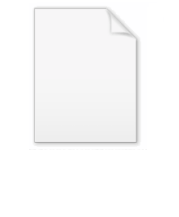
Oz (programming language)
Encyclopedia
Oz is a multiparadigm programming language, developed in the Programming Systems Lab at Université catholique de Louvain
, for programming language education. It has a canonical textbook: Concepts, Techniques, and Models of Computer Programming
.
Oz was first designed by Gert Smolka and his students in 1991. In 1996 the development of Oz continued in cooperation with the research group of Seif Haridi and Peter Van Roy at the Swedish Institute of Computer Science
. Since 1999, Oz has been continually developed by an international group, the Mozart Consortium, which originally consisted of Saarland University
, the Swedish Institute of Computer Science
, and the Université catholique de Louvain
. In 2005, the responsibility for managing Mozart development was transferred to a core group, the Mozart Board, with the express purpose of opening Mozart development to a larger community.
The Mozart Programming System
is the primary implementation of Oz. It is released with an open source
license by the Mozart Consortium. Mozart has been ported to different flavors of Unix
, FreeBSD
, Linux
, Microsoft Windows
, and Mac OS X
.
s, including logic, functional (both lazy
and eager
), imperative, object-oriented, constraint, distributed, and concurrent programming. Oz has both a simple formal semantics (see chapter 13 of the book mentioned below) and an efficient implementation. Oz is a concurrency
-oriented language, as the term was introduced by Joe Armstrong, the main designer of the Erlang language. A concurrency-oriented language makes concurrency both easy to use and efficient. Oz supports a canonical GUI language QTk.
In addition to multi-paradigm programming, the major strengths of Oz are in constraint programming
and distributed programming. Due to its factored design, Oz is able to successfully implement a network-transparent distributed programming model. This model makes it easy to program open, fault-tolerant applications within the language. For constraint programming, Oz introduces the idea of "computation spaces"; these allow user-defined search and distribution strategies orthogonal to the constraint domain.
.
Basic data structures:
Those data structures are values (constant), first class
and dynamically type checked.
It is not possible to change the value of a dataflow variable once it is bound:
Dataflow variables make it easy to create concurrent stream agents:
Because of the way dataflow variables works it is possible to put threads anywhere in the program and it is guaranteed that it will have the same result. This makes concurrent programming very easy. Threads are very cheap: it is possible to have a hundred thousand threads running at once.
algorithm by recursively creating concurrent stream agents that filter out non-prime numbers:
by default, but lazy evaluation
is possible:
With a port and a thread the programmer can define asynchronous agents:
With these simple semantic changes we can support the whole object-oriented paradigm.
With a little syntactic sugar OOP becomes well integrated in Oz.
See also
External links
Université catholique de Louvain
The Université catholique de Louvain, sometimes known, especially in Belgium, as UCL, is Belgium's largest French-speaking university. It is located in Louvain-la-Neuve and in Brussels...
, for programming language education. It has a canonical textbook: Concepts, Techniques, and Models of Computer Programming
Concepts, Techniques, and Models of Computer Programming
Concepts, Techniques, and Models of Computer Programming is a textbook published in 2004 about general computer programming concepts from MIT Press written by Université catholique de Louvain professor Peter Van Roy and Royal Institute of Technology, Sweden professor Seif Haridi.Using a carefully...
.
Oz was first designed by Gert Smolka and his students in 1991. In 1996 the development of Oz continued in cooperation with the research group of Seif Haridi and Peter Van Roy at the Swedish Institute of Computer Science
Swedish Institute of Computer Science
The Swedish Institute of Computer Science, SICS, is an independent non-profit research organization with a research focus on applied computer science. The institute carries out research in a number of areas, including networked embedded systems, future Internet technologies, large scale...
. Since 1999, Oz has been continually developed by an international group, the Mozart Consortium, which originally consisted of Saarland University
Saarland University
Saarland University is a university located in Saarbrücken, the capital of the German state of Saarland, and Homburg. It was founded in 1948 in Homburg in co-operation with France and is organized in 8 faculties that cover all major fields of science...
, the Swedish Institute of Computer Science
Swedish Institute of Computer Science
The Swedish Institute of Computer Science, SICS, is an independent non-profit research organization with a research focus on applied computer science. The institute carries out research in a number of areas, including networked embedded systems, future Internet technologies, large scale...
, and the Université catholique de Louvain
Université catholique de Louvain
The Université catholique de Louvain, sometimes known, especially in Belgium, as UCL, is Belgium's largest French-speaking university. It is located in Louvain-la-Neuve and in Brussels...
. In 2005, the responsibility for managing Mozart development was transferred to a core group, the Mozart Board, with the express purpose of opening Mozart development to a larger community.
