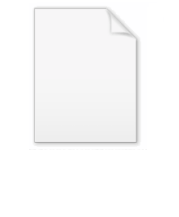
ThinBasic
Encyclopedia
thinBasic is a BASIC
-like computer
programming
language
interpreter
with a central core engine architecture surrounded by many specialized modules. Although originally designed mainly for computer automation, thanks to its modular structure it can be used for wide range of tasks.
Besides those mentioned in the table above, programmer can define pointers, user defined types and unions
.
The special features related to user defined types in ThinBASIC are:
Variables can be defined in global, local or static scope.
ThinBASIC supports arrays of up to 3 dimensions.
To use a module, programmer just has to write:
Uses "ModuleName"
By doing so, modules can immediately provide:
, FreeBASIC
, C
, MASM
).
It provides:
thinAir allows using the debugger as well.
This component is called thinDebug and can be watched on the image linked below.
' Specifies program will use functions from console module
uses "Console"
' TBMain represents main body of the program
function TBMain
' Creates variable to hold user name
local UserName as string
' Asks user for the name
Console_Print("What is your name?: ")
' Stores it to variable
UserName = Console_ReadLine
' If length of username is 0 then no name is specified, else program will say hello
if len(UserName) = 0 then
Console_PrintLine("No user name specified...")
else
Console_PrintLine("Hello " + UserName + "!")
end if
' Waits for any key from user before program ends
Console_WaitKey
end function
Calling function by composing it name at run-time from strings:
' Use File module for handling files
uses "FILE"
function TBMain
' Assign file name to variable, notice assignment can be done at time of declaring variable
local MyFile as string = "c:\File.txt"
local ReturnValue as long
' Functions are named Load_, so we can determine
' the function name we need at run time and save some lines of code
call "Load_"+FILE_PATHSPLIT(MyFile, %Path_Ext) ( MyFile ) To ReturnValue
end function
function Load_TXT( fName as string ) as long
' Code to load TXT file here
end function
function Load_BMP( fName as string ) as long
' Code to load BMP file here
end function
Code to draw 3D cube, which can be manipulated using arrow keys:
note: some keywords not correctly highlighted due to old version of syntax highligher on the Wiki
Uses "TBGL"
Begin Const
' -- Scene IDs
%sScene = 1
' -- Entity IDs
%eCamera = 1
%eLight
%eBox
End Const
Function TBMain
Local hWnd As DWord
Local FrameRate As Double
' -- Create and show window
hWnd = TBGL_CreateWindowEx("TBGL script - press ESC to quit", 640, 480, 32, %TBGL_WS_WINDOWED Or %TBGL_WS_CLOSEBOX)
TBGL_ShowWindow
' -- Create scene
TBGL_SceneCreate(%sScene)
' -- Create basic entities
' -- Create camera to look from 15, 15, 15 to 0, 0, 0
TBGL_EntityCreateCamera(%sScene, %eCamera)
TBGL_EntitySetPos(%sScene, %eCamera, 15, 15, 15)
TBGL_EntitySetTargetPos(%sScene, %eCamera, 0, 0, 0)
' -- Create point light
TBGL_EntityCreateLight(%sScene, %eLight)
TBGL_EntitySetPos(%sScene, %eLight, 15, 10, 5)
' -- Create something to look at
TBGL_EntityCreateBox(%sScene, %eBox, 0, 1, 1, 1, 0, 255, 128, 0)
TBGL_EntitySetPos(%sScene, %eBox, 0, 0, 0)
' -- Resets status of all keys
TBGL_ResetKeyState
' -- Main loop
While TBGL_IsWindow(hWnd)
FrameRate = TBGL_GetFrameRate
TBGL_ClearFrame
TBGL_SceneRender(%sScene)
TBGL_DrawFrame
' -- ESCAPE key to exit application
If TBGL_GetWindowKeyState(hWnd, %VK_ESCAPE) Then Exit While
If TBGL_GetWindowKeyState(hWnd, %VK_LEFT) Then
TBGL_EntityTurn(%sScene, %eBox, 0,-90/FrameRate, 0)
ElseIf TBGL_GetWindowKeyState(hWnd, %VK_RIGHT) Then
TBGL_EntityTurn(%sScene, %eBox, 0, 90/FrameRate, 0)
End If
Wend
TBGL_DestroyWindow
End Function
Disadvantages:
XP Professional
using PowerBASIC, and requires Internet Explorer
version 5.50 or above.
