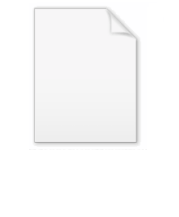
Tiny BASIC
Encyclopedia
Tiny BASIC is a dialect of the BASIC
programming language
that can fit into as little as 2 or 3 KB
of memory. This small size made it invaluable in the early days of microcomputers (the mid-1970s), when typical memory size was only 4–8 KB.
. He was urged to create the standard by Bob Albrecht of the Homebrew Computer Club
. He had seen BASIC on minicomputer
s and felt it would be the perfect match for new machines like the MITS Altair 8800
, which had been released in January 1975.
Bob and Dennis published the design document in the newsletter of the People's Computer Company
. In December 1975, Dick Whipple and John Arnold created an interpreter
for the language that required only 3K of RAM. Bob and Dennis decided to publish this version and corrections to the original design documents in a newsletter dedicated to Tiny BASIC, which they called "Dr. Dobb's Journal of Tiny BASIC Calisthenics and Orthodontia"
. In the 1976 issues several versions of Tiny BASIC, including design descriptions and full source code, were published.
(usually generated by a keyboard's "Enter" key).
line ::= number statement CR | statement CR
statement ::= PRINT expr-list
IF expression relop expression THEN statement
GOTO expression
INPUT var-list
LET var = expression
GOSUB expression
RETURN
CLEAR
LIST
RUN
END
expr-list ::= (string|expression) * )
var-list ::= var *
expression ::= (+|-|ε) term ((+|-) term)*
term ::= factor ((*|/) factor)*
factor ::= var | number | (expression)
var ::= A | B | C .... | Y | Z
number ::= digit digit*
digit ::= 0 | 1 | 2 | 3 | ... | 8 | 9
relop ::= < (>|=|ε) | > (<|=|ε) | =
A BREAK from the console will interrupt execution of the program
Source: Dr. Dobb's Journal of Computer Calisthenics & Orthodontia, Volume 1, Number 1, 1976, p.9.
(IL) is used. (At least two later versions, Palo Alto Tiny BASIC and 68000 Tiny BASIC, are direct interpreters). An interpreter
written in IL interprets a line of Tiny Basic code and executes it. The IL is run on an abstract machine
, which interprets IL code. The idea to use an interpreted language goes back to Val Schorre (with META II
, 1964) and Glennie (Syntax Machine). See also virtual machine
, CLI
.
The following table gives a partial list of the commands of the interpreted language in which the Tiny BASIC interpreter is written. The length of the whole interpreter program is only 120 IL operations. Thus the choice of an interpretive approach economized on memory space and implementation effort, although the BASIC programs run thereon were executed somewhat slowly. The CRLF in the last line symbolizes a carriage return followed by a line feed.
Source: Dr. Dobb's Journal, Volume 1, Number 1, 1976, p.12.
BASIC
BASIC is a family of general-purpose, high-level programming languages whose design philosophy emphasizes ease of use - the name is an acronym from Beginner's All-purpose Symbolic Instruction Code....
programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
that can fit into as little as 2 or 3 KB
Kilobyte
The kilobyte is a multiple of the unit byte for digital information. Although the prefix kilo- means 1000, the term kilobyte and symbol KB have historically been used to refer to either 1024 bytes or 1000 bytes, dependent upon context, in the fields of computer science and information...
of memory. This small size made it invaluable in the early days of microcomputers (the mid-1970s), when typical memory size was only 4–8 KB.
History
The language was first developed solely as a standards document, written primarily by Dennis Allison, a member of the Computer Science faculty at Stanford UniversityStanford University
The Leland Stanford Junior University, commonly referred to as Stanford University or Stanford, is a private research university on an campus located near Palo Alto, California. It is situated in the northwestern Santa Clara Valley on the San Francisco Peninsula, approximately northwest of San...
. He was urged to create the standard by Bob Albrecht of the Homebrew Computer Club
Homebrew Computer Club
The Homebrew Computer Club was an early computer hobbyist users' group in Silicon Valley, which met from March 5, 1975 to December 1986...
