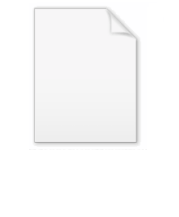
Programming idiom
Encyclopedia
A programming idiom is a means of expressing a recurring construct in one or more programming language
s. Generally speaking, a programming idiom is an expression of a simple task or algorithm
that is not a built-in feature in the programming language being used, or, conversely, the use of an unusual or notable feature that is built in to a programming language. The term can be used more broadly, however, to refer to complex algorithms or programming design patterns.
Knowing the idioms associated with a programming language and how to use them is an important part of gaining fluency
in that language.
, the code to increment a counter by one is mundane:
The C language
, and many others derived from it, have language-specific features that make this code shorter:
There is a difference between the first two expressions, which yield the new version of
Pascal
, as a keyword-centric language, contains a built in procedure for the same operation:
These are the idiomatic ways of "adding one to a counter".
In many languages, code for swapping the values in two variables looks like the following:
In Perl
, the list assignment syntax allows a more succinct expression:
varies widely between different programming languages, although it often takes the form of a while loop
where the test condition is always true. In Pascal, for example:
There are several ways to write an infinite loop in C, including a loop very similar to the Pascal example, but the following idiom uses the unusual appearance of the empty for loop
condition to draw attention visually to the loop:
Perl allows the C syntax above, but supports some other syntax as well. For example:
Ada loops forever this readable way:
Also readable python syntax:
operation: we have to scan the array until we meet the element, or until the end.
Therefore, we create an associative array
in which the array elements are keys, and the value is irrelevant. This assumes an implementation of associative arrays in which lookup is fast (e.g. hash table
s, as in Perl
).
The following idiom is commonly used to express this in Perl:
Read more in the article Opaque pointer
.
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
s. Generally speaking, a programming idiom is an expression of a simple task or algorithm
Algorithm
In mathematics and computer science, an algorithm is an effective method expressed as a finite list of well-defined instructions for calculating a function. Algorithms are used for calculation, data processing, and automated reasoning...
that is not a built-in feature in the programming language being used, or, conversely, the use of an unusual or notable feature that is built in to a programming language. The term can be used more broadly, however, to refer to complex algorithms or programming design patterns.
Knowing the idioms associated with a programming language and how to use them is an important part of gaining fluency
Fluency
Fluency is the property of a person or of a system that delivers information quickly and with expertise.-Speech:...
in that language.
Incrementing a counter
In a language like BASICBASIC
BASIC is a family of general-purpose, high-level programming languages whose design philosophy emphasizes ease of use - the name is an acronym from Beginner's All-purpose Symbolic Instruction Code....
, the code to increment a counter by one is mundane:
i = i + 1
The C language
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
, and many others derived from it, have language-specific features that make this code shorter:
i += 1; /* i = i + 1; */
++i; /* same result */
i++; /* same result */
There is a difference between the first two expressions, which yield the new version of
i
, and the third, which yields the old version of i
. When the expressions are used as isolated statements, as in this example, the yielded value is ignored.Pascal
Pascal (programming language)
Pascal is an influential imperative and procedural programming language, designed in 1968/9 and published in 1970 by Niklaus Wirth as a small and efficient language intended to encourage good programming practices using structured programming and data structuring.A derivative known as Object Pascal...
, as a keyword-centric language, contains a built in procedure for the same operation:
i := i + 1;
Inc(i); (* same *)
These are the idiomatic ways of "adding one to a counter".
Swapping values between variables
- Main article: Swap (computer science)Swap (computer science)In computer programming, the act of swapping two variables refers to mutually exchanging the values of the variables. Usually, this is done with the data in memory...
In many languages, code for swapping the values in two variables looks like the following:
temp = a;
a = b;
b = temp;
In Perl
Perl
Perl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular...
, the list assignment syntax allows a more succinct expression:
($a, $b) = ($b, $a);
Infinite loop
The code used to write an infinite (nonterminating) loopInfinite loop
An infinite loop is a sequence of instructions in a computer program which loops endlessly, either due to the loop having no terminating condition, having one that can never be met, or one that causes the loop to start over...
varies widely between different programming languages, although it often takes the form of a while loop
While loop
In most computer programming languages, a while loop is a control flow statement that allows code to be executed repeatedly based on a given boolean condition. The while loop can be thought of as a repeating if statement....
where the test condition is always true. In Pascal, for example:
while true do begin
do_something;
end;
There are several ways to write an infinite loop in C, including a loop very similar to the Pascal example, but the following idiom uses the unusual appearance of the empty for loop
For loop
In computer science a for loop is a programming language statement which allows code to be repeatedly executed. A for loop is classified as an iteration statement....
condition to draw attention visually to the loop:
for {
do_something;
}
Perl allows the C syntax above, but supports some other syntax as well. For example:
do_something while (1); # Succinct one-line infinite loop
# same as
while (1) { do_something };
# Using a "naked block" and the redo operator
{
do_something;
redo;
}
Ada loops forever this readable way:
loop
do_something;
end loop;
Also readable python syntax:
while True:
do_something
Array lookup hash table
Suppose we have an array of items, and we need to perform an operation in which we often need to determine whether some arbitrary item is in the array or not. Looking up an element in an array is an O(n)Big O notation
In mathematics, big O notation is used to describe the limiting behavior of a function when the argument tends towards a particular value or infinity, usually in terms of simpler functions. It is a member of a larger family of notations that is called Landau notation, Bachmann-Landau notation, or...
operation: we have to scan the array until we meet the element, or until the end.
Therefore, we create an associative array
Associative array
In computer science, an associative array is an abstract data type composed of a collection of pairs, such that each possible key appears at most once in the collection....
in which the array elements are keys, and the value is irrelevant. This assumes an implementation of associative arrays in which lookup is fast (e.g. hash table
Hash table
In computer science, a hash table or hash map is a data structure that uses a hash function to map identifying values, known as keys , to their associated values . Thus, a hash table implements an associative array...
s, as in Perl
Perl
Perl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular...
).
The following idiom is commonly used to express this in Perl:
my %elements = map { $_ => 1 } @elements;
Pimpl Idiom
In OOP the implementation details of a API-level class can be hidden in an own implementation class. A pointer (or reference) to that class is stored in the API-level class.Read more in the article Opaque pointer
Opaque pointer
In computer programming, an opaque pointer is a special case of an opaque data type, a datatype that is declared to be a pointer to a record or data structure of some unspecified type....
.
External links
- C++ programming idioms from Wikibooks.