BASIC
BASIC is a family of general-purpose, high-level programming languages whose design philosophy emphasizes ease of use - the name is an acronym from Beginner's All-purpose Symbolic Instruction Code....
-like computer
Computer
A computer is a programmable machine designed to sequentially and automatically carry out a sequence of arithmetic or logical operations. The particular sequence of operations can be changed readily, allowing the computer to solve more than one kind of problem...
programming
Computer programming
Computer programming is the process of designing, writing, testing, debugging, and maintaining the source code of computer programs. This source code is written in one or more programming languages. The purpose of programming is to create a program that performs specific operations or exhibits a...
language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
interpreter
Interpreter (computing)
In computer science, an interpreter normally means a computer program that executes, i.e. performs, instructions written in a programming language...
with a central core engine architecture surrounded by many specialized modules. Although originally designed mainly for computer automation, thanks to its modular structure it can be used for wide range of tasks.
Main Features
- Modular structure
- Rich set of predefined keywords
- User-defined functions can take up to 32 parameters
- All main flow control statements:
- SELECT CASE
- IF ... THEN/ELSEIF/ELSE/END IF
- loops ( infiniteInfinite loopAn infinite loop is a sequence of instructions in a computer program which loops endlessly, either due to the loop having no terminating condition, having one that can never be met, or one that causes the loop to start over...
, conditionalConditional loopIn computer programming, conditional loops or repetitive control structures are a way for computer programs to repeat one or more various steps depending on conditions set either by the programmer initially or real-time by the actual program....
, FORFor loopIn computer science a for loop is a programming language statement which allows code to be repeatedly executed. A for loop is classified as an iteration statement....
, WHILE, DO/LOOP WHILE ..., DO/LOOP UNTIL ...)
- Strong focus on string manipulation
- Implicit line continuation
- Dynamic calling of functions with function name composed at runtime using string expression
- Mechanism of include files allows specify them using wildcard
- Binding to third party DLLs (such as OpenGLOpenGLOpenGL is a standard specification defining a cross-language, cross-platform API for writing applications that produce 2D and 3D computer graphics. The interface consists of over 250 different function calls which can be used to draw complex three-dimensional scenes from simple primitives. OpenGL...
, OpenCLOpenCLOpenCL is a framework for writing programs that execute across heterogeneous platforms consisting of CPUs, GPUs, and other processors. OpenCL includes a language for writing kernels , plus APIs that are used to define and then control the platforms...
, XMLXMLExtensible Markup Language is a set of rules for encoding documents in machine-readable form. It is defined in the XML 1.0 Specification produced by the W3C, and several other related specifications, all gratis open standards....
, ODEOpen Dynamics EngineThe Open Dynamics Engine is a physics engine in C/C++. Its two main components are a rigid body dynamics simulation engine and a collision detection engine...
) can be provided via header fileHeader fileSome programming languages use header files. These files allow programmers to separate certain elements of a program's source code into reusable files. Header files commonly contain forward declarations of classes, subroutines, variables, and other identifiers...
s - Professional and huge help material
- Advanced IDE
- Hundred of examples covering many programming aspects
- A Basic GUI tutorial released as thinBasic source code ready to be used for new programs
Variables and data types
ThinBASIC supports wide range of numeric and string data types.Integer | Floating point | String | Other |
---|---|---|---|
BYTE | SINGLE | STRING | VARIANT Variant type Variant is a data type in certain programming languages, particularly Visual Basic and C++ when using the Component Object Model.In Visual Basic the Variant data type is a tagged union that can be used to represent any other data type except fixed-length string type and... |
WORD | DOUBLE | STRING * n | GUID |
DWORD | CURRENCY | ASCIIZ * n | BOOLEAN |
INTEGER | EXTENDED, EXT | UDT (User defined type) | |
LONG | UNIONS | ||
QUAD |
Besides those mentioned in the table above, programmer can define pointers, user defined types and unions
Union (computer science)
In computer science, a union is a value that may have any of several representations or formats; or a data structure that consists of a variable which may hold such a value. Some programming languages support special data types, called union types, to describe such values and variables...