. He had seen BASIC on minicomputer
Minicomputer
A minicomputer is a class of multi-user computers that lies in the middle range of the computing spectrum, in between the largest multi-user systems and the smallest single-user systems...
s and felt it would be the perfect match for new machines like the MITS Altair 8800
Altair 8800
The MITS Altair 8800 was a microcomputer design from 1975 based on the Intel 8080 CPU and sold by mail order through advertisements in Popular Electronics, Radio-Electronics and other hobbyist magazines. The designers hoped to sell only a few hundred build-it-yourself kits to hobbyists, and were...
, which had been released in January 1975.
Bob and Dennis published the design document in the newsletter of the People's Computer Company
People's Computer Company
People's Computer Company was an organization, a newsletter and, later, a quasiperiodical called the "dragonsmoke." PCC was founded and produced by Bob Albrecht & George Firedrake in Menlo Park, California in the early 1970s.The first newsletter announced itself with the following...
. In December 1975, Dick Whipple and John Arnold created an interpreter
Interpreter (computing)
In computer science, an interpreter normally means a computer program that executes, i.e. performs, instructions written in a programming language...
for the language that required only 3K of RAM. Bob and Dennis decided to publish this version and corrections to the original design documents in a newsletter dedicated to Tiny BASIC, which they called "Dr. Dobb's Journal of Tiny BASIC Calisthenics and Orthodontia"
Dr. Dobb's Journal
Dr. Dobb's Journal was a monthly journal published in the United States by CMP Technology. It covered topics aimed at computer programmers. DDJ was the first regular periodical focused on microcomputer software, rather than hardware. It later became a monthly section within the periodical...
. In the 1976 issues several versions of Tiny BASIC, including design descriptions and full source code, were published.
Tiny BASIC grammar
The grammar is listed below in Backus-Naur form. In the listing, an asterisk ("*") denotes zero or more of the object to its left — except for the first asterisk in the definition of "term", which is the multiplication operator; parentheses group objects; and an epsilon ("ε") signifies the empty set. As is common in computer language grammar notation, the vertical bar ("|") distinguishes alternatives, as does their being listed on separate lines. The symbol "CR" denotes a carriage returnCarriage return
Carriage return, often shortened to return, refers to a control character or mechanism used to start a new line of text.Originally, the term "carriage return" referred to a mechanism or lever on a typewriter...
(usually generated by a keyboard's "Enter" key).
line ::= number statement CR | statement CR
statement ::= PRINT expr-list
IF expression relop expression THEN statement
GOTO expression
INPUT var-list
LET var = expression
GOSUB expression
RETURN
CLEAR
LIST
RUN
END
expr-list ::= (string|expression) * )
var-list ::= var *
expression ::= (+|-|ε) term ((+|-) term)*
term ::= factor ((*|/) factor)*
factor ::= var | number | (expression)
var ::= A | B | C .... | Y | Z
number ::= digit digit*
digit ::= 0 | 1 | 2 | 3 | ... | 8 | 9
relop ::= < (>|=|ε) | > (<|=|ε) | =
A BREAK from the console will interrupt execution of the program
Source: Dr. Dobb's Journal of Computer Calisthenics & Orthodontia, Volume 1, Number 1, 1976, p.9.
Implementation in interpreted language
For some implementations, an interpreted languageInterpreted language
Interpreted language is a programming language in which programs are 'indirectly' executed by an interpreter program. This can be contrasted with a compiled language which is converted into machine code and then 'directly' executed by the host CPU...
(IL) is used. (At least two later versions, Palo Alto Tiny BASIC and 68000 Tiny BASIC, are direct interpreters). An interpreter
Interpreter (computing)
In computer science, an interpreter normally means a computer program that executes, i.e. performs, instructions written in a programming language...
written in IL interprets a line of Tiny Basic code and executes it. The IL is run on an abstract machine
Abstract machine
An abstract machine, also called an abstract computer, is a theoretical model of a computer hardware or software system used in automata theory...
