "perceptibility in peripheral vision," "visibility," "the reflex blink technique," "rate of work" , "eye...
and reduced complexity
Cyclomatic complexity
Cyclomatic complexity is a software metric . It was developed by Thomas J. McCabe, Sr. in 1976 and is used to indicate the complexity of a program. It directly measures the number of linearly independent paths through a program's source code...
to improve the maintainability
Maintainability
In engineering, maintainability is the ease with which a product can be maintained in order to:* isolate defects or their cause* correct defects or their cause* meet new requirements* make future maintenance easier, or* cope with a changed environment...
of the source code, as well as a more expressive internal architecture
Software architecture
The software architecture of a system is the set of structures needed to reason about the system, which comprise software elements, relations among them, and properties of both...
or object model
Object model
In computing, object model has two related but distinct meanings:# The properties of objects in general in a specific computer programming language, technology, notation or methodology that uses them. For example, the Java objects model, the COM object model, or the object model of OMT...
to improve extensibility
Extensibility
In software engineering, extensibility is a system design principle where the implementation takes into consideration future growth. It is a systemic measure of the ability to extend a system and the level of effort required to implement the extension...
.
Overview
Refactoring is usually motivated by noticing a code smellCode smell
In computer programming, code smell is any symptom in the source code of a program that possibly indicates a deeper problem.Often the deeper problem hinted by a code smell can be uncovered when the code is subjected to a short feedback cycle where it is refactored in small, controlled steps, and...
. For example the method at hand may be very long, or it may be a near duplicate of another nearby method. Once recognized, such problems can be addressed by refactoring the source code
Source code
In computer science, source code is text written using the format and syntax of the programming language that it is being written in. Such a language is specially designed to facilitate the work of computer programmers, who specify the actions to be performed by a computer mostly by writing source...
, or transforming it into a new form that behaves the same as before but that no longer "smells
Code smell
In computer programming, code smell is any symptom in the source code of a program that possibly indicates a deeper problem.Often the deeper problem hinted by a code smell can be uncovered when the code is subjected to a short feedback cycle where it is refactored in small, controlled steps, and...
". For a long routine, extract one or more smaller subroutines. Or for duplicate routines, remove the duplication and utilize one shared function in their place. Failure to perform refactoring can result in accumulating technical debt
Technical debt
Technical debt are synonymous, neologistic metaphors referring to the eventual consequences of poor software architecture and software development within a codebase....
.
There are two general categories of benefits to the activity of refactoring.
- Maintainability. It is easier to fix bugs because the source code is easy to read and the intent of its author is easy to grasp. This might be achieved by reducing large monolithic routines into a set of individually concise, well-named, single-purpose methods. It might be achieved by moving a method to a more appropriate class, or by removing misleading comments.
- Extensibility. It is easier to extend the capabilities of the application if it uses recognizable design patternsDesign PatternsDesign Patterns: Elements of Reusable Object-Oriented Software is a software engineering book describing recurring solutions to common problems in software design. The book's authors are Erich Gamma, Richard Helm, Ralph Johnson and John Vlissides with a foreword by Grady Booch. The authors are...
, and it provides some flexibility where none before may have existed.
Before refactoring a section of code, a solid set of automatic unit tests is needed. The tests should demonstrate in a few seconds that the behavior of the module is correct. The process is then an iterative cycle of making a small program transformation
Program transformation
A program transformation is any operation that takes a computer program and generates another program. In many cases the transformed program is required to be semantically equivalent to the original, relative to a particular formal semantics and in fewer cases the transformations result in programs...
, testing it to ensure correctness, and making another small transformation. If at any point a test fails, you undo your last small change and try again, possibly in a different way. Through many small steps the program moves from where it was to where you want it to be. Proponents of extreme programming
Extreme Programming
Extreme programming is a software development methodology which is intended to improve software quality and responsiveness to changing customer requirements...
and other agile methodologies
Agile software development
Agile software development is a group of software development methodologies based on iterative and incremental development, where requirements and solutions evolve through collaboration between self-organizing, cross-functional teams...
describe this activity as an integral part of the software development cycle
Software development process
A software development process, also known as a software development life cycle , is a structure imposed on the development of a software product. Similar terms include software life cycle and software process. It is often considered a subset of systems development life cycle...
