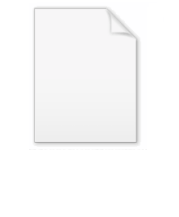
Sequence point
Encyclopedia
A sequence point in imperative programming
defines any point in a computer program
's execution
at which it is guaranteed that all side effect
s of previous evaluations will have been performed, and no side effects from subsequent evaluations have yet been performed. They are often mentioned in reference to C
and C++
, because the result of some expressions can depend on the order of evaluation of their subexpressions. Adding one or more sequence points is one method of ensuring a consistent result, because this restricts the possible orders of evaluation.
s
Sequence points also come into play when the same variable is modified more than once within a single expression. An often-cited example is the C
expression
operators act like functions, and thus operators that have been overloaded introduce sequence points in the same way as function calls.)
Imperative programming
In computer science, imperative programming is a programming paradigm that describes computation in terms of statements that change a program state...
defines any point in a computer program
Computer program
A computer program is a sequence of instructions written to perform a specified task with a computer. A computer requires programs to function, typically executing the program's instructions in a central processor. The program has an executable form that the computer can use directly to execute...
's execution
Execution (computers)
Execution in computer and software engineering is the process by which a computer or a virtual machine carries out the instructions of a computer program. The instructions in the program trigger sequences of simple actions on the executing machine...
at which it is guaranteed that all side effect
Side effect (computer science)
In computer science, a function or expression is said to have a side effect if, in addition to returning a value, it also modifies some state or has an observable interaction with calling functions or the outside world...
s of previous evaluations will have been performed, and no side effects from subsequent evaluations have yet been performed. They are often mentioned in reference to C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
and C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
, because the result of some expressions can depend on the order of evaluation of their subexpressions. Adding one or more sequence points is one method of ensuring a consistent result, because this restricts the possible orders of evaluation.
Examples of ambiguity
Consider two functionSubroutine
In computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....
s
f
and g
. In C and C++, the +
operator is not associated with a sequence point, and therefore in the expressionExpression (programming)
An expression in a programming language is a combination of explicit values, constants, variables, operators, and functions that are interpreted according to the particular rules of precedence and of association for a particular programming language, which computes and then produces another value...
f+g
it is possible that either f
or g
will be executed first. The comma operator introduces a sequence point, and therefore in the code f,g
the order of evaluation is defined: first f
is called, and then g
is called.Sequence points also come into play when the same variable is modified more than once within a single expression. An often-cited example is the C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
expression
i=i++
, which apparently both assigns i
its previous value and increments i
. The final value of i
is ambiguous, because, depending on the order of expression evaluation, the increment may occur before, after, or interleaved with the assignment. The definition of a particular language might specify one of the possible behaviors or simply say the behavior is undefined. In C and C++, evaluating such an expression yields undefined behavior.Sequence points in C and C++
In C and C++, sequence points occur in the following places. (In C++, overloadedOperator overloading
In object oriented computer programming, operator overloading—less commonly known as operator ad-hoc polymorphism—is a specific case of polymorphism, where different operators have different implementations depending on their arguments...
operators act like functions, and thus operators that have been overloaded introduce sequence points in the same way as function calls.)
- Between evaluation of the left and right operands of the && (logical ANDLogical conjunctionIn logic and mathematics, a two-place logical operator and, also known as logical conjunction, results in true if both of its operands are true, otherwise the value of false....
), || (logical ORLogical disjunctionIn logic and mathematics, a two-place logical connective or, is a logical disjunction, also known as inclusive disjunction or alternation, that results in true whenever one or more of its operands are true. E.g. in this context, "A or B" is true if A is true, or if B is true, or if both A and B are...
), and comma operators. For example, in the expression*p++ != 0 && *q++ != 0
, all side effects of the sub-expression*p++ != 0
are completed before any attempt to accessq
.
- Between the evaluation of the first operand of the ternary "question-mark" operator?:In computer programming, ?: is a ternary operator that is part of the syntax for a basic conditional expression in several programming languages...
and the second or third operand. For example, in the expressiona = (*p++) ? (*p++) : 0
there is a sequence point after the first*p++
, meaning it has already been incremented by the time the second instance is executed. - At the end of a full expression. This category includes expression statements (such as the assignment
a=b;
), return statementReturn statementIn computer programming, a return statement causes execution to leave the current subroutine and resume at the point in the code immediately after where the subroutine was called, known as its return address. The return address is saved, usually on the process's call stack, as part of the operation...
s, the controlling expressions ofif
,switch
,while
, ordo
-while
statements, and all three expressions in afor
statement. - Before a function is entered in a function call. The order in which the arguments are evaluated is not specified, but this sequence point means that all of their side effects are complete before the function is entered. In the expression
f(i++) + g(j++) + h(k++)
,f
is called with a parameter of the original value ofi
, buti
is incremented before entering the body off
. Similarly,j
andk
are updated before enteringg
andh
respectively. However, it is not specified in which orderf
,g
,h
are executed, nor in which orderi
,j
,k
are incremented. Variablesj
andk
in the body off
may or may not have been already incremented. Note that a function callf(a,b,c)
is not a use of the comma operatorComma operatorIn the C and C++ programming languages, the comma operator is a binary operator that evaluates its first operand and discards the result, and then evaluates the second operand and returns this value...
and the order of evaluation fora
,b
, andc
is unspecified. - At a function return, after the return value is copied into the calling context. (This sequence point is only specified in the C++ standard; it is present only implicitly in C.)
- At the end of an initializer; for example, after the evaluation of
5
in the declarationint a = 5;
.