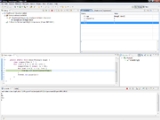
Breakpoint
Encyclopedia
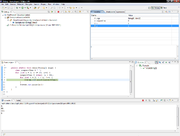
Software development
Software development is the development of a software product...
, a breakpoint is an intentional stopping or pausing place in a program
Computer program
A computer program is a sequence of instructions written to perform a specified task with a computer. A computer requires programs to function, typically executing the program's instructions in a central processor. The program has an executable form that the computer can use directly to execute...
, put in place for debugging
Debugging
Debugging is a methodical process of finding and reducing the number of bugs, or defects, in a computer program or a piece of electronic hardware, thus making it behave as expected. Debugging tends to be harder when various subsystems are tightly coupled, as changes in one may cause bugs to emerge...
purposes. It is also sometimes simply referred to as a pause.
More generally, a breakpoint is a means of acquiring knowledge about a program during its execution. During the interruption, the programmer
Programmer
A programmer, computer programmer or coder is someone who writes computer software. The term computer programmer can refer to a specialist in one area of computer programming or to a generalist who writes code for many kinds of software. One who practices or professes a formal approach to...
inspects the test environment (general purpose registers, memory
Virtual memory
In computing, virtual memory is a memory management technique developed for multitasking kernels. This technique virtualizes a computer architecture's various forms of computer data storage , allowing a program to be designed as though there is only one kind of memory, "virtual" memory, which...
, logs, files
Computer file
A computer file is a block of arbitrary information, or resource for storing information, which is available to a computer program and is usually based on some kind of durable storage. A file is durable in the sense that it remains available for programs to use after the current program has finished...
, etc.) to find out whether the program is functioning as expected. In practice, a breakpoint consists of one or more conditions that determine when a program's execution should be interrupted.
Breakpoint conditions
The most common form of a breakpoint is the one where the program's execution is interrupted right before a programmer-specified instruction is executed. This is often referred to as an instruction breakpoint.Other kinds of conditions can also be used, such as the reading, writing, or modification of a specific location in an area of memory. This is often referred to as a conditional breakpoint, a data breakpoint, or a watchpoint.
Breakpoints can also be used to interrupt execution at a particular time, upon a keystroke etc.
Inspection tools
When a breakpoint is hit, various tools are used to inspect the state of the program or alter it. Stack traceStack trace
A stack trace is a report of the active stack frames at a certain point in time during the execution of a program.It is commonly used during interactive and post-mortem debugging...
of each thread
Thread (computer science)
In computer science, a thread of execution is the smallest unit of processing that can be scheduled by an operating system. The implementation of threads and processes differs from one operating system to another, but in most cases, a thread is contained inside a process...
may be used to see the chain of function
Subroutine
In computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....
calls that led to the paused instruction. A list of watches allows to view the values of selected variables
Variable (programming)
In computer programming, a variable is a symbolic name given to some known or unknown quantity or information, for the purpose of allowing the name to be used independently of the information it represents...
and expressions
Expression (programming)
An expression in a programming language is a combination of explicit values, constants, variables, operators, and functions that are interpreted according to the particular rules of precedence and of association for a particular programming language, which computes and then produces another value...
. There may also be tools to show the contents of registers
Processor register
In computer architecture, a processor register is a small amount of storage available as part of a CPU or other digital processor. Such registers are addressed by mechanisms other than main memory and can be accessed more quickly...
, loaded program modules and other information.
Hardware
Many processorsCentral processing unit
The central processing unit is the portion of a computer system that carries out the instructions of a computer program, to perform the basic arithmetical, logical, and input/output operations of the system. The CPU plays a role somewhat analogous to the brain in the computer. The term has been in...
include hardware
Computer hardware
Personal computer hardware are component devices which are typically installed into or peripheral to a computer case to create a personal computer upon which system software is installed including a firmware interface such as a BIOS and an operating system which supports application software that...
support for breakpoints (typically instruction and data breakpoints). As an example, the x86 instruction set architecture provides hardware support for breakpoints with its x86 debug registers. Such hardware may include limitations, for example not allowing breakpoints on instructions located in branch delay slot
Branch delay slot
In computer architecture, a delay slot is an instruction slot that gets executed without the effects of a preceding instruction. The most common form is a single arbitrary instruction located immediately after a branch instruction on a RISC or DSP architecture; this instruction will execute even if...
s. This kind of limitation is imposed by the microarchitecture
Microarchitecture
In computer engineering, microarchitecture , also called computer organization, is the way a given instruction set architecture is implemented on a processor. A given ISA may be implemented with different microarchitectures. Implementations might vary due to different goals of a given design or...
of the processor and varies from processor to processor.