.
List of refactoring techniques
Here are some examples of code refactorings; some of these may only apply to certain languages or language types. A longer list can be found in Fowler's Refactoring book and on Fowler's Refactoring Website.- Techniques that allow for more abstractionAbstraction (computer science)In computer science, abstraction is the process by which data and programs are defined with a representation similar to its pictorial meaning as rooted in the more complex realm of human life and language with their higher need of summarization and categorization , while hiding away the...
- Encapsulate Field – force code to access the field with getter and setter methods
- Generalize TypeGeneralize TypeType generalization is a technique commonly used in refactoring. The idea is to draw on the benefits of object-orientation and make more-generalized types, thus enabling more code sharing, leading to better maintainability as there is less code to write...
– create more general types to allow for more code sharing - Replace type-checking code with State/Strategy
- Replace conditional with polymorphism
- Techniques for breaking code apart into more logical pieces
- Extract Method, to turn part of a larger methodMethod (computer science)In object-oriented programming, a method is a subroutine associated with a class. Methods define the behavior to be exhibited by instances of the associated class at program run time...
into a new method. By breaking down code in smaller pieces, it is more easily understandable. This is also applicable to functions. - Extract ClassExtract classIn Software engineering, the Extract class refactoring is applied when a class becomes overweight with too many methods and its purpose becomes unclear. It involves creating a new class and moving methods and/or data to the new class.-Further reading:...
moves part of the code from an existing class into a new class.
- Extract Method, to turn part of a larger method
- Techniques for improving names and location of code
- Move Method or Move Field – move to a more appropriate ClassClass (computer science)In object-oriented programming, a class is a construct that is used as a blueprint to create instances of itself – referred to as class instances, class objects, instance objects or simply objects. A class defines constituent members which enable these class instances to have state and behavior...
or source file - Rename MethodRename methodRename Method is a refactoring that changes a name of a method into a new one, that better reveals its purpose.To have clearer, more understandable code, programmers would optimally want to change method names to reflect exactly what the method does...
or Rename Field – changing the name into a new one that better reveals its purpose - Pull UpPull Up refactoringIn software engineering, Pull Up refactoring involves moving a member of a class, such as a method, from a Subclass into a Superclass.-How and when can this refactoring be applied?:...
– in OOPObject-oriented programmingObject-oriented programming is a programming paradigm using "objects" – data structures consisting of data fields and methods together with their interactions – to design applications and computer programs. Programming techniques may include features such as data abstraction,...
, move to a superclass - Push Down – in OOPObject-oriented programmingObject-oriented programming is a programming paradigm using "objects" – data structures consisting of data fields and methods together with their interactions – to design applications and computer programs. Programming techniques may include features such as data abstraction,...
, move to a subclass
- Move Method or Move Field – move to a more appropriate Class
Hardware refactoring
While the term refactoring originally referred exclusively to refactoring of software code, in recent years code written in hardware description languageHardware description language
In electronics, a hardware description language or HDL is any language from a class of computer languages, specification languages, or modeling languages for formal description and design of electronic circuits, and most-commonly, digital logic...
s (HDLs) has also been refactored. The term hardware refactoring is used as a shorthand term for refactoring of code in hardware description languages. Since HDLs are not considered to be programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
s by most hardware engineers, hardware refactoring is to be considered a separate field from traditional code refactoring.
Automated refactoring of analog hardware descriptions (in VHDL-AMS
VHDL-AMS
VHDL-AMS is a derivative of the hardware description language VHDL . It includes analog and mixed-signal extensions in order to define the behavior of analog and mixed-signal systems ....
) has been proposed by Zeng and Huss. In their approach, refactoring preserves the simulated behavior of a hardware design. The non-functional measurement that improves is that refactored code can be processed by standard synthesis tools, while the original code cannot.
