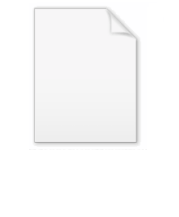
Precompiled header
Encyclopedia
In computer programming
, a precompiled header
is a technique used by some C
or C++
compiler
s to reduce compilation time
.
and C++
programming language
s, a header file
is a file whose text may be automatically included in another source file
by the C preprocessor
by the use of a compiler directive in the source file.
Header files can sometimes contain very large amounts of source code (for instance, the header files
and Mac OS X
, respectively).
To reduce compilation times, some compilers allow header files to be compiled into a form that is faster for the compiler to process. This intermediate form is known as a precompiled header, and is commonly held in a file named with the extension .pch or similar, such as .gch under the GNU Compiler Collection
.
A related feature is the prefix header
, which is a file that is automatically included by the compiler without requiring the use of any compiler directives. Prefix headers are commonly precompiled, but not all precompiled headers are prefix headers.
//header.hpp
...
//source.cpp
...
When compiling source.cpp for the first time with the precompiled header feature turned on, the compiler will generate a precompiled header,
class A {
...
};
Suppose class B refers to an attribute of class A.
class B1 {
...
A mA;
};
If the definition of class A were to change, B.hpp would have to be recompiled. To avoid this, a programmer might refer to A by a pointer. Under this syntax, B.hpp would be:
class A;
class B2 {
...
A *mA;
};
As can be seen, a forward declaration of class A is used instead of including the header. But this pointer syntax complicates the usage of the variable as price for lesser compilation.
This problem is solved by the usage of precompiled headers. When the files are compiled for the first time, A.hpp and B.hpp are compiled into A.gch and B.pch, and A.cpp and B.cpp are compiled into their object file
s. When the definition of class A is changed, B.hpp does not have to be parsed again.
IDE
wizards
, that describes both standard system and project specific include files
that are used frequently but hardly ever change.
Compatible compilers (for example, Visual C++ 6.0 and newer) will precompile this file to reduce overall compile times. Visual C++ will not compile anything before the
The AFX in stdafx.h stands for Application Framework eXtensions. AFX was the original abbreviation for the Microsoft Foundation Classes (MFC). While the name stdafx.h is used by default, projects may specify an alternative name.
(3.4 and newer). GCC's approach is similar to these of VC and compatible compilers. GCC saves precompiled versions of header files using a ".gch" suffix. When compiling a source file, the compiler checks whether this file is present in the same directory and uses it if possible.
GCC can only use the precompiled version if the same compiler switches are set as when the header was compiled and it may use at most one. Further, only preprocessor instructions may be placed before the precompiled header (because it must be directly or indirectly included through another normal header, before any compilable code).
GCC automatically identifies most header files by their extension. However, if this fails (e.g. because of non-standard header extensions), the -x switch can be used to ensure that GCC treats the file as a header.
, it is common to include the
In addition, C++Builder can be instrumented to use a specific header file as precompiled header, similar to the mechanism provided by Visual C++.
C++Builder 2009 introduces a "Precompiled Header Wizard" which parses all source modules of the project for included header files, classifies them (i.e. excludes header files if they are part of the project or do not have an Include guard
) and generates and tests a precompiled header for the specified files automatically.
Computer programming
Computer programming is the process of designing, writing, testing, debugging, and maintaining the source code of computer programs. This source code is written in one or more programming languages. The purpose of programming is to create a program that performs specific operations or exhibits a...
, a precompiled header
Header file
Some programming languages use header files. These files allow programmers to separate certain elements of a program's source code into reusable files. Header files commonly contain forward declarations of classes, subroutines, variables, and other identifiers...
is a technique used by some C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
or C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
compiler
Compiler
A compiler is a computer program that transforms source code written in a programming language into another computer language...
s to reduce compilation time
Compile time
In computer science, compile time refers to either the operations performed by a compiler , programming language requirements that must be met by source code for it to be successfully compiled , or properties of the program that can be reasoned about at compile time.The operations performed at...
.
Overview
In the CC (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
and C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
s, a header file
Header file
Some programming languages use header files. These files allow programmers to separate certain elements of a program's source code into reusable files. Header files commonly contain forward declarations of classes, subroutines, variables, and other identifiers...
is a file whose text may be automatically included in another source file
Source code
In computer science, source code is text written using the format and syntax of the programming language that it is being written in. Such a language is specially designed to facilitate the work of computer programmers, who specify the actions to be performed by a computer mostly by writing source...
by the C preprocessor
C preprocessor
The C preprocessor is the preprocessor for the C and C++ computer programming languages. The preprocessor handles directives for source file inclusion , macro definitions , and conditional inclusion ....
by the use of a compiler directive in the source file.
Header files can sometimes contain very large amounts of source code (for instance, the header files
windows.h
and Cocoa/Cocoa.h
on Microsoft WindowsMicrosoft Windows
Microsoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal...
and Mac OS X
Mac OS X
Mac OS X is a series of Unix-based operating systems and graphical user interfaces developed, marketed, and sold by Apple Inc. Since 2002, has been included with all new Macintosh computer systems...
, respectively).
