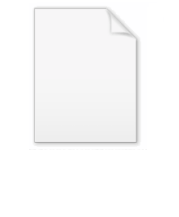
Ellipsis (programming operator)
Encyclopedia
In programming
In programming, ellipsis notation (.. or ...) is mostly used for two usages: Either to denote ranges or to denote a variable or unspecified number of arguments.Most programming languages other than Perl6 require the ellipsis to be written as a series of periods; a single (Unicode) ellipsis character cannot be used.
Ranges
In some programming languageProgramming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
s (including Perl
Perl
Perl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular...
, Ruby
Ruby (programming language)
Ruby is a dynamic, reflective, general-purpose object-oriented programming language that combines syntax inspired by Perl with Smalltalk-like features. Ruby originated in Japan during the mid-1990s and was first developed and designed by Yukihiro "Matz" Matsumoto...
, Groovy, Haskell
Haskell (programming language)
Haskell is a standardized, general-purpose purely functional programming language, with non-strict semantics and strong static typing. It is named after logician Haskell Curry. In Haskell, "a function is a first-class citizen" of the programming language. As a functional programming language, the...
, and Pascal
Pascal (programming language)
Pascal is an influential imperative and procedural programming language, designed in 1968/9 and published in 1970 by Niklaus Wirth as a small and efficient language intended to encourage good programming practices using structured programming and data structuring.A derivative known as Object Pascal...
), a shortened two-dot ellipsis is used to represent a range of values given two endpoints; for example, to iterate through a list of integer
Integer
The integers are formed by the natural numbers together with the negatives of the non-zero natural numbers .They are known as Positive and Negative Integers respectively...
s between 1 and 100 inclusive in Perl:
foreach (1..100)
In Perl and Ruby, the
...
operator denotes a half-open range, i.e. that includes the start value but not the end value.Perl overloads
Operator overloading
In object oriented computer programming, operator overloading—less commonly known as operator ad-hoc polymorphism—is a specific case of polymorphism, where different operators have different implementations depending on their arguments...
the ".." operator in scalar context as a stateful bistable
Bistability
Bistability is a fundamental phenomenon in nature. Something that is bistable can be resting in either of two states. These rest states need not be symmetric with respect to stored energy...
Boolean
Boolean datatype
In computer science, the Boolean or logical data type is a data type, having two values , intended to represent the truth values of logic and Boolean algebra...
test, roughly equivalent to "true while x but not yet y". In Perl6, the 3-character ellipsis is also known as the "yadda yadda yadda" operator and, similarly to its linguistic meaning
Linguistic meaning
The nature of meaning, its definition, elements, and types, was discussed by philosophers Aristotle, Augustine, and Aquinas. According to them 'meaning is a relationship between two sorts of things: signs and the kinds of things they mean '. One term in the relationship of meaning necessarily...
, serves as a "stand-in" for code to be inserted later. In addition, an actual Unicode
Unicode
Unicode is a computing industry standard for the consistent encoding, representation and handling of text expressed in most of the world's writing systems...
ellipsis character is used to serve as a type of marker in a perl6 format string.
The GNU Compiler Collection
GNU Compiler Collection
The GNU Compiler Collection is a compiler system produced by the GNU Project supporting various programming languages. GCC is a key component of the GNU toolchain...
has an extension to the C and C++ language to allow case ranges in switch statement
Switch statement
In computer programming, a switch, case, select or inspect statement is a type of selection control mechanism that exists in most imperative programming languages such as Pascal, Ada, C/C++, C#, Java, and so on. It is also included in several other types of languages...
s:
switch(u) {
case 0 ... 0x7F : putchar(c); break;
case 0x80 ... 0x7FF : putchar(0xC0 + c>>6); putchar( 0x80 + c&0x3f); break;
case 0x800 ... 0xFFFF : putchar(0xE0 + c>>12); putchar( 0x80 + (c>>6)&0x3f); putchar( 0x80 + (c>>12) ); break;
default: error("not supported!");
}
Variable number of parameters
In the C programming languageC (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
, an ellipsis is used to represent a variable number of parameters
Variadic function
In computer programming, a variadic function is a function of indefinite arity, i.e., one which accepts a variable number of arguments. Support for variadic functions differs widely among programming languages....
to a function. For example:
void func(const char* str, ...)
The above function in C could then be called with different types and numbers of parameters such as:
func("input string", 5, 10, 15);
and
func("input string", "another string", 0.5);
As of version 1.5, Java
Java (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
has adopted this "varargs" functionality. For example:
public int func(int num, String... strings)
C99
C99
C99 is a modern dialect of the C programming language. It extends the previous version with new linguistic and library features, and helps implementations make better use of available computer hardware and compiler technology.-History:...
introduced macros with a variable number of arguments
Variadic macro
A variadic macro is a feature of the C preprocessor whereby a macro may be declared to accept a varying number of arguments.Variable-argument macros were introduced in the ISO/IEC 9899:1999 revision of the C programming language standard in 1999...
.
C++0x
C++0x
C++11, also formerly known as C++0x, is the name of the most recent iteration of the C++ programming language, replacing C++03, approved by the ISO as of 12 August 2011...
introduces templates with a variable number of arguments.
Multiple dimensions
In PythonPython (programming language)
Python is a general-purpose, high-level programming language whose design philosophy emphasizes code readability. Python claims to "[combine] remarkable power with very clear syntax", and its standard library is large and comprehensive...
, particularly in numpy, an ellipsis is used for slicing an arbitrary number of dimensions for a high-dimensional array:
>>> import numpy as np
>>> t = np.random.rand(2, 3, 4, 5)
>>> t[..., 0].shape # select 1st element from last dimension, copy rest
(2, 3, 4)
>>> t[0, ...].shape # select 1st element from first dimension, copy rest
(3, 4, 5)
Other semantics
In MATLABMATLAB
MATLAB is a numerical computing environment and fourth-generation programming language. Developed by MathWorks, MATLAB allows matrix manipulations, plotting of functions and data, implementation of algorithms, creation of user interfaces, and interfacing with programs written in other languages,...
, a three-character ellipsis is used to indicate line continuation, making the sequence of lines
x = [ 1 2 3 ...
4 5 6 ];
semantically equivalent to the single line
x = [ 1 2 3 4 5 6 ];
In the Unified Modeling Language
Unified Modeling Language
Unified Modeling Language is a standardized general-purpose modeling language in the field of object-oriented software engineering. The standard is managed, and was created, by the Object Management Group...
(UML), a two-character ellipsis is used to indicate variable cardinality of an association. For example, a cardinality of 1..* means that the number of elements aggregated in an association can range from 1 to infinity (a usage equivalent to Kleene plus).