Refactoring of digital HDLs, albeit manual refactoring, has also been investigated by Synopsys
Synopsys
Synopsys, Inc. is one of the largest companies in the Electronic Design Automation industry. Synopsys' first and best-known product is Design Compiler, a logic-synthesis tool. Synopsys offers a wide range of other products used in the design of an application-specific integrated circuit...
fellow
Fellow
A fellow in the broadest sense is someone who is an equal or a comrade. The term fellow is also used to describe a person, particularly by those in the upper social classes. It is most often used in an academic context: a fellow is often part of an elite group of learned people who are awarded...
Mike Keating. His target is to make complex systems easier to understand, which increases
the designers' productivity.
In the summer of 2008, there was an intense discussion about refactoring of VHDL code on the news://comp.lang.vhdl newsgroup
Newsgroup
A usenet newsgroup is a repository usually within the Usenet system, for messages posted from many users in different locations. The term may be confusing to some, because it is usually a discussion group. Newsgroups are technically distinct from, but functionally similar to, discussion forums on...
. The discussion revolved around a specific manual refactoring performed by one engineer, and the question to whether or not automated tools for such refactoring exist.
As of late 2009, Sigasi is offering automated tool support for VHDL refactoring.
AMIQ DVT, an IDE for hardware design and verification, provides refactoring capabilities for e (verification language)
E (verification language)
e is a hardware verification language which is tailored to implementing highly flexible and reusable verification testbenches.- History :...
, SystemVerilog
SystemVerilog
In the semiconductor and electronic design industry, SystemVerilog is a combined Hardware Description Language and Hardware Verification Language based on extensions to Verilog.-History:...
, Verilog
Verilog
In the semiconductor and electronic design industry, Verilog is a hardware description language used to model electronic systems. Verilog HDL, not to be confused with VHDL , is most commonly used in the design, verification, and implementation of digital logic chips at the register-transfer level...
and VHDL.
History
In the past refactoring was avoided in development processes. One example of this is that CVS (created in 1984) does not version the moving or renaming of files and directories.Although refactoring code has been done informally for years, William Opdyke
William Opdyke
William F. Opdyke is a computer scientist. His 1992 Ph.D. thesis from the University of Illinois at Urbana–Champaign, Refactoring Object-Oriented Frameworks, was the first in-depth study of code refactoring as a software engineering technique...
's 1992 Ph.D. dissertation is the first known paper to specifically examine refactoring, although all the theory and machinery have long been available as program transformation
Program transformation
A program transformation is any operation that takes a computer program and generates another program. In many cases the transformed program is required to be semantically equivalent to the original, relative to a particular formal semantics and in fewer cases the transformations result in programs...
systems. All of these resources provide a catalog of common methods for refactoring; a refactoring method has a description of how to apply the method
Scientific method
Scientific method refers to a body of techniques for investigating phenomena, acquiring new knowledge, or correcting and integrating previous knowledge. To be termed scientific, a method of inquiry must be based on gathering empirical and measurable evidence subject to specific principles of...
and indicator
Indicator
Indicator may mean:In chemistry:* pH indicator, a chemical detector for protons in acid-base titrations* Redox indicator, a chemical detector for redox titrations* Complexometric indicator, a chemical detector for metal ions in complexometric titrations...
s for when you should (or should not) apply the method.
Martin Fowler
Martin Fowler
-Online presentations:* at RailsConf 2006* at JAOO 2006* at QCon London 2007 * at QCon London 2008 * at ThoughtWorks Quarterly Technology Briefing, October 2008...
's book Refactoring: Improving the Design of Existing Code is the canonical reference.
The first known use of the term "refactoring" in the published literature was in a September, 1990 article by William F. Opdyke and Ralph E. Johnson.
Opdyke's Ph.D. thesis, published in 1992, also used this term.
The term "factoring" has been used in the Forth community since at least the early 1980s. Chapter Six of Leo Brodie's book Thinking Forth (1984) is dedicated to the subject.
