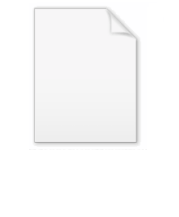
Event loop
Encyclopedia
In computer science
, the event loop, message dispatcher, message loop, message pump, or run loop is a programming construct that waits for and dispatches events
or messages
in a program
. It works by polling
some internal or external "event provider", which generally blocks
until an event has arrived, and then calls the relevant event handler ("dispatches the event"). The event-loop may be used in conjunction with a reactor
, if the event provider follows the file interface (which can be selected or polled). The event loop almost always operates asynchronously with the message originator.
When the event loop forms the central control flow construct of a program, as it often does, and is thus at the highest level of control within the program, it may be termed the main loop or main event loop.
(assigned and usually owned by the underlying operating system) into the program for processing. In the strictest sense, an event loop is one of the methods for implementing inter-process communication
. In fact, message processing exists in many systems, including a kernel-level component of the Mach operating system. The event loop is a specific implementation technique of systems that use message passing
.
interfaces, most modern applications feature a main loop. The get_next_message routine is typically provided by the operating system, and blocks
until a message is available. Thus, the loop is only entered when there is something to process.
function main
initialize
while message != quit
message := get_next_message
process_message(message)
end while
end function
, the "everything is a file" paradigm naturally leads to a file-based event loop. Reading from and writing to files, inter-process communication, network communication and device control are all achieved using file I/O, with the target identified by a file descriptor
. The select
and poll system calls allow a set of file descriptors to be monitored for a change of state, e.g. when data becomes available to be read.
For example, consider a program that reads from a continuously updated file and displays its contents in the X Window System
, which communicates with clients over a socket (either Unix domain
or Berkeley
):
main:
file_fd = open ("logfile")
x_fd = open_display
construct_interface
while changed_fds = select ({file_fd, x_fd}):
if file_fd in changed_fds:
data = read_from (file_fd)
append_to_display (data)
send_repaint_message
if x_fd in changed_fds:
process_x_messages
). Signals are received in signal handlers, small, limited pieces of code that run while the rest of the task is suspended; if a signal is received and handled while the task is blocking in
then the task will be suspended between instructions until the signal handler returns.
Thus an obvious way to handle signals is for signal handlers to set a global flag and have the event loop check for the flag immediately before and after the
: if a signal arrives immediately between checking the flag and calling
The solution arrived at by POSIX
is the pselect call, which is similar to
, forcing glibc to emulate it via a method prone to the very same race condition
An alternative, more portable solution, is to convert asynchronous events to file-based events using the self-pipe trick, where "a signal handler writes a byte to a pipe whose other end is monitored by select in the main program". In Linux kernel
version 2.6.22, a new system call signalfd was added, which allows receiving signals via a special file descriptor.
operating system requires user-interactive processes that wish to run on the operating system to construct a message loop
for responding to events. In this operating system, a message is equated to an event created and imposed upon the operating system. An event can range from user interaction, network traffic, system processing, timer activity, and interprocess communication among others. For non-interactive, I/O only events, Windows has IO Completion Ports
. IO Completion Port loops run separately from the Message loop, and do not interact with the Message loop out of the box.
The "heart" of most Win32 applications
is the WinMain function, which calls GetMessage, in a loop. GetMessage blocks until a message, or "event", is received. After some optional processing, it will call DispatchMessage, which dispatches the message to the relevant handler, also known as WindowProc
. Normally, messages that have no special WindowProc are dispatched to DefWindowProc, the default one. DispatchMessage calls the window-proc of the HWND handle
of the message (Registered with the RegisterClass function).
applications.
However, some messages have different rules, such as messages that are always received last, or messages with a different documented priority.
applications using Xlib
directly are built around the
.
Very few programs use Xlib directly. In the more common case, GUI toolkits based on Xlib usually support adding events. For example, toolkits based on Xt
intrinsics have
Please note that it is not safe to call Xlib functions from a signal handler, because the X application may have been interrupted in an arbitrary state, e.g. within
event loop was originally created for use in GTK+
but is now used in non-GUI applications as well, such as D-Bus
. The resource polled is the collection of file descriptor
s the application is interested in; the polling block will be interrupted if a signal
arrives or a timeout expires (e.g. if the application has specified a timeout or idle task). While GLib has built-in support for file descriptor and child termination events, it is possible to add an event source for any event that can be handled in a prepare-check-dispatch model.http://developer.gnome.org/glib/2.30/glib-The-Main-Event-Loop.html#mainloop-states
Application libraries that are built on the GLib event loop include GStreamer
and the asynchronous I/O
methods of GnomeVFS, but GTK+
remains the most visible client library. Events from the windowing system
(in X
, read off the X socket
) are translated by GDK
into GTK+ events and emitted as GLib signals on the application's widget objects.
