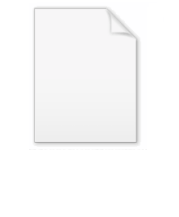
Undefined value
Encyclopedia
In computing undefined value
is a condition where an expression
has not a correct value, although it is syntactically correct. Undefined value may not be confused with empty string
, boolean "false" or other "empty" (but defined) values. Depending on circumstances, evaluation to undefined value may lead to exception or undefined behaviour
, but in some programming language
s undefined values can occur during a normal, predictable course of program execution.
Dynamically typed languages usually treat undefined values explicitly (when possible), e.g. Perl
has
is undefined, when its argument is out of domain of definition. This include numerous arithmetic
al cases such as division by zero
, square root
or logarithm
of a negative number etc.; see NaN
.
Even some mathematically well-defined expressions like exp
(100000
) may be undefined in floating point
arithmetic because exceed its limits. If implementation supports ±∞
, then this value may be computed as +∞ (inf), however.
Element of an array is undefined when index is out of bounds, as a lookup in an associative array
for a key which it does not contain.
An argument of variadic function
, which was not passed to it, is undefined when function is called.
A variable
which is not initialized, has undefined (or unpredictable) value until it was assigned.
Dereference of null pointer lead to undefined value and usually rises an exception immediately.
Any expression of the bottom type
is undefined by definition, because that type has no values.
The value of a function which loops forever
(for example, in the case of failed μ operator in a partial recursive function) may be seen as undefined too, but only of a theoretical interest because such function never returns.
In such statically typed
languages as C
(C++) there is no specific notion of a value undefined at runtime. Arithmetically undefined expressions invoke exceptions and crash the program, if uncaught
. Undefined (means, unpredictable) data in C and similar languages may appear in case of flawy designed program or unexpected fault, and represent a severe danger, particularly pointers to deallocated memory
and null pointers to arrays or structures
. Even an attempt to read a value, which a garbage pointer refers to, can crash a program.
reserves a special
, undefinedness of an expression is denoted as expr↑, and definedness as expr↓.
Value (computer science)
In computer science, a value is an expression which cannot be evaluated any further . The members of a type are the values of that type. For example, the expression "1 + 2" is not a value as it can be reduced to the expression "3"...
is a condition where an expression
Expression (programming)
An expression in a programming language is a combination of explicit values, constants, variables, operators, and functions that are interpreted according to the particular rules of precedence and of association for a particular programming language, which computes and then produces another value...
has not a correct value, although it is syntactically correct. Undefined value may not be confused with empty string
Empty string
In computer science and formal language theory, the empty string is the unique string of length zero. It is denoted with λ or sometimes Λ or ε....
, boolean "false" or other "empty" (but defined) values. Depending on circumstances, evaluation to undefined value may lead to exception or undefined behaviour
Undefined behaviour
In computer programming, undefined behavior is a feature of some programming languages—most famously C. In these languages, to simplify the specification and allow some flexibility in implementation, the specification leaves the results of certain operations specifically undefined.For...
, but in some programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
s undefined values can occur during a normal, predictable course of program execution.
Dynamically typed languages usually treat undefined values explicitly (when possible), e.g. Perl
Perl
Perl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular...
has
undef
operator which can "assign" such value to a variable. In other type systems an undefined value can mean an unknown, unpredictable value, or merely a program failure on attempt of its evaluation. Nullable types offer an intermediate approach; see below.Examples
Value of a partial functionPartial function
In mathematics, a partial function from X to Y is a function ƒ: X' → Y, where X' is a subset of X. It generalizes the concept of a function by not forcing f to map every element of X to an element of Y . If X' = X, then ƒ is called a total function and is equivalent to a function...
is undefined, when its argument is out of domain of definition. This include numerous arithmetic
Arithmetic
Arithmetic or arithmetics is the oldest and most elementary branch of mathematics, used by almost everyone, for tasks ranging from simple day-to-day counting to advanced science and business calculations. It involves the study of quantity, especially as the result of combining numbers...
al cases such as division by zero
Division by zero
In mathematics, division by zero is division where the divisor is zero. Such a division can be formally expressed as a / 0 where a is the dividend . Whether this expression can be assigned a well-defined value depends upon the mathematical setting...
, square root
Square root
In mathematics, a square root of a number x is a number r such that r2 = x, or, in other words, a number r whose square is x...
or logarithm
Logarithm
The logarithm of a number is the exponent by which another fixed value, the base, has to be raised to produce that number. For example, the logarithm of 1000 to base 10 is 3, because 1000 is 10 to the power 3: More generally, if x = by, then y is the logarithm of x to base b, and is written...
of a negative number etc.; see NaN
NaN
In computing, NaN is a value of the numeric data type representing an undefined or unrepresentable value, especially in floating-point calculations...
