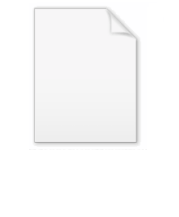
Class-based programming
Encyclopedia
Class-based programming, or more commonly class-orientation, refers to the style of object-oriented programming
in which inheritance is achieved by defining classes of objects, as opposed to the objects themselves (compare Prototype-based programming
).
The most popular and developed model of OOP is a class-based model, as opposed to an object-based model. In this model, objects are entities that combine state
(i.e., data), behavior
(i.e., procedures, or method
s) and identity
(unique existence among all other objects). The structure and behavior of an object are defined by a class, which is a definition
, or blueprint
, of all objects of a specific type. An object must be explicitly created based on a class and an object thus created is considered to be an instance of that class. An object is similar to a structure
, with the addition of method pointers, member access control, and an implicit data member which locates instances of the class (i.e. actual objects of that class) in the class hierarchy (essential for runtime inheritance features).
prevents users from breaking the invariants
of the class, which is useful because it allows the implementation of a class of objects to be changed for aspects not exposed in the interface without impact to user code. The definitions of encapsulation focus on the grouping and packaging of related information (cohesion
) rather than security issues. OOP languages do not normally offer formal security restrictions to the internal object state. Using a method of access is a matter of convention for the interface design.
Inheritance
is typically done by grouping objects into classes, and defining class
es as extension
s of existing classes, and thus grouping classes into trees or lattices reflecting behavioral commonality. Although the use of classes is the most popular technique for inheritance, another well-known technique is Prototype-based programming
.
, does not hold. Therefore normally one must distinguish subtyping and subclassing. Most current object-oriented languages distinguish subtyping and subclassing, however some approaches to design do not.
Also, another common example is that a person object created from a child class cannot become an object of parent class because a child class and a parent class inherit a person class but class-based languages mostly do not allow to change the kind of class of the object at runtime. For class-based languages, this restriction is essential in order to preserve unified view of the class to its users. The users should not need to care whether one of the implementations of a method happens to cause changes that break the invariants
of the class. Such changes can be made by destroying the object and constructing another in its place. Polymorphism can be used to preserve the relevant interfaces even when such changes are done, because the objects are viewed as black box abstractions and accessed via object identity
. However, usually the value of object references referring to the object is changed, which causes effects to client code.
introduced the class abstraction, the canonical example of a class-based language is Smalltalk
. Others include C++
, Java
and C#.
Object-oriented programming
Object-oriented programming is a programming paradigm using "objects" – data structures consisting of data fields and methods together with their interactions – to design applications and computer programs. Programming techniques may include features such as data abstraction,...
in which inheritance is achieved by defining classes of objects, as opposed to the objects themselves (compare Prototype-based programming
Prototype-based programming
Prototype-based programming is a style of object-oriented programming in which classes are not present, and behavior reuse is performed via a process of cloning existing objects that serve as prototypes. This model can also be known as classless, prototype-oriented or instance-based programming...
).
The most popular and developed model of OOP is a class-based model, as opposed to an object-based model. In this model, objects are entities that combine state
State (computer science)
In computer science and automata theory, a state is a unique configuration of information in a program or machine. It is a concept that occasionally extends into some forms of systems programming such as lexers and parsers....
(i.e., data), behavior
Behavior
Behavior or behaviour refers to the actions and mannerisms made by organisms, systems, or artificial entities in conjunction with its environment, which includes the other systems or organisms around as well as the physical environment...
(i.e., procedures, or method
Method (computer science)
In object-oriented programming, a method is a subroutine associated with a class. Methods define the behavior to be exhibited by instances of the associated class at program run time...
s) and identity
Identity (object-oriented programming)
An identity in object-oriented programming, object-oriented design and object-oriented analysis describes the property of objects that distinguishes them from other objects. This is closely related to the philosophical concept of identity....
(unique existence among all other objects). The structure and behavior of an object are defined by a class, which is a definition
Definition
A definition is a passage that explains the meaning of a term , or a type of thing. The term to be defined is the definiendum. A term may have many different senses or meanings...
, or blueprint
Blueprint
A blueprint is a type of paper-based reproduction usually of a technical drawing, documenting an architecture or an engineering design. More generally, the term "blueprint" has come to be used to refer to any detailed plan....
, of all objects of a specific type. An object must be explicitly created based on a class and an object thus created is considered to be an instance of that class. An object is similar to a structure
Data structure
In computer science, a data structure is a particular way of storing and organizing data in a computer so that it can be used efficiently.Different kinds of data structures are suited to different kinds of applications, and some are highly specialized to specific tasks...
, with the addition of method pointers, member access control, and an implicit data member which locates instances of the class (i.e. actual objects of that class) in the class hierarchy (essential for runtime inheritance features).