.
The special features related to user defined types in ThinBASIC are:
- possibility to inherit members from one or more other user defined types
- static members (members whose value is shared among all variables of given UDT)
- dynamic strings
Variables can be defined in global, local or static scope.
ThinBASIC supports arrays of up to 3 dimensions.
Modules
ThinBASIC is being installed with modules. Each module is dedicated to specific area of interest.Module name | Purpose |
---|---|
UI | creation of dialogs and windows, handling of controls, 2D double-buffered graphics |
Console | handling of Windows console |
File | creating, copying and saving information to files |
Eval | formula evaluation |
TBGL | 2D/3D hardware accelerated graphics |
TBDI | Game devices input |
Bundle | script self-bundling to EXE |
INet | Internet communications |
Stat | Statistics performed on arrays, such as median, arithmetic/geometric/harmonic mean, standard deviation, standard error... |
Tokenizer | various functionalities for parsing text |
XPrint | printing of text, shapes and bitmaps |
Oxygen | JIT compiler of machine code, assembler and BASIC like syntax which can be used to speed up the performance of time critical parts of otherwise interpreted ThinBASIC code |
... | ... |
To use a module, programmer just has to write:
Uses "ModuleName"
By doing so, modules can immediately provide:
- functions and procedures implementations
- variable predefinition
- constants predefinition
- user-defined type definition
Customizing the language
Language can be enhanced by module development using SDK for many languages ( PowerBASICPowerBASIC
PowerBASIC is the brand of several commercial compilers by Venice, Florida-based PowerBASIC Inc. that compile a dialect of the BASIC programming language. The DOS versions have a syntax similar to that of QBasic and QuickBASIC, while the Windows versions utilize a standard BASIC syntax that can be...
, FreeBASIC
FreeBASIC
FreeBASIC is a free/open source , 32-bit BASIC compiler for Microsoft Windows, protected-mode DOS , Linux, FreeBSD and Xbox....
, C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
, MASM
Microsoft Macro Assembler
The Microsoft Macro Assembler is an x86 assembler that uses the Intel syntax for Microsoft Windows. there was a version of the Microsoft Macro Assembler for 16-bit and 32-bit assembly sources, MASM, and a different one, ML64, for 64-bit sources only...
).
Integrated development environment (IDE)
ThinBASIC comes with own IDE, called thinAir, in the default installation.It provides:
- Customizable syntax highlighting
- Code templates
- Multiple source files opened at once
- Ability to view one source using multiple views
- Optional script obfuscation
- Creation of independent executable from the script
- Access to the help file
thinAir allows using the debugger as well.
This component is called thinDebug and can be watched on the image linked below.
Code samples
Console program, which asks user about name and then greets him:' Specifies program will use functions from console module
uses "Console"
' TBMain represents main body of the program
function TBMain
' Creates variable to hold user name
local UserName as string
' Asks user for the name
Console_Print("What is your name?: ")
' Stores it to variable
UserName = Console_ReadLine
' If length of username is 0 then no name is specified, else program will say hello
if len(UserName) = 0 then
Console_PrintLine("No user name specified...")
else
Console_PrintLine("Hello " + UserName + "!")