The CFRunLoop is abstracted in Cocoa
as an NSRunLoop, which allows any message (equivalent to a function call in non-reflective
runtimes) to be queued for dispatch to any object.
Computer science
Computer science or computing science is the study of the theoretical foundations of information and computation and of practical techniques for their implementation and application in computer systems...
, the event loop, message dispatcher, message loop, message pump, or run loop is a programming construct that waits for and dispatches events
Event-driven programming
In computer programming, event-driven programming or event-based programming is a programming paradigm in which the flow of the program is determined by events—i.e., sensor outputs or user actions or messages from other programs or threads.Event-driven programming can also be defined as an...
or messages
Message passing
Message passing in computer science is a form of communication used in parallel computing, object-oriented programming, and interprocess communication. In this model, processes or objects can send and receive messages to other processes...
in a program
Computer program
A computer program is a sequence of instructions written to perform a specified task with a computer. A computer requires programs to function, typically executing the program's instructions in a central processor. The program has an executable form that the computer can use directly to execute...
. It works by polling
Polling (computer science)
Polling, or polled operation, in computer science, refers to actively sampling the status of an external device by a client program as a synchronous activity. Polling is most often used in terms of input/output , and is also referred to as polled or software driven .Polling is sometimes used...
some internal or external "event provider", which generally blocks
Blocking (computing)
Blocking occurs when a subroutine does not return until it either completes its task or fails with an error or exception. A process that is blocked is one that waits for some event, such as a resource becoming available or the completion of an I/O operation.In a multitasking computer system,...
until an event has arrived, and then calls the relevant event handler ("dispatches the event"). The event-loop may be used in conjunction with a reactor
Reactor pattern
The reactor design pattern is an event handling pattern for handling service requests delivered concurrently to a service handler by one or more inputs...
, if the event provider follows the file interface (which can be selected or polled). The event loop almost always operates asynchronously with the message originator.
When the event loop forms the central control flow construct of a program, as it often does, and is thus at the highest level of control within the program, it may be termed the main loop or main event loop.
Message passing
Message pumps are said to 'pump' messages from the program's message queueMessage queue
In computer science, message queues and mailboxes are software-engineering components used for interprocess communication, or for inter-thread communication within the same process. They use a queue for messaging – the passing of control or of content...
(assigned and usually owned by the underlying operating system) into the program for processing. In the strictest sense, an event loop is one of the methods for implementing inter-process communication
Inter-process communication
In computing, Inter-process communication is a set of methods for the exchange of data among multiple threads in one or more processes. Processes may be running on one or more computers connected by a network. IPC methods are divided into methods for message passing, synchronization, shared...
. In fact, message processing exists in many systems, including a kernel-level component of the Mach operating system. The event loop is a specific implementation technique of systems that use message passing
Message passing
Message passing in computer science is a form of communication used in parallel computing, object-oriented programming, and interprocess communication. In this model, processes or objects can send and receive messages to other processes...
.
Alternative designs
This approach is in contrast to a number of other alternatives:- Traditionally, a program simply ran once then terminated. This type of program was very common in the early days of computing, and lacked any form of user interactivity. This is still used frequently, particularly in the form of command line driven programs. Any parameters are set up in advance and passed in one go when the program starts.
- Menu-driven designs. These still may feature a main loop but are not usually thought of as event drivenEvent-driven programmingIn computer programming, event-driven programming or event-based programming is a programming paradigm in which the flow of the program is determined by events—i.e., sensor outputs or user actions or messages from other programs or threads.Event-driven programming can also be defined as an...
in the usual sense. Instead, the user is presented with an ever-narrowing set of options until the task they wish to carry out is the only option available. Limited interactivity through the menus is available.