The Mozart Programming System
Mozart Programming System
The Mozart Programming System is a multiplatform implementation of the Oz programming language developed by an international group, the Mozart Consortium, which originally consisted of Saarland University, the Swedish Institute of Computer Science, and the Université catholique de Louvain.Mozart...
is the primary implementation of Oz. It is released with an open source
Open source
The term open source describes practices in production and development that promote access to the end product's source materials. Some consider open source a philosophy, others consider it a pragmatic methodology...
license by the Mozart Consortium. Mozart has been ported to different flavors of Unix
Unix
Unix is a multitasking, multi-user computer operating system originally developed in 1969 by a group of AT&T employees at Bell Labs, including Ken Thompson, Dennis Ritchie, Brian Kernighan, Douglas McIlroy, and Joe Ossanna...
, FreeBSD
FreeBSD
FreeBSD is a free Unix-like operating system descended from AT&T UNIX via BSD UNIX. Although for legal reasons FreeBSD cannot be called “UNIX”, as the direct descendant of BSD UNIX , FreeBSD’s internals and system APIs are UNIX-compliant...
, Linux
Linux
Linux is a Unix-like computer operating system assembled under the model of free and open source software development and distribution. The defining component of any Linux system is the Linux kernel, an operating system kernel first released October 5, 1991 by Linus Torvalds...
, Microsoft Windows
Microsoft Windows
Microsoft Windows is a series of operating systems produced by Microsoft.Microsoft introduced an operating environment named Windows on November 20, 1985 as an add-on to MS-DOS in response to the growing interest in graphical user interfaces . Microsoft Windows came to dominate the world's personal...
, and Mac OS X
Mac OS X
Mac OS X is a series of Unix-based operating systems and graphical user interfaces developed, marketed, and sold by Apple Inc. Since 2002, has been included with all new Macintosh computer systems...
.
Language features
Oz contains most of the concepts of the major programming paradigmProgramming paradigm
A programming paradigm is a fundamental style of computer programming. Paradigms differ in the concepts and abstractions used to represent the elements of a program and the steps that compose a computation A programming paradigm is a fundamental style of computer programming. (Compare with a...
s, including logic, functional (both lazy
Lazy evaluation
In programming language theory, lazy evaluation or call-by-need is an evaluation strategy which delays the evaluation of an expression until the value of this is actually required and which also avoids repeated evaluations...
and eager
Eager evaluation
In computer programming, eager evaluation or greedy evaluation is the evaluation strategy in most traditional programming languages. In eager evaluation an expression is evaluated as soon as it gets bound to a variable. The term is typically used to contrast lazy evaluation, where expressions are...
), imperative, object-oriented, constraint, distributed, and concurrent programming. Oz has both a simple formal semantics (see chapter 13 of the book mentioned below) and an efficient implementation. Oz is a concurrency
Concurrency (computer science)
In computer science, concurrency is a property of systems in which several computations are executing simultaneously, and potentially interacting with each other...
-oriented language, as the term was introduced by Joe Armstrong, the main designer of the Erlang language. A concurrency-oriented language makes concurrency both easy to use and efficient. Oz supports a canonical GUI language QTk.
In addition to multi-paradigm programming, the major strengths of Oz are in constraint programming
Constraint programming
Constraint programming is a programming paradigm wherein relations between variables are stated in the form of constraints. Constraints differ from the common primitives of imperative programming languages in that they do not specify a step or sequence of steps to execute, but rather the properties...
and distributed programming. Due to its factored design, Oz is able to successfully implement a network-transparent distributed programming model. This model makes it easy to program open, fault-tolerant applications within the language. For constraint programming, Oz introduces the idea of "computation spaces"; these allow user-defined search and distribution strategies orthogonal to the constraint domain.
Data structures
Oz is based on a core language with very few datatypes that can be extended into more practical ones through syntactic sugarSyntactic sugar
Syntactic sugar is a computer science term that refers to syntax within a programming language that is designed to make things easier to read or to express....
.
Basic data structures:
- Numbers: floating points or integer (real integer).
- Records: for grouping data :
circle(x:0 y:1 radius:3 color:blue style:dots)
- Lists: a simple linear structure,
'|'(2 '|'(4 '|'(6 '|'(8 nil))))
2|(4|(6|(8|nil))) % syntactic sugar
2|4|6|8|nil % more syntactic sugar
[2 4 6 8] % even more syntactic sugar
Those data structures are values (constant), first class
First-class object
In programming language design, a first-class citizen , in the context of a particular programming language, is an entity that can be constructed at run-time, passed as a parameter, returned from a subroutine, or assigned into a variable...
and dynamically type checked.
