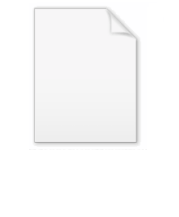
Function prologue
Encyclopedia
In assembly language
programming
, the function prologue is a few lines of code at the beginning of a function, which prepare the stack
and registers
for use within the function. Similarly, the function epilogue appears at the end of the function, and restores the stack and registers to the state they were in before the function was called.
The prologue and epilogue are not a part of the assembly language itself; they represent a convention used by assembly language programmer
s, and compiler
s of many higher-level languages
. They are fairly rigid, having the same form in each function.
Several possible prologues can be written, resulting in slightly different stack configuration. These differences are acceptable, as long as the programmer or compiler uses the stack in the correct way inside the function.
For example, these three steps may be accomplished in 32-bit x86 assembly language
by the following instructions (using AT&T syntax):
Where n is the size of the local variables, in byte
s, and l means long, which occupies 4 byte
s. The above sequence is typical of the output produced by the GCC
compiler.
The x86 uses a slightly different prologue; it can be called with the
More complex prologues can be obtained using different values (other than 0) for the second operand of the
s, as required by languages such as Pascal. Modern versions of these languages however don't use these instructions because they limit the procedure-nesting depth in some cases.
The given epilogue will reverse the effects of either of the above prologues (either the full one, or the one which uses
For example, these three steps may be accomplished in 32-bit x86 assembly language
by the following instructions (using AT&T syntax):
Like the prologue, the x86 processor contains a built-in instruction which performs part of the epilogue. The following code is equivalent to the above code:
The
A function may contain multiple epilogues. Every function exit point must either jump to a common epilogue at the end, or contain its own epilogue. Therefore, programmers or compilers often use the combination of
compiler would substitute a
Assembly language
An assembly language is a low-level programming language for computers, microprocessors, microcontrollers, and other programmable devices. It implements a symbolic representation of the machine codes and other constants needed to program a given CPU architecture...
programming
Computer programming
Computer programming is the process of designing, writing, testing, debugging, and maintaining the source code of computer programs. This source code is written in one or more programming languages. The purpose of programming is to create a program that performs specific operations or exhibits a...
, the function prologue is a few lines of code at the beginning of a function, which prepare the stack
Call stack
In computer science, a call stack is a stack data structure that stores information about the active subroutines of a computer program. This kind of stack is also known as an execution stack, control stack, run-time stack, or machine stack, and is often shortened to just "the stack"...
and registers
Processor register
In computer architecture, a processor register is a small amount of storage available as part of a CPU or other digital processor. Such registers are addressed by mechanisms other than main memory and can be accessed more quickly...
for use within the function. Similarly, the function epilogue appears at the end of the function, and restores the stack and registers to the state they were in before the function was called.
The prologue and epilogue are not a part of the assembly language itself; they represent a convention used by assembly language programmer
Programmer
A programmer, computer programmer or coder is someone who writes computer software. The term computer programmer can refer to a specialist in one area of computer programming or to a generalist who writes code for many kinds of software. One who practices or professes a formal approach to...
s, and compiler
Compiler
A compiler is a computer program that transforms source code written in a programming language into another computer language...
s of many higher-level languages
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
. They are fairly rigid, having the same form in each function.
Prologue
A function prologue typically does the following actions if the architecture has a base pointer (also known as frame pointer) and a stack pointer (the following actions may not be applicable to those architectures that are missing a base pointer or stack pointer) :- Pushes the old base pointer onto the stack, such that it can be restored later (by getting the new base pointer value which is set in the next step and is always pointed to this location).
- Assigns the value of stack pointer (which is pointed to the saved base pointer and the top of the old stack frame) into base pointer such that a new stack frame will be created on top of the old stack frame (i.e. the top of the old stack frame will became the base of the new stack frame).
- Moves the stack pointer further (either decreasing or increasing its value, depends on whether the stack grow down or up. On x86 and x64 platform, its value get decreased) to make room for the variables (i.e. memory allocation for function's local variable)
Several possible prologues can be written, resulting in slightly different stack configuration. These differences are acceptable, as long as the programmer or compiler uses the stack in the correct way inside the function.
For example, these three steps may be accomplished in 32-bit x86 assembly language
X86 assembly language
x86 assembly language is a family of backward-compatible assembly languages, which provide some level of compatibility all the way back to the Intel 8008. x86 assembly languages are used to produce object code for the x86 class of processors, which includes Intel's Core series and AMD's Phenom and...
by the following instructions (using AT&T syntax):
- pushl %ebp
- movl %esp, %ebp
- subl $n, %esp
Where n is the size of the local variables, in byte
Byte
The byte is a unit of digital information in computing and telecommunications that most commonly consists of eight bits. Historically, a byte was the number of bits used to encode a single character of text in a computer and for this reason it is the basic addressable element in many computer...
s, and l means long, which occupies 4 byte
Byte
The byte is a unit of digital information in computing and telecommunications that most commonly consists of eight bits. Historically, a byte was the number of bits used to encode a single character of text in a computer and for this reason it is the basic addressable element in many computer...
s. The above sequence is typical of the output produced by the GCC
GNU Compiler Collection
The GNU Compiler Collection is a compiler system produced by the GNU Project supporting various programming languages. GCC is a key component of the GNU toolchain...
compiler.
The x86 uses a slightly different prologue; it can be called with the
enter
instruction:
- enter $n, $0
More complex prologues can be obtained using different values (other than 0) for the second operand of the
enter
instruction. These prologues push several base/frame pointers to allow for nested functionNested function
In computer programming, a nested function is a function which is lexically encapsulated within another function. It can only be called by the enclosing function or by functions directly or indirectly nested within the same enclosing function. In other words, the scope of the nested function is...
s, as required by languages such as Pascal. Modern versions of these languages however don't use these instructions because they limit the procedure-nesting depth in some cases.
Epilogue
Function epilogue reverses the actions of the function prologue and returns control to the calling function. It typically does the following actions (this procedure may differ from one architecture to another):- Replaces the stack pointer with the current base (or frame) pointer, so the stack pointer is restored to its value before the prologue
- Pops the base pointer off the stack, so it is restored to its value before the prologue
- Returns to the calling function, by popping the previous frame's program counter off the stack and jumping to it
The given epilogue will reverse the effects of either of the above prologues (either the full one, or the one which uses
enter
).For example, these three steps may be accomplished in 32-bit x86 assembly language
X86 assembly language
x86 assembly language is a family of backward-compatible assembly languages, which provide some level of compatibility all the way back to the Intel 8008. x86 assembly languages are used to produce object code for the x86 class of processors, which includes Intel's Core series and AMD's Phenom and...
by the following instructions (using AT&T syntax):
- mov %ebp, %esp
- pop %ebp
- ret
Like the prologue, the x86 processor contains a built-in instruction which performs part of the epilogue. The following code is equivalent to the above code:
- leave
- ret
The
leave
instruction performs the mov
and pop
instructions, as outlined above.A function may contain multiple epilogues. Every function exit point must either jump to a common epilogue at the end, or contain its own epilogue. Therefore, programmers or compilers often use the combination of
leave
and ret
to exit the function at any point. (For example, a CC (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
compiler would substitute a
return
statement with a leave
/ret
sequence).