end if
' Waits for any key from user before program ends
Console_WaitKey
end function
Calling function by composing it name at run-time from strings:
' Use File module for handling files
uses "FILE"
function TBMain
' Assign file name to variable, notice assignment can be done at time of declaring variable
local MyFile as string = "c:\File.txt"
local ReturnValue as long
' Functions are named Load_
' the function name we need at run time and save some lines of code
call "Load_"+FILE_PATHSPLIT(MyFile, %Path_Ext) ( MyFile ) To ReturnValue
end function
function Load_TXT( fName as string ) as long
' Code to load TXT file here
end function
function Load_BMP( fName as string ) as long
' Code to load BMP file here
end function
Code to draw 3D cube, which can be manipulated using arrow keys:
note: some keywords not correctly highlighted due to old version of syntax highligher on the Wiki
Uses "TBGL"
Begin Const
' -- Scene IDs
%sScene = 1
' -- Entity IDs
%eCamera = 1
%eLight
%eBox
End Const
Function TBMain
Local hWnd As DWord
Local FrameRate As Double
' -- Create and show window
hWnd = TBGL_CreateWindowEx("TBGL script - press ESC to quit", 640, 480, 32, %TBGL_WS_WINDOWED Or %TBGL_WS_CLOSEBOX)
TBGL_ShowWindow
' -- Create scene
TBGL_SceneCreate(%sScene)
' -- Create basic entities
' -- Create camera to look from 15, 15, 15 to 0, 0, 0
TBGL_EntityCreateCamera(%sScene, %eCamera)
TBGL_EntitySetPos(%sScene, %eCamera, 15, 15, 15)
TBGL_EntitySetTargetPos(%sScene, %eCamera, 0, 0, 0)
' -- Create point light
TBGL_EntityCreateLight(%sScene, %eLight)
TBGL_EntitySetPos(%sScene, %eLight, 15, 10, 5)
' -- Create something to look at
TBGL_EntityCreateBox(%sScene, %eBox, 0, 1, 1, 1, 0, 255, 128, 0)
TBGL_EntitySetPos(%sScene, %eBox, 0, 0, 0)
' -- Resets status of all keys
TBGL_ResetKeyState
' -- Main loop
While TBGL_IsWindow(hWnd)
FrameRate = TBGL_GetFrameRate
TBGL_ClearFrame
TBGL_SceneRender(%sScene)
TBGL_DrawFrame
' -- ESCAPE key to exit application
If TBGL_GetWindowKeyState(hWnd, %VK_ESCAPE) Then Exit While
If TBGL_GetWindowKeyState(hWnd, %VK_LEFT) Then
TBGL_EntityTurn(%sScene, %eBox, 0,-90/FrameRate, 0)
ElseIf TBGL_GetWindowKeyState(hWnd, %VK_RIGHT) Then
TBGL_EntityTurn(%sScene, %eBox, 0, 90/FrameRate, 0)
End If
Wend
TBGL_DestroyWindow
End Function
Pros and cons
Advantages:- Takes full advantage of resources provided by WindowsMicrosoft WindowsMicrosoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal...
platform ( registryWindows registryThe Windows Registry is a hierarchical database that stores configuration settings and options on Microsoft Windows operating systems. It contains settings for low-level operating system components as well as the applications running on the platform: the kernel, device drivers, services, SAM, user...
, user interface, work with processes, COMComponent Object ModelComponent Object Model is a binary-interface standard for software componentry introduced by Microsoft in 1993. It is used to enable interprocess communication and dynamic object creation in a large range of programming languages...
, DLLDynamic-link libraryDynamic-link library , or DLL, is Microsoft's implementation of the shared library concept in the Microsoft Windows and OS/2 operating systems...
s) - Usually fast execution
- Speed optimizations can be done using machine code and assembler
- Under continuous development
- Great string library with tons of functions working on dynamic strings
Disadvantages:
- Only for WindowsMicrosoft WindowsMicrosoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal...
platform - Performance of applications can be lower comparing to output of compilers, thanks to language interpreterInterpreter (computing)In computer science, an interpreter normally means a computer program that executes, i.e. performs, instructions written in a programming language...
nature - Object-oriented programmingObject-oriented programmingObject-oriented programming is a programming paradigm using "objects" – data structures consisting of data fields and methods together with their interactions – to design applications and computer programs. Programming techniques may include features such as data abstraction,...
classes are not implemented
Compatibility
thinBASIC has been developed under Microsoft WindowsMicrosoft Windows
Microsoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal...