In extreme programming, the Extract Method refactoring technique has essentially the same meaning as factoring in Forth; to break down a "word" (or function) into smaller, more easily maintained functions.
Automated code refactoring
Many software editorsText editor
A text editor is a type of program used for editing plain text files.Text editors are often provided with operating systems or software development packages, and can be used to change configuration files and programming language source code....
and IDEs
Integrated development environment
An integrated development environment is a software application that provides comprehensive facilities to computer programmers for software development...
have automated refactoring support. Here is a list of a few of these editors, or so-called refactoring browsers.
- IntelliJ IDEAIntelliJ IDEAIntelliJ IDEA is a commercial Java IDE by JetBrains. It is often simply referred to as "IDEA" or "IntelliJ."-History:The first version of IntelliJ IDEA was released in January 2001, and at the time was the only available Java IDE with advanced code navigation and code refactoring capabilities...
(for JavaJava (programming language)Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
) - Eclipse'sEclipse (software)Eclipse is a multi-language software development environment comprising an integrated development environment and an extensible plug-in system...
JavaJava (programming language)Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
Development Toolkit (JDT) - NetBeansNetBeansNetBeans refers to both a platform framework for Java desktop applications, and an integrated development environment for developing with Java, JavaScript, PHP, Python, Groovy, C, C++, Scala, Clojure, and others...
(for Java)- and RefactoringNG, a Netbeans module for refactoring where you can write transformationsSource-to-source compilerA source-to-source compiler is a type of compiler that takes a high level programming language as its input and outputs a high level language. For example, an automatic parallelizing compiler will frequently take in a high level language program as an input and then transform the code and annotate...
rules of the program's abstract syntax treeAbstract syntax treeIn computer science, an abstract syntax tree , or just syntax tree, is a tree representation of the abstract syntactic structure of source code written in a programming language. Each node of the tree denotes a construct occurring in the source code. The syntax is 'abstract' in the sense that it...
.
- and RefactoringNG, a Netbeans module for refactoring where you can write transformations
- Embarcadero Delphi
- Visual Studio (for .NET)
- JustCodeJustCodeJustCode is a refactoring and code analysis productivity plug-in for Microsoft Visual Studio .NET 2005, 2008 and 2010. JustCode is developed by Telerik and launched in 2009...
(addon for Visual Studio) - ReSharperReSharperReSharper is a refactoring and productivity extension by JetBrains that extends native functionality of Microsoft Visual Studio 2003, 2005, 2008 and 2010....
(addon for Visual Studio) - CoderushCodeRushCodeRush is a refactoring and productivity plugin by DevExpress that extends native functionality of Microsoft Visual Studio .NET 2003, 2005, 2008 and 2010....
(addon for Visual Studio) - Visual AssistVisual AssistVisual Assist X is a plugin for Microsoft Visual Studio developed by Whole Tomato. It primarily enhances IntelliSense and syntax highlighting. It also enhances code suggestions, refactoring options and spell checking support for comments. It can also detect basic syntax mistakes, such as use of...
(addon for Visual Studio with refactoring support for VB, VB.NET. C# and C++) - DMS Software Reengineering ToolkitDMS Software Reengineering ToolkitThe DMS Software Reengineering Toolkit is a proprietary set of program transformation tools available for automating custom source program analysis, modification, translation or generation of software systems for arbitrary mixtures of source languages for large scale software systems.DMS has been...
(Implements large-scale refactoring for C, C++, C#, COBOL, Java, PHP and other languages) - PhotranPhotranPhotran is an Integrated Development Environment for Fortran 77, 90, 95, and 2003 based on Eclipse and the CDT. The project is maintained by the University of Illinois at Urbana-Champaign and IBM.-See also:* KDevelop...
(a FortranFortranFortran is a general-purpose, procedural, imperative programming language that is especially suited to numeric computation and scientific computing...
plugin for the Eclipse IDEEclipse (software)Eclipse is a multi-language software development environment comprising an integrated development environment and an extensible plug-in system...
