.gif)
Redirection (Unix)
Encyclopedia
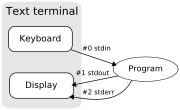
Computing
Computing is usually defined as the activity of using and improving computer hardware and software. It is the computer-specific part of information technology...
, redirection is a function common to most command-line interpreters, including the various Unix shell
Unix shell
A Unix shell is a command-line interpreter or shell that provides a traditional user interface for the Unix operating system and for Unix-like systems...
s that can redirect standard streams
Standard streams
In Unix and Unix-like operating systems , as well as certain programming language interfaces, the standard streams are preconnected input and output channels between a computer program and its environment when it begins execution...
to user-specified locations.
In unix-like operating systems programs do redirection with the dup2(2) system call
System call
In computing, a system call is how a program requests a service from an operating system's kernel. This may include hardware related services , creating and executing new processes, and communicating with integral kernel services...
, or its less-flexible but higher-level stdio
Standard streams
In Unix and Unix-like operating systems , as well as certain programming language interfaces, the standard streams are preconnected input and output channels between a computer program and its environment when it begins execution...
analogues, freopen(3) and popen(3).
Redirecting standard input and standard output
Redirection is usually implemented by placing certain characters between commandsCommand (computing)
In computing, a command is a directive to a computer program acting as an interpreter of some kind, in order to perform a specific task. Most commonly a command is a directive to some kind of command line interface, such as a shell....
. Typically, the syntax
Syntax
In linguistics, syntax is the study of the principles and rules for constructing phrases and sentences in natural languages....
of these characters is as follows:
command1 > file1
executes command1, placing the output in file1. This will clobber
Clobber
Clobber is an abstract strategy game invented in 2001 by combinatorial game theorists Michael H. Albert, J.P. Grossman and Richard Nowakowski. It has subsequently been studied by Elwyn Berlekamp and Erik Demaine among others...
any existing data in file1 (.
To append output to the end of the file, use the >> operator:
command1 >> file1
command1 < file1
executes command1, using file1 as the source of input (as opposed to the keyboard
Computer keyboard
In computing, a keyboard is a typewriter-style keyboard, which uses an arrangement of buttons or keys, to act as mechanical levers or electronic switches...
).
command1 < infile > outfile
combines the two capabilities: command1 reads from infile and writes to outfile
Piping
Programs can be run together such that one program reads the output from another with no need for an explicit intermediate file: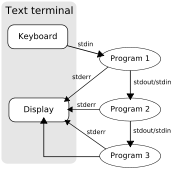
command1 | command2
executes command1, using its output as the input for command2 (commonly called piping
Pipeline (Unix)
In Unix-like computer operating systems , a pipeline is the original software pipeline: a set of processes chained by their standard streams, so that the output of each process feeds directly as input to the next one. Each connection is implemented by an anonymous pipe...
, with the "|" character being known as "pipe").
This is similar to using two redirects and a temporary file:
command1 > tempfile
command2 < tempfile
rm tempfile
A good example for command piping is combining
echo
with another command to achieve something interactive in a non-interactive shell, e.g. echo -e "user\npass" | ftp localhost
This runs the ftp
File Transfer Protocol
File Transfer Protocol is a standard network protocol used to transfer files from one host to another host over a TCP-based network, such as the Internet. FTP is built on a client-server architecture and utilizes separate control and data connections between the client and server...
client with input user, press return, then pass.
Redirecting to and from the standard file handles
In Unix shellUnix shell
A Unix shell is a command-line interpreter or shell that provides a traditional user interface for the Unix operating system and for Unix-like systems...
s derived from the original Bourne shell
Bourne shell
The Bourne shell, or sh, was the default Unix shell of Unix Version 7 and most Unix-like systems continue to have /bin/sh - which will be the Bourne shell, or a symbolic link or hard link to a compatible shell - even when more modern shells are used by most users.Developed by Stephen Bourne at AT&T...
, the first two actions can be further modified by placing a number (the file descriptor
File descriptor
In computer programming, a file descriptor is an abstract indicator for accessing a file. The term is generally used in POSIX operating systems...
) immediately before the character; this will affect which stream is used for the redirection. The Unix standard I/O streams are:
Handle | Name | Description |
---|---|---|
0 | stdin | Standard input |
1 | stdout | Standard output |
2 | stderr | Standard error |
For example:
command1 2> file1
executes command1, directing the standard error stream to file1.
In shells derived from csh
C shell
The C shell is a Unix shell that was created by Bill Joy while a graduate student at University of California, Berkeley in the late 1970s. It has been distributed widely, beginning with the 2BSD release of the BSD Unix system that Joy began distributing in 1978...
(the C shell
C shell
The C shell is a Unix shell that was created by Bill Joy while a graduate student at University of California, Berkeley in the late 1970s. It has been distributed widely, beginning with the 2BSD release of the BSD Unix system that Joy began distributing in 1978...
), the syntax instead appends the & character to the redirect characters, thus achieving a similar result.
Another useful capability is to redirect one standard file handle to another. The most popular variation is to merge standard error into standard output so error messages can be processed together with (or alternately to) the usual output. Example:
find / -name .profile > results 2>&1
will try to find all files named .profile. Executed without redirection, it will output hits to stdout and errors (e.g. for lack of privilege to traverse protected directories) to stderr. If standard output is directed to file results, error messages appear on the console. To see both hits and error messages in file results, merge stderr (handle 2) into stdout (handle 1) using 2>&1 .
If the merged output is to be piped into another program, the file merge sequence 2>&1 must precede the pipe symbol, thus:
find / -name .profile 2>&1 | less
A simplified but non-POSIX conforming form of the command:
command > file 2>&1
is (not available in Bourne Shell prior to version 4, final release, or in the standard shell Debian Almquist shell
Debian Almquist shell
The Debian Almquist shell is a Unix shell, much smaller than bash but still aiming at POSIX-compliancy. It requires less disk space but is also less feature-rich.- History :...
used in Debian/Ubuntu):
command &>file
or:
command >&file
NOTE: It is possible use
2>&1
before ">
" but the result is commonly misunderstood.The rule is that any redirection sets the handle to the output stream independently.
So "
2>&1
" sets handle 2
to whatever handle 1
points to, which at that point usually is stdout.Then "
>
" redirects handle 1
to something else, e.g. a file, but it does not change handle 2
., still pointing to stdout.In the following example. standard output is written to file, but errors are redirected from stderr to stdout, i.e. sent to the screen.
command 2>&1 > file
Chained pipelines
The redirection and piping tokens can be chained together to create complex commands. For example:ls | grep '\.sh' | sort > shlist
lists the contents of the current directory, where this output is filtered to only contain lines which contain .sh, sort this resultant output lexicographically, and place the final output in shlist. This type of construction is used very commonly in shell script
Shell script
A shell script is a script written for the shell, or command line interpreter, of an operating system. It is often considered a simple domain-specific programming language...
s and batch file
Batch file
In DOS, OS/2, and Microsoft Windows, batch file is the name given to a type of script file, a text file containing a series of commands to be executed by the command interpreter....
s.
Redirect to multiple outputs
The standard command tee can redirect output from a command to several destinations.ls -lrt | tee xyz
This directs the file list output to both standard output and the file xyz.
See also
- Here-document, a way of specifying text for input in command line shells
- Shell shovelingShell shovelingShell shoveling, in network security, refers to the act of redirecting the input and output of a shell to a service so that it can be remotely accessed....
External links
- Redirection Definition by The Linux Information Project (LINFO)
- I/O Redirection in The Linux Documentation Project
- Redirection in Windows
- Creating a Child Process with Redirected Input and Output in Windows