.
Even some mathematically well-defined expressions like exp
Exponential function
In mathematics, the exponential function is the function ex, where e is the number such that the function ex is its own derivative. The exponential function is used to model a relationship in which a constant change in the independent variable gives the same proportional change In mathematics,...
(100000
100000 (number)
One hundred thousand is the natural number following 99999 and preceding 100001. In scientific notation, it is written as 105.In South Asia, one hundred thousand is called a lakh...
) may be undefined in floating point
Floating point
In computing, floating point describes a method of representing real numbers in a way that can support a wide range of values. Numbers are, in general, represented approximately to a fixed number of significant digits and scaled using an exponent. The base for the scaling is normally 2, 10 or 16...
arithmetic because exceed its limits. If implementation supports ±∞
Extended real number line
In mathematics, the affinely extended real number system is obtained from the real number system R by adding two elements: +∞ and −∞ . The projective extended real number system adds a single object, ∞ and makes no distinction between "positive" or "negative" infinity...
, then this value may be computed as +∞ (inf), however.
Element of an array is undefined when index is out of bounds, as a lookup in an associative array
Associative array
In computer science, an associative array is an abstract data type composed of a collection of pairs, such that each possible key appears at most once in the collection....
for a key which it does not contain.
An argument of variadic function
Variadic function
In computer programming, a variadic function is a function of indefinite arity, i.e., one which accepts a variable number of arguments. Support for variadic functions differs widely among programming languages....
, which was not passed to it, is undefined when function is called.
A variable
Variable (programming)
In computer programming, a variable is a symbolic name given to some known or unknown quantity or information, for the purpose of allowing the name to be used independently of the information it represents...
which is not initialized, has undefined (or unpredictable) value until it was assigned.
Dereference of null pointer lead to undefined value and usually rises an exception immediately.
Any expression of the bottom type
Bottom type
In type theory, a theory within mathematical logic, the bottom type is the type that has no values. It is also called the zero or empty type, and is sometimes denoted with falsum .A function whose return type is bottom cannot return any value...
is undefined by definition, because that type has no values.
The value of a function which loops forever
Halting problem
In computability theory, the halting problem can be stated as follows: Given a description of a computer program, decide whether the program finishes running or continues to run forever...
(for example, in the case of failed μ operator in a partial recursive function) may be seen as undefined too, but only of a theoretical interest because such function never returns.
Treatment
In Perl language, definedness of an expression can be checked via predicatedefined(
expr)
. The use of undefined value in Perl is quite safe, it is equivalent to false in logical context (under if etc.).In such statically typed
Type system
A type system associates a type with each computed value. By examining the flow of these values, a type system attempts to ensure or prove that no type errors can occur...
languages as C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
(C++) there is no specific notion of a value undefined at runtime. Arithmetically undefined expressions invoke exceptions and crash the program, if uncaught
Exception handling
Exception handling is a programming language construct or computer hardware mechanism designed to handle the occurrence of exceptions, special conditions that change the normal flow of program execution....
. Undefined (means, unpredictable) data in C and similar languages may appear in case of flawy designed program or unexpected fault, and represent a severe danger, particularly pointers to deallocated memory
Dangling pointer
Dangling pointers and wild pointers in computer programming are pointers that do not point to a valid object of the appropriate type. These are special cases of memory safety violations....
and null pointers to arrays or structures
Struct (C programming language)
A struct in C programming language is a structured type that aggregates a fixed set of labelled objects, possibly of different types, into a single object.A struct declaration consists of a list of fields, each of which can have any type...
. Even an attempt to read a value, which a garbage pointer refers to, can crash a program.
Undefined value and nullable types
A nullable data typeData type
In computer programming, a data type is a classification identifying one of various types of data, such as floating-point, integer, or Boolean, that determines the possible values for that type; the operations that can be done on values of that type; the meaning of the data; and the way values of...
reserves a special
null
value for representing undefined value, so null value become a kind of value; note that undefined value generally is not. Unlike languages with dynamic typing, a variable of nullable type (as implemented in C#) must be initialized before it can be used.Notation
In computability theoryComputability theory
Computability theory, also called recursion theory, is a branch of mathematical logic that originated in the 1930s with the study of computable functions and Turing degrees. The field has grown to include the study of generalized computability and definability...
, undefinedness of an expression is denoted as expr↑, and definedness as expr↓.