, which interprets IL code. The idea to use an interpreted language goes back to Val Schorre (with META II
META II
META II is a compiler writing language first released in 1962 by D. V. Schorre. It consists of syntax equations resembling Backus normal form and into which instructions to output assembly language commands are inserted. Compilers have been written in this language for VALGOL I and VALGOL II...
, 1964) and Glennie (Syntax Machine). See also virtual machine
Virtual machine
A virtual machine is a "completely isolated guest operating system installation within a normal host operating system". Modern virtual machines are implemented with either software emulation or hardware virtualization or both together.-VM Definitions:A virtual machine is a software...
, CLI
Common Language Infrastructure
The Common Language Infrastructure is an open specification developed by Microsoft and standardized by ISO and ECMA that describes the executable code and runtime environment that form the core of the Microsoft .NET Framework and the free and open source implementations Mono and Portable.NET...
.
The following table gives a partial list of the commands of the interpreted language in which the Tiny BASIC interpreter is written. The length of the whole interpreter program is only 120 IL operations. Thus the choice of an interpretive approach economized on memory space and implementation effort, although the BASIC programs run thereon were executed somewhat slowly. The CRLF in the last line symbolizes a carriage return followed by a line feed.
TST lbl, string | If string matches the BASIC line, advance cursor over string and execute the next IL instruction; if the test fails, execute the IL instruction at the label lbl |
CALL lbl | Execute the IL subroutine starting at lbl; save the IL address following the CALL on the control stack |
RTN | Return to the IL location specified at the top of the control stack |
DONE | Report a syntax error if after deleting leading blanks the cursor is not positioned to reach a carriage return |
JUMP lbl | Continue execution of the IL at the label specified |
PRS | Print characters from the BASIC text up to but not including the closing quotation mark |
PRN | Print number obtained by popping the top of the expression stack |
SPC | Insert spaces to move the print head to next zone |
NLINE | Output a CRLF to the printer |
Source: Dr. Dobb's Journal, Volume 1, Number 1, 1976, p.12.
External links
- Robert Uiterwyk's MICRO BASIC – A MC6800 tiny BASIC later sold with the SWTPCSWTPCThe U.S. company SWTPC started in 1964 as DEMCO . It was incorporated in 1967 as Southwest Technical Products Corporation of San Antonio, Texas...
6800 computer - MINOL – Erik Mueller's MINOL – Tiny BASIC with strings for Intel 8080
- TBL – A similar IL to Tiny BASIC's, used in the first successful compilers for PL/I, Fortran, etc., on limited memory minicomputerMinicomputerA minicomputer is a class of multi-user computers that lies in the middle range of the computing spectrum, in between the largest multi-user systems and the smallest single-user systems...
s - 68000 Tiny BASIC – Based on Li-Chen WangLi-Chen WangDr. Li-Chen Wang is an American computer engineer, best known for his Palo Alto Tiny BASIC for Intel 8080-based microcomputers.This was the fourth version of Tiny BASIC that appeared in Dr. Dobb's Journal of Computer Calisthenics & Orthodontia, but probably the most influential. It appeared in the...
's Palo Alto Tiny BASIC, and published in the 100th edition of Dr. Dobb's - Tiny BASIC – An implementation written in SmallBASICSmallBASICSmallBASIC is a BASIC programming language dialect with interpreters released as free software under the GNU General Public License version 2.-Description:...
- tinyBasic – An implementation written in iziBasic
- Tiny BASIC – A live web version, ported to Run BASIC from iziBasic
- TinyBasic – A port of Tom Pittman's TinyBasic C interpreter to JavaJava (programming language)Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
, C# and Adobe FlexAdobe FlexAdobe Flex is a software development kit released by Adobe Systems for the development and deployment of cross-platform rich Internet applications based on the Adobe Flash platform...
. Includes live web versions. - TinyBasic – A modern implementation on a 16bit Microcontroller.