Software
Without hardware support, debuggers have to implement breakpoints in software. For instruction breakpoints, this is a comparatively simple task of replacing the instruction at the location of the breakpoint by either:- an instruction that calls the debugger directly (e.g. a system callSystem callIn computing, a system call is how a program requests a service from an operating system's kernel. This may include hardware related services , creating and executing new processes, and communicating with integral kernel services...
) or - an invalid instruction that causes a deliberate program interrupt (that is then intercepted/handled by the debugger)
Alternatively,
- an instruction set simulatorInstruction Set SimulatorAn instruction set simulator is a simulation model, usually coded in a high-level programming language, which mimics the behavior of a mainframe or microprocessor by "reading" instructions and maintaining internal variables which represent the processor's registers.Instruction simulation is a...
can implement unconditional or conditional breakpoints, by simply embedding the appropriate condition tests within its own normal program cycle - that also naturally allows non-invasive breakpoints (on read-onlyRead-only memoryRead-only memory is a class of storage medium used in computers and other electronic devices. Data stored in ROM cannot be modified, or can be modified only slowly or with difficulty, so it is mainly used to distribute firmware .In its strictest sense, ROM refers only...
programs for instance). - Interpreted languageInterpreted languageInterpreted language is a programming language in which programs are 'indirectly' executed by an interpreter program. This can be contrasted with a compiled language which is converted into machine code and then 'directly' executed by the host CPU...
s can effectively use the same concept as above in their program cycle. - "Instrumenting"Instrumentation (computer programming)In context of computer programming, instrumentation refers to an ability to monitor or measure the level of a product's performance, to diagnose errors and to write trace information. Programmers implement instrumentation in the form of code instructions that monitor specific components in a system...
all the source code with additional source statements that issue a function that invoke an internal or external debug subroutine, is yet another common approach. This method increases the binaryBinary fileA binary file is a computer file which may contain any type of data, encoded in binary form for computer storage and processing purposes; for example, computer document files containing formatted text...
size and might adversely effect normal memory allocation and exception handlers. "Debug" options exist on some compilers to implement this technique semi-transparently.
Some debuggers allow program variables in memory to be modified before resuming, effectively allowing the introduction of "hand-coded" temporary assignments for test purposes. Similarly, program instructions can often be skipped to determine the effect of changes to the program logic - enabling questions about program execution to be answered in a direct way (i.e. without assumptions or guesswork). In many cases it may be the only practical method of testing obscure "event-driven" error subroutines that rarely, if ever, get executed - without the added risk of leaving temporary source changes.
Implementing data breakpoints in software however, can greatly reduce the performance of the application being debugged - since it is using additional resources on the same processor. However, this is normally acceptable during testing and the amount of information available from the debugger is not restricted by limitations of debug data known to the hardware. For instance, a software implementation can collect logical path data at program/subroutine/instruction level to considerably augment what might be stored by the particular hardware platform for inspection. The instruction set simulation method considerably reduces the compared to the (repeated) instruction replacement method, also reducing cache misses.
Some programming language implementations expose
Reflection (computer science)
In computer science, reflection is the process by which a computer program can observe and modify its own structure and behavior at runtime....
their debugging functions for use by other programs.
For example, some FORTRAN
Fortran
Fortran is a general-purpose, procedural, imperative programming language that is especially suited to numeric computation and scientific computing...
dialects have an
AT
statement, which was originally intended to act as an instruction breakpoint.Python
Python (programming language)
Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive...
implements a debugger accessible from a Python program.
These facilities can be and are abused to act like the COMEFROM
COMEFROM
In computer programming, COMEFROM is an obscure control flow structure used in some programming languages, originally as a joke....
statement.
See also
- Program Animation (Stepping)Program animationProgram animation or Stepping refers to the very common debugging method of executing code one "line" at a time. The programmer may examine the state of the program, machine, and related data before and after execution of a particular line of code...
- IBM OLIVER (CICS interactive test/debug)
- SIMON (Batch Interactive test/debug)SIMON (Batch Interactive test/debug)SIMON was a proprietary test/debugging toolkit for interactively testing Batch programs designed to run on IBM's System 360/370/390 architecture....
- SIMMONSIMMONSIMMON was a proprietary software testing system developed in the late 1960s in the IBM Product Test Laboratory, then at Poughkeepsie, N.Y...