Usage
Due to the predominance of GUIGui
Gui or guee is a generic term to refer to grilled dishes in Korean cuisine. These most commonly have meat or fish as their primary ingredient, but may in some cases also comprise grilled vegetables or other vegetarian ingredients. The term derives from the verb, "gupda" in Korean, which literally...
interfaces, most modern applications feature a main loop. The get_next_message routine is typically provided by the operating system, and blocks
Blocking (computing)
Blocking occurs when a subroutine does not return until it either completes its task or fails with an error or exception. A process that is blocked is one that waits for some event, such as a resource becoming available or the completion of an I/O operation.In a multitasking computer system,...
until a message is available. Thus, the loop is only entered when there is something to process.
function main
initialize
while message != quit
message := get_next_message
process_message(message)
end while
end function
File interface
Under UnixUnix
Unix is a multitasking, multi-user computer operating system originally developed in 1969 by a group of AT&T employees at Bell Labs, including Ken Thompson, Dennis Ritchie, Brian Kernighan, Douglas McIlroy, and Joe Ossanna...
, the "everything is a file" paradigm naturally leads to a file-based event loop. Reading from and writing to files, inter-process communication, network communication and device control are all achieved using file I/O, with the target identified by a file descriptor
File descriptor
In computer programming, a file descriptor is an abstract indicator for accessing a file. The term is generally used in POSIX operating systems...
. The select
Select (Unix)
select is a system call and application programming interface in Unix-like and POSIX-compliant operating systems for examining the status of file descriptors of open input/output channels...
and poll system calls allow a set of file descriptors to be monitored for a change of state, e.g. when data becomes available to be read.
For example, consider a program that reads from a continuously updated file and displays its contents in the X Window System
X Window System
The X window system is a computer software system and network protocol that provides a basis for graphical user interfaces and rich input device capability for networked computers...
, which communicates with clients over a socket (either Unix domain
Unix domain socket
A Unix domain socket or IPC socket is a data communications endpoint for exchanging data between processes executing within the same host operating system. While similar in functionality to...
or Berkeley
Berkeley sockets
The Berkeley sockets application programming interface comprises a library for developing applications in the C programming language that perform inter-process communication, most commonly for communications across a computer network....
):
main:
file_fd = open ("logfile")
x_fd = open_display
construct_interface
while changed_fds = select ({file_fd, x_fd}):
if file_fd in changed_fds:
data = read_from (file_fd)
append_to_display (data)
send_repaint_message
if x_fd in changed_fds:
process_x_messages
Handling signals
One of the few things in Unix that do not conform to the file interface are asynchronous events (signalsSignal (computing)
A signal is a limited form of inter-process communication used in Unix, Unix-like, and other POSIX-compliant operating systems. Essentially it is an asynchronous notification sent to a process in order to notify it of an event that occurred. When a signal is sent to a process, the operating system...
). Signals are received in signal handlers, small, limited pieces of code that run while the rest of the task is suspended; if a signal is received and handled while the task is blocking in
select
then select will return early with EINTR; if a signal is received while the task is CPU boundCPU bound
In computer science, CPU bound is when the time for a computer to complete a task is determined principally by the speed of the central processor: processor utilization is high, perhaps at 100% usage for many seconds or minutes...
then the task will be suspended between instructions until the signal handler returns.
Thus an obvious way to handle signals is for signal handlers to set a global flag and have the event loop check for the flag immediately before and after the
select
call; if it is set, handle the signal in the same manner as with events on file descriptors. Unfortunately, this gives rise to a race conditionRace condition
A race condition or race hazard is a flaw in an electronic system or process whereby the output or result of the process is unexpectedly and critically dependent on the sequence or timing of other events...
: if a signal arrives immediately between checking the flag and calling
select
, then it will not be handled until select
returns for some other reason (for example, being interrupted by a frustrated user).The solution arrived at by POSIX
POSIX
POSIX , an acronym for "Portable Operating System Interface", is a family of standards specified by the IEEE for maintaining compatibility between operating systems...
is the pselect call, which is similar to
select
but takes an additional sigmask
parameter, which describes a signal mask. This allows an application to mask signals in the main task, then remove the mask for the duration of the select
call, such that signal handlers are only called while the application is I/O bound. However, implementations of pselect
have only recently become reliable; versions of Linux prior to 2.6.16 do not have a pselect
system callSystem call
In computing, a system call is how a program requests a service from an operating system's kernel. This may include hardware related services , creating and executing new processes, and communicating with integral kernel services...
, forcing glibc to emulate it via a method prone to the very same race condition
pselect
is intended to avoid.An alternative, more portable solution, is to convert asynchronous events to file-based events using the self-pipe trick, where "a signal handler writes a byte to a pipe whose other end is monitored by select in the main program". In Linux kernel
Linux kernel
The Linux kernel is an operating system kernel used by the Linux family of Unix-like operating systems. It is one of the most prominent examples of free and open source software....
version 2.6.22, a new system call signalfd was added, which allows receiving signals via a special file descriptor.