) - SharpSort addin for Visual Studio 2008
- Sigasi HDT (for VHDL)
- XcodeXcodeXcode is a suite of tools, developed by Apple, for developing software for Mac OS X and iOS. Xcode 4.2, the latest major version, is available on the Mac App Store for free for Mac OS X 10.7 , and on the Apple Developer Connection website for free to registered developers Xcode is a suite of tools,...
- Smalltalk Refactoring Browser (for SmalltalkSmalltalkSmalltalk is an object-oriented, dynamically typed, reflective programming language. Smalltalk was created as the language to underpin the "new world" of computing exemplified by "human–computer symbiosis." It was designed and created in part for educational use, more so for constructionist...
) - Simplifide (for Verilog, VHDL and SystemVerilog)
- Tidier (for ErlangErlangErlang may refer to:* Agner Krarup Erlang , a mathematician and engineer after whom several concepts are named** Erlang , a unit to measure traffic in telecommunications or other domains...
) - AMIQ DVT (for e, SystemVerilog, Verilog and VHDL)
See also
- Code reviewCode reviewCode review is systematic examination of computer source code. It is intended to find and fix mistakes overlooked in the initial development phase, improving both the overall quality of software and the developers' skills...
- Database refactoringDatabase refactoringA database refactoring is a simple change to a database schema that improves its design while retaining both its behavioral and informational semantics...
- Design pattern (computer science)Design pattern (computer science)In software engineering, a design pattern is a general reusable solution to a commonly occurring problem within a given context in software design. A design pattern is not a finished design that can be transformed directly into code. It is a description or template for how to solve a problem that...
- Obfuscated codeObfuscated codeObfuscated code is source or machine code that has been made difficult to understand for humans. Programmers may deliberately obfuscate code to conceal its purpose or its logic to prevent tampering, deter reverse engineering, or as a puzzle or recreational challenge for someone reading the source...
- Software peer reviewSoftware peer reviewIn software development, peer review is a type of software review in which a work product is examined by its author and one or more colleagues, in order to evaluate its technical content and quality.-Purpose:...
- PrefactoringPrefactoringPrefactoring is the application of past experience to the creation of new software systems. Its relationship to its namesake refactoring is that lessons learned from refactoring are part of that experience....
- Rewrite (programming)Rewrite (programming)A rewrite in computer programming is the act or result of re-implementing a large portion of existing functionality without re-use of its source code. When the rewrite is not using existing code at all, it is common to speak of a rewrite from scratch...
- Separation of concernsSeparation of concernsIn computer science, separation of concerns is the process of separating a computer program into distinct features that overlap in functionality as little as possible. A concern is any piece of interest or focus in a program. Typically, concerns are synonymous with features or behaviors...
- Test-driven developmentTest-driven developmentTest-driven development is a software development process that relies on the repetition of a very short development cycle: first the developer writes a failing automated test case that defines a desired improvement or new function, then produces code to pass that test and finally refactors the new...
- Modular programmingModular programmingModular programming is a software design technique that increases the extent to which software is composed of separate, interchangeable components called modules by breaking down program functions into modules, each of which accomplishes one function and contains everything necessary to accomplish...
- Redesign (software)
Further reading
- Mens, Tom and Tourwé, Tom (2004) A Survey of Software Refactoring, IEEE Transactions on Software Engineering, February 2004 (vol. 30 no. 2), pp. 126-139
External links
- What Is Refactoring? (c2.com article)
- Martin Fowler's homepage about refactoring
- Aspect-Oriented Refactoring by Ramnivas Laddad
- A Survey of Software Refactoring by Tom Mens and Tom Tourwé
- Refactoring Java Code
- Refactoring To Patterns Catalog
- Extract Boolean Variable from Conditional (a refactoring pattern not listed in the above catalog)
- Test-Driven Development With Refactoring
- Revisiting Fowler’s Video Store: Refactoring Code, Refining Abstractions