Encapsulation
EncapsulationInformation hiding
In computer science, information hiding is the principle of segregation of the design decisions in a computer program that are most likely to change, thus protecting other parts of the program from extensive modification if the design decision is changed...
prevents users from breaking the invariants
Invariant (computer science)
In computer science, a predicate is called an invariant to a sequence of operations provided that: if the predicate is true before starting the sequence, then it is true at the end of the sequence.-Use:...
of the class, which is useful because it allows the implementation of a class of objects to be changed for aspects not exposed in the interface without impact to user code. The definitions of encapsulation focus on the grouping and packaging of related information (cohesion
Cohesion (computer science)
In computer programming, cohesion is a measure of how strongly-related each piece of functionality expressed by the source code of a software module is...
) rather than security issues. OOP languages do not normally offer formal security restrictions to the internal object state. Using a method of access is a matter of convention for the interface design.
Inheritance
- See InheritanceInheritance (object-oriented programming)In object-oriented programming , inheritance is a way to reuse code of existing objects, establish a subtype from an existing object, or both, depending upon programming language support...
Inheritance
Inheritance (computer science)
In object-oriented programming , inheritance is a way to reuse code of existing objects, establish a subtype from an existing object, or both, depending upon programming language support...
is typically done by grouping objects into classes, and defining class
Class (computer science)
In object-oriented programming, a class is a construct that is used as a blueprint to create instances of itself – referred to as class instances, class objects, instance objects or simply objects. A class defines constituent members which enable these class instances to have state and behavior...
es as extension
Extension (semantics)
In any of several studies that treat the use of signs - for example, in linguistics, logic, mathematics, semantics, and semiotics - the extension of a concept, idea, or sign consists of the things to which it applies, in contrast with its comprehension or intension, which consists very roughly of...
s of existing classes, and thus grouping classes into trees or lattices reflecting behavioral commonality. Although the use of classes is the most popular technique for inheritance, another well-known technique is Prototype-based programming
Prototype-based programming
Prototype-based programming is a style of object-oriented programming in which classes are not present, and behavior reuse is performed via a process of cloning existing objects that serve as prototypes. This model can also be known as classless, prototype-oriented or instance-based programming...
.
Critique of class-based models
Class-based languages, or, to be more precise, typed languages, where subclassing is the only way of subtyping, have been criticized for mixing up implementations and interfaces—the essential principle in object-oriented programming. The critics say one might create a bag class that stores a collection of objects, then extends it to make a new class called a set class where the duplication of objects is eliminated. Now, a function that takes a bag class may expect that adding two objects increases the size of a bag by two, yet if one passes an object of a set class, then adding two objects may or may not increase the size of a bag by two. The problem arises precisely because subclassing implies subtyping even in the instances where the principle of subtyping, known as the Liskov substitution principleLiskov substitution principle
Substitutability is a principle in object-oriented programming. It states that, in a computer program, if S is a subtype of T, then objects of type T may be replaced with objects of type S without altering any of the desirable properties of that program...
, does not hold. Therefore normally one must distinguish subtyping and subclassing. Most current object-oriented languages distinguish subtyping and subclassing, however some approaches to design do not.
Also, another common example is that a person object created from a child class cannot become an object of parent class because a child class and a parent class inherit a person class but class-based languages mostly do not allow to change the kind of class of the object at runtime. For class-based languages, this restriction is essential in order to preserve unified view of the class to its users. The users should not need to care whether one of the implementations of a method happens to cause changes that break the invariants
Invariant (computer science)
In computer science, a predicate is called an invariant to a sequence of operations provided that: if the predicate is true before starting the sequence, then it is true at the end of the sequence.-Use:...
of the class. Such changes can be made by destroying the object and constructing another in its place. Polymorphism can be used to preserve the relevant interfaces even when such changes are done, because the objects are viewed as black box abstractions and accessed via object identity
Identity (object-oriented programming)
An identity in object-oriented programming, object-oriented design and object-oriented analysis describes the property of objects that distinguishes them from other objects. This is closely related to the philosophical concept of identity....
. However, usually the value of object references referring to the object is changed, which causes effects to client code.
Example languages
Although SimulaSimula
Simula is a name for two programming languages, Simula I and Simula 67, developed in the 1960s at the Norwegian Computing Center in Oslo, by Ole-Johan Dahl and Kristen Nygaard...
introduced the class abstraction, the canonical example of a class-based language is Smalltalk
Smalltalk
Smalltalk is an object-oriented, dynamically typed, reflective programming language. Smalltalk was created as the language to underpin the "new world" of computing exemplified by "human–computer symbiosis." It was designed and created in part for educational use, more so for constructionist...
. Others include C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
, Java
Java (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
and C#.