Windows applications
The Microsoft WindowsMicrosoft Windows
Microsoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal...
operating system requires user-interactive processes that wish to run on the operating system to construct a message loop
Message loop in Microsoft Windows
Microsoft Windows programs are event-based. They act upon messages that the operating system posts to the main thread of the application. These messages are received from the message queue by the application by repeatedly calling the GetMessage function in a section of code called the "event...
for responding to events. In this operating system, a message is equated to an event created and imposed upon the operating system. An event can range from user interaction, network traffic, system processing, timer activity, and interprocess communication among others. For non-interactive, I/O only events, Windows has IO Completion Ports
Input/output completion port
Input/Output Completion Port is an API for performing multiple simultaneous asynchronous input/output operations in Windows NT versions 3.5 and later, AIX and on Solaris 10 and later. An input/output completion port object is created and associated with a number of sockets or file handles...
. IO Completion Port loops run separately from the Message loop, and do not interact with the Message loop out of the box.
The "heart" of most Win32 applications
Application software
Application software, also known as an application or an "app", is computer software designed to help the user to perform specific tasks. Examples include enterprise software, accounting software, office suites, graphics software and media players. Many application programs deal principally with...
is the WinMain function, which calls GetMessage, in a loop. GetMessage blocks until a message, or "event", is received. After some optional processing, it will call DispatchMessage, which dispatches the message to the relevant handler, also known as WindowProc
WindowProc
In Win32 application programming, WindowProc is a user-defined callback function that processes messages sent to a window. This function is specified when an application registers its window class, and can be named anything...
. Normally, messages that have no special WindowProc are dispatched to DefWindowProc, the default one. DispatchMessage calls the window-proc of the HWND handle
Smart pointer
In computer science, a smart pointer is an abstract data type that simulates a pointer while providing additional features, such as automatic garbage collection or bounds checking. These additional features are intended to reduce bugs caused by the misuse of pointers while retaining efficiency...
of the message (Registered with the RegisterClass function).
Message ordering
More recent versions of Microsoft Windows provide the guarantee to the programmer that messages will be delivered to an application's message loop in the order that they were perceived by the system and its peripherals. This guarantee is essential when considering the design consequences of multithreadedThread (computer science)
In computer science, a thread of execution is the smallest unit of processing that can be scheduled by an operating system. The implementation of threads and processes differs from one operating system to another, but in most cases, a thread is contained inside a process...
applications.
However, some messages have different rules, such as messages that are always received last, or messages with a different documented priority.
Xlib event loop
XX Window System
The X window system is a computer software system and network protocol that provides a basis for graphical user interfaces and rich input device capability for networked computers...
applications using Xlib
Xlib
Xlib is an X Window System protocol client library written in the C programming language. It contains functions for interacting with an X server. These functions allow programmers to write programs without knowing the details of the protocol...
directly are built around the
XNextEvent
family of functions; XNextEvent
blocks until an event appears on the event queue, whereupon the application processes it appropriately. The Xlib event loop only handles window system events; applications which need to be able to wait on other files and devices could construct their own event loop from primitives such as ConnectionNumber
, but in practice tend to use multithreadingThread (computer science)
In computer science, a thread of execution is the smallest unit of processing that can be scheduled by an operating system. The implementation of threads and processes differs from one operating system to another, but in most cases, a thread is contained inside a process...
.
Very few programs use Xlib directly. In the more common case, GUI toolkits based on Xlib usually support adding events. For example, toolkits based on Xt
Intrinsics
X Toolkit Intrinsics is a library used in the X Window System. More precisely, it is a library that uses the low-level Xlib library and provides a friendly API to develop X11 software with graphical widgets...
intrinsics have
XtAppAddInput
and XtAppAddTimeout
.Please note that it is not safe to call Xlib functions from a signal handler, because the X application may have been interrupted in an arbitrary state, e.g. within
XNextEvent
. See http://www.ist.co.uk/motif/books/vol6A/ch-26.fm.html for a solution for X11R5, X11R6 and Xt.GLib event loop
The GLibGLib
GLib is a cross-platform software utility library that began as part of the GTK+ project. However, before releasing version 2 of GTK+, the project's developers decided to separate non-GUI-specific code from the GTK+ platform, thus creating GLib as a separate product...
event loop was originally created for use in GTK+
GTK+
GTK+ is a cross-platform widget toolkit for creating graphical user interfaces. It is licensed under the terms of the GNU LGPL, allowing both free and proprietary software to use it. It is one of the most popular toolkits for the X Window System, along with Qt.The name GTK+ originates from GTK;...
but is now used in non-GUI applications as well, such as D-Bus
D-Bus
In computing, D-Bus is a simple inter-process communication open-source system for software applications to communicate with one another. Heavily influenced by KDE2–3's DCOP system, D-Bus has replaced DCOP in the KDE 4 release. An implementation of D-Bus supports most POSIX operating...