XP Professional
Windows XP
Windows XP is an operating system produced by Microsoft for use on personal computers, including home and business desktops, laptops and media centers. First released to computer manufacturers on August 24, 2001, it is the second most popular version of Windows, based on installed user base...
using PowerBASIC, and requires Internet Explorer
Internet Explorer
Windows Internet Explorer is a series of graphical web browsers developed by Microsoft and included as part of the Microsoft Windows line of operating systems, starting in 1995. It was first released as part of the add-on package Plus! for Windows 95 that year...
version 5.50 or above.
- Extensively tested: Windows 2000 and ServerWindows 2000Windows 2000 is a line of operating systems produced by Microsoft for use on personal computers, business desktops, laptops, and servers. Windows 2000 was released to manufacturing on 15 December 1999 and launched to retail on 17 February 2000. It is the successor to Windows NT 4.0, and is the...
, XPWindows XPWindows XP is an operating system produced by Microsoft for use on personal computers, including home and business desktops, laptops and media centers. First released to computer manufacturers on August 24, 2001, it is the second most popular version of Windows, based on installed user base...
, VistaWindows VistaWindows Vista is an operating system released in several variations developed by Microsoft for use on personal computers, including home and business desktops, laptops, tablet PCs, and media center PCs...
, Windows 7, Server 2003Windows Server 2003Windows Server 2003 is a server operating system produced by Microsoft, introduced on 24 April 2003. An updated version, Windows Server 2003 R2, was released to manufacturing on 6 December 2005... - Partly supported: Windows 98 SEWindows 98Windows 98 is a graphical operating system by Microsoft. It is the second major release in the Windows 9x line of operating systems. It was released to manufacturing on 15 May 1998 and to retail on 25 June 1998. Windows 98 is the successor to Windows 95. Like its predecessor, it is a hybrid...
, MeWindows MeWindows Millennium Edition, or Windows Me , is a graphical operating system released on September 14, 2000 by Microsoft, and was the last operating system released in the Windows 9x series. Support for Windows Me ended on July 11, 2006.... - Unsupported: Windows 95Windows 95Windows 95 is a consumer-oriented graphical user interface-based operating system. It was released on August 24, 1995 by Microsoft, and was a significant progression from the company's previous Windows products...
External links
- Official web site
- Community forum
- Online help
- Download page
- thinAir, thinBasic official IDE
- thinDebug, thinBasic Debugger
- Graphics tutorials
- thinBASIC Adventure Builder
- PCOPY! Issue #40, November 16, 2007, About ThinBasic, Eros Olmi.
- PCOPY! Issue #50, March 15, 2007, 3D graphics in ThinBASIC, Petr Schreiber.
- ThinBasic Journal #1, July 5, 2008, PDF
- ThinBasic Journal #2, November 26, 2008, PDF
- MovieFX: Combining photo with 3D object, September 01, 2010
- MovieFX: Blending based bokeh, January 01, 2011
- ThinBasic review at basics.mindteq.com
See also
- Basic4GLBasic4GLBasic4GL is an interpreted, open source version of the BASIC programming language which features support for 3D computer graphics using OpenGL...
- Baltie
- Blitz BasicBlitz BASICBlitz BASIC refers to the programming language dialect that was interpreted by the first Blitz compilers, devised by New Zealand-based developer Mark Sibly. Being derived from BASIC, Blitz syntax was designed to be easy to pick-up for beginners first learning to program...
- Brutus2DBrutus2DBrutus2D is a game programming language developed using Visual Basic, VBScript and DirectX.It is aimed at the amateur programmer who is interested in learning more about programming & game development....
- DarkbasicDarkBASICDarkBASIC is a commercial game creation programming language released by The Game Creators. The language is a structured form of BASIC and is similar to AMOS on the Amiga. The purpose of the language is game creation using Microsoft's DirectX from a BASIC programming language. It is faster and...
- FreeBASICFreeBASICFreeBASIC is a free/open source , 32-bit BASIC compiler for Microsoft Windows, protected-mode DOS , Linux, FreeBSD and Xbox....
- Game MakerGame MakerGameMaker is a Windows and Mac IDE originally developed by Mark Overmars in the Delphi programming language. It is currently developed and published by YoYo Games, a software company in which Overmars is involved...
- Interactive fiction development systems
- List of BASIC dialects
- List of BASIC dialects by platform