Functions
Functions are first class values, allowing higher order functional programming:
fun {Fact N}
if N =< 0 then 1 else N*{Fact N-1} end
end
fun {Comb N K}
{Fact N} div ({Fact K} * {Fact N-K}) % integers can't overflow in Oz (unless no memory is left)
end
fun {SumList List}
case List of nil then 0
[] H|T then H+{SumList T} % pattern matching on lists
end
end
Dataflow variables and declarative concurrency
When the program encounters an unbound variable it waits for a value:
thread
Z = X+Y % will wait until both X and Y are bound to a value.
{Browse Z} % shows the value of Z.
end
thread X = 40 end
thread Y = 2 end
It is not possible to change the value of a dataflow variable once it is bound:
X = 1
X = 2 % error
Dataflow variables make it easy to create concurrent stream agents:
fun {Ints N Max}
if N Max then nil
else
{Delay 1000}
N|{Ints N+1 Max}
end
end
fun {Sum S Stream}
case Stream of nil then S
[] H|T then S|{Sum H+S T} end
end
local X Y in
thread X = {Ints 0 1000} end
thread Y = {Sum 0 X} end
{Browse Y}
end
Because of the way dataflow variables works it is possible to put threads anywhere in the program and it is guaranteed that it will have the same result. This makes concurrent programming very easy. Threads are very cheap: it is possible to have a hundred thousand threads running at once.
Example: Trial division sieve
This example computes a stream of prime numbers using the Trial divisionTrial division
Trial division is the most laborious but easiest to understand of the integer factorization algorithms. Its ease of implementation makes it a viable integer factorization option for devices with little available memory, such as graphing calculators....
algorithm by recursively creating concurrent stream agents that filter out non-prime numbers:
fun {Sieve Xs}
case Xs of nil then nil
[] X|Xr then Ys in
thread Ys = {Filter Xr fun {$ Y} Y mod X \= 0 end} end
X|{Sieve Ys}
end
end
Laziness
Oz uses eager evaluationEager evaluation
In computer programming, eager evaluation or greedy evaluation is the evaluation strategy in most traditional programming languages. In eager evaluation an expression is evaluated as soon as it gets bound to a variable. The term is typically used to contrast lazy evaluation, where expressions are...
by default, but lazy evaluation
Lazy evaluation
In programming language theory, lazy evaluation or call-by-need is an evaluation strategy which delays the evaluation of an expression until the value of this is actually required and which also avoids repeated evaluations...
is possible:
fun lazy {Fact N}
if N =< 0 then 1 else N*{Fact N-1} end
end
local X Y in
X = {Fact 100}
Y = X + 1 % the value of X is needed and fact is computed
end
Message passing concurrency
The declarative concurrent model can be extended with message passing through simple semantics:
declare
local Stream Port in
Port = {NewPort Stream}
{Send Port 1} % Stream is now 1|_ ('_' indicates an unbound and unnamed variable)
{Send Port 2} % Stream is now 1|2|_
...
{Send Port n} % Stream is now 1|2| .. |n|_
end
With a port and a thread the programmer can define asynchronous agents:
fun {NewAgent Init Fun}
Msg Out in
thread {FoldL Msg Fun Init Out} end
{NewPort Msg}
end
State and objects
It is again possible to extend the declarative model to support state and object-oriented programming with very simple semantics; we create a new mutable data structure called Cells:
local A X in
A = {NewCell 0}
A := 1 % changes the value of A to 1
X = @A % @ is used to access the value of A
end
With these simple semantic changes we can support the whole object-oriented paradigm.
With a little syntactic sugar OOP becomes well integrated in Oz.
class Counter
attr val
meth init(Value)
val:=Value
end
meth browse
{Browse @val}
end
meth inc(Value)
val :=@val+Value
end
end
local C in
C = {New Counter init(0)}
{C inc(6)}
{C browse}
end
See also
- AliceAlice (programming language)Alice ML is a functional programming language designed by the at Saarland University. It is a dialect of Standard ML, augmented with support for lazy evaluation, concurrency and constraint programming.-Overview:Alice extends Standard ML in a number of ways that distinguish it from its predecessor...
, the concurrent functional constraint programming language from Saarland University - Curry, a functional logic programming language
- Mercury, a functional logic programming language
- Dataflow programming
- Visual PrologVisual PrologVisual Prolog, also formerly known as PDC Prolog and Turbo Prolog, is a strongly typed object-oriented extension of Prolog. As Turbo Prolog it was marketed by Borland, but it is now developed and marketed by the Danish firm Prolog Development Center that originally developed it...
, an object-oriented, functional, logic programming language
External links
- A quick Oz overview
- Tutorial of Oz
- Oz Mozart Tutorial in a blog format
- Programming Language Research at UCL: One of the core developers of Mozart/Oz, this group does research using Mozart/Oz as the vehicle
- Multiparadigm Programming in Mozart/Oz: Proceedings of MOZ 2004: Conference which gives a snapshot of the work being done with Mozart/Oz