. The resource polled is the collection of file descriptor
File descriptor
In computer programming, a file descriptor is an abstract indicator for accessing a file. The term is generally used in POSIX operating systems...
s the application is interested in; the polling block will be interrupted if a signal
Signal (computing)
A signal is a limited form of inter-process communication used in Unix, Unix-like, and other POSIX-compliant operating systems. Essentially it is an asynchronous notification sent to a process in order to notify it of an event that occurred. When a signal is sent to a process, the operating system...
arrives or a timeout expires (e.g. if the application has specified a timeout or idle task). While GLib has built-in support for file descriptor and child termination events, it is possible to add an event source for any event that can be handled in a prepare-check-dispatch model.http://developer.gnome.org/glib/2.30/glib-The-Main-Event-Loop.html#mainloop-states
Application libraries that are built on the GLib event loop include GStreamer
GStreamer
GStreamer is a pipeline-based multimedia framework written in the C programming language with the type system based on GObject.GStreamer allows a programmer to create a variety of media-handling components, including simple audio playback, audio and video playback, recording, streaming and editing...
and the asynchronous I/O
Asynchronous I/O
Asynchronous I/O, or non-blocking I/O, is a form of input/output processing that permits other processing to continue before the transmission has finished....
methods of GnomeVFS, but GTK+
GTK+
GTK+ is a cross-platform widget toolkit for creating graphical user interfaces. It is licensed under the terms of the GNU LGPL, allowing both free and proprietary software to use it. It is one of the most popular toolkits for the X Window System, along with Qt.The name GTK+ originates from GTK;...
remains the most visible client library. Events from the windowing system
Windowing system
A windowing system is a component of a graphical user interface , and more specifically of a desktop environment, which supports the implementation of window managers, and provides basic support for graphics hardware, pointing devices such as mice, and keyboards...
(in X
X Window System
The X window system is a computer software system and network protocol that provides a basis for graphical user interfaces and rich input device capability for networked computers...
, read off the X socket
Unix domain socket
A Unix domain socket or IPC socket is a data communications endpoint for exchanging data between processes executing within the same host operating system. While similar in functionality to...
) are translated by GDK
GDK
GDK is a computer graphics library that acts as a wrapper around the low-level drawing and windowing functions provided by the underlying graphics system...
into GTK+ events and emitted as GLib signals on the application's widget objects.
Core Foundation run loops
Exactly one CFRunLoop is allowed per thread, and arbitrarily many sources and observers can be attached. Sources then communicate with observers through the run loop, with it organising queueing and dispatch of messages.The CFRunLoop is abstracted in Cocoa
Cocoa (API)
Cocoa is Apple's native object-oriented application programming interface for the Mac OS X operating system and—along with the Cocoa Touch extension for gesture recognition and animation—for applications for the iOS operating system, used on Apple devices such as the iPhone, the iPod Touch, and...
as an NSRunLoop, which allows any message (equivalent to a function call in non-reflective
Reflection (computer science)
In computer science, reflection is the process by which a computer program can observe and modify its own structure and behavior at runtime....
runtimes) to be queued for dispatch to any object.
See also
- Message passingMessage passingMessage passing in computer science is a form of communication used in parallel computing, object-oriented programming, and interprocess communication. In this model, processes or objects can send and receive messages to other processes...
- Inter-process communicationInter-process communicationIn computing, Inter-process communication is a set of methods for the exchange of data among multiple threads in one or more processes. Processes may be running on one or more computers connected by a network. IPC methods are divided into methods for message passing, synchronization, shared...
- Asynchronous I/OAsynchronous I/OAsynchronous I/O, or non-blocking I/O, is a form of input/output processing that permits other processing to continue before the transmission has finished....
- The game loop in Game programmingGame programmingGame programming, a subset of game development, is the programming of computer, console or arcade games. Though often engaged in by professional game programmers, many novices may program games as a hobby...