To reduce compilation times, some compilers allow header files to be compiled into a form that is faster for the compiler to process. This intermediate form is known as a precompiled header, and is commonly held in a file named with the extension .pch or similar, such as .gch under the GNU Compiler Collection
GNU Compiler Collection
The GNU Compiler Collection is a compiler system produced by the GNU Project supporting various programming languages. GCC is a key component of the GNU toolchain...
.
A related feature is the prefix header
Prefix header
In computer programming, a prefix header is a feature found in some C or C++ compilers used to simplify code and/or to reduce compilation time.- Overview :...
, which is a file that is automatically included by the compiler without requiring the use of any compiler directives. Prefix headers are commonly precompiled, but not all precompiled headers are prefix headers.
Simple Example
Given a C++ file source.cpp that includes header.hpp://header.hpp
...
//source.cpp
- include "header.hpp"
...
When compiling source.cpp for the first time with the precompiled header feature turned on, the compiler will generate a precompiled header,
header.pch
. The next time, the compiler can skip the compilation phase relating to header.hpp
and instead use header.pch directly.Forward declaration
We have two classes: A (defined in A.hpp and implemented in A.cpp) and B (defined in B.hpp and implemented in B.cpp). Class A is quite simple:class A {
...
};
Suppose class B refers to an attribute of class A.
- include "A.hpp"
class B1 {
...
A mA;
};
If the definition of class A were to change, B.hpp would have to be recompiled. To avoid this, a programmer might refer to A by a pointer. Under this syntax, B.hpp would be:
class A;
class B2 {
...
A *mA;
};
As can be seen, a forward declaration of class A is used instead of including the header. But this pointer syntax complicates the usage of the variable as price for lesser compilation.
This problem is solved by the usage of precompiled headers. When the files are compiled for the first time, A.hpp and B.hpp are compiled into A.gch and B.pch, and A.cpp and B.cpp are compiled into their object file
Object file
An object file is a file containing relocatable format machine code that is usually not directly executable. Object files are produced by an assembler, compiler, or other language translator, and used as input to the linker....
s. When the definition of class A is changed, B.hpp does not have to be parsed again.
stdafx.h
stdafx.h is a file, generated by Microsoft Visual StudioMicrosoft Visual Studio
Microsoft Visual Studio is an integrated development environment from Microsoft. It is used to develop console and graphical user interface applications along with Windows Forms applications, web sites, web applications, and web services in both native code together with managed code for all...
IDE
Integrated development environment
An integrated development environment is a software application that provides comprehensive facilities to computer programmers for software development...
wizards
Wizard (software)
A software wizard or setup assistant is a user interface type that presents a user with a sequence of dialog boxes that lead the user through a series of well-defined steps. Tasks that are complex, infrequently performed, or unfamiliar may be easier to perform using a wizard...
, that describes both standard system and project specific include files
Header file
Some programming languages use header files. These files allow programmers to separate certain elements of a program's source code into reusable files. Header files commonly contain forward declarations of classes, subroutines, variables, and other identifiers...
that are used frequently but hardly ever change.
Compatible compilers (for example, Visual C++ 6.0 and newer) will precompile this file to reduce overall compile times. Visual C++ will not compile anything before the
#include "stdafx.h"
in the source file, unless the compile option /Yu'stdafx.h'
is unchecked (by default); it assumes all code in the source up to and including that line is already compiled.The AFX in stdafx.h stands for Application Framework eXtensions. AFX was the original abbreviation for the Microsoft Foundation Classes (MFC). While the name stdafx.h is used by default, projects may specify an alternative name.
GCC
Precompiled headers are supported in GCCGNU Compiler Collection
The GNU Compiler Collection is a compiler system produced by the GNU Project supporting various programming languages. GCC is a key component of the GNU toolchain...
(3.4 and newer). GCC's approach is similar to these of VC and compatible compilers. GCC saves precompiled versions of header files using a ".gch" suffix. When compiling a source file, the compiler checks whether this file is present in the same directory and uses it if possible.
GCC can only use the precompiled version if the same compiler switches are set as when the header was compiled and it may use at most one. Further, only preprocessor instructions may be placed before the precompiled header (because it must be directly or indirectly included through another normal header, before any compilable code).
GCC automatically identifies most header files by their extension. However, if this fails (e.g. because of non-standard header extensions), the -x switch can be used to ensure that GCC treats the file as a header.
C++Builder
In the default project configuration, the C++Builder compiler implicitly generates precompiled headers for all headers included by a source module until the line#pragma hdrstop
is found. Precompiled headers are shared for all modules of the project if possible. For example, when working with the Visual Component LibraryVisual Component Library
VCL is a visual component-based object-oriented framework for developing Microsoft Windows applications. It was developed by Borland for use in, and tightly integrated with, its Delphi and C++Builder RAD tools...
, it is common to include the
vcl.h
header first which contains most of the commonly used VCL header files. Thus, the precompiled header can be shared across all project modules, which dramatically reduces the build times.In addition, C++Builder can be instrumented to use a specific header file as precompiled header, similar to the mechanism provided by Visual C++.
C++Builder 2009 introduces a "Precompiled Header Wizard" which parses all source modules of the project for included header files, classifies them (i.e. excludes header files if they are part of the project or do not have an Include guard
Include guard
In the C and C++ programming languages, an #include guard, sometimes called a macro guard, is a particular construct used to avoid the problem of double inclusion when dealing with the #include directive...
) and generates and tests a precompiled header for the specified files automatically.