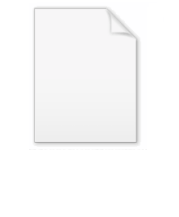
Math.h
Encyclopedia
C mathematical operations are a group of functions in the standard library
of the C programming language implementing basic mathematical functions. Most of the functions involve the use of floating point
numbers. Different C standards provide different albeit backwards-compatible, sets of functions. C mathematical functions are inherited in C++
.
Any functions that operate on angles use radians as the unit of angle.
In C89, all functions accept only type
of an integral value
Exponential functions
Power functions
Trigonometric functions
Hyperbolic functions
Error and gamma functions
Nearest integer floating point operations
Floating point manipulation functions
Classification
).
All operations on complex numbers are defined in
Basic operations
Exponentiation operations
Trigonometric operations
Hyperbolic operations
C standard library
The C Standard Library is the standard library for the programming language C, as specified in the ANSI C standard.. It was developed at the same time as the C POSIX library, which is basically a superset of it...
of the C programming language implementing basic mathematical functions. Most of the functions involve the use of floating point
Floating point
In computing, floating point describes a method of representing real numbers in a way that can support a wide range of values. Numbers are, in general, represented approximately to a fixed number of significant digits and scaled using an exponent. The base for the scaling is normally 2, 10 or 16...
numbers. Different C standards provide different albeit backwards-compatible, sets of functions. C mathematical functions are inherited in C++
C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
.
Overview of functions
Most of the mathematical functions are placed inmath.h
header (cmath
header in C++). The functions that operate on integers, such as abs
, labs
, div
, and ldiv
, are instead specified in the stdlib.h
header (cstdlib
header in C++).Any functions that operate on angles use radians as the unit of angle.
In C89, all functions accept only type
double
for the floating-point arguments. In C99, this limitation was fixed by introducing new sets of functions with f
and l
suffixes that work on float
and long double
arguments respectively.abs
, labs
, llabs
- computes absolute valueAbsolute value
In mathematics, the absolute value |a| of a real number a is the numerical value of a without regard to its sign. So, for example, the absolute value of 3 is 3, and the absolute value of -3 is also 3...
of an integral value
fabs
- computes absolute value of a floating point valuediv
, ldiv
, lldiv
- computes the quotient and remainder of integer divisionfmod
- remainder of the floating point division operationremainder
- signed remainder of the division operationremquo
- signed remainder as well as the three last bits of the division operationfma
- fused multiply-add operationfmax
- larger of two floating point valuesfmin
- smaller of two floating point valuesfdim
- positive difference of two floating point valuesnan
, nanf
, nanl
- returns a not-a-number (NaN)Exponential functions
exp
- returns e raised to the given powerexp2
- returns 2 raised to the given powerexpm1
- returns e raised to the given power, minus onelog
- computes natural (base e) logarithm (to base e)log10
- computes common (base 10) logarithmlog1p
- computes natural logarithm (to base e) of 1 plus the given numberilogb
- extracts exponent of the numberlogb
- extracts exponent of the numberPower functions
sqrt
- computes square rootSquare root
In mathematics, a square root of a number x is a number r such that r2 = x, or, in other words, a number r whose square is x...
cbrt
- computes cubic roothypot
- computes square root of the sum of the squares of two given numberspow
- raises a number to the given powerTrigonometric functions
sin
- computes sineSine
In mathematics, the sine function is a function of an angle. In a right triangle, sine gives the ratio of the length of the side opposite to an angle to the length of the hypotenuse.Sine is usually listed first amongst the trigonometric functions....
cos
- computes cosinetan
- computes tangentTangent
In geometry, the tangent line to a plane curve at a given point is the straight line that "just touches" the curve at that point. More precisely, a straight line is said to be a tangent of a curve at a point on the curve if the line passes through the point on the curve and has slope where f...
asin
- computes arc sineInverse hyperbolic function
The inverses of the hyperbolic functions are the area hyperbolic functions. The names hint at the fact that they give the area of a sector of the unit hyperbola in the same way that the inverse trigonometric functions give the arc length of a sector on the unit circle...
acos
- computes arc cosineInverse hyperbolic function
The inverses of the hyperbolic functions are the area hyperbolic functions. The names hint at the fact that they give the area of a sector of the unit hyperbola in the same way that the inverse trigonometric functions give the arc length of a sector on the unit circle...
atan
- computes arc tangentInverse hyperbolic function
The inverses of the hyperbolic functions are the area hyperbolic functions. The names hint at the fact that they give the area of a sector of the unit hyperbola in the same way that the inverse trigonometric functions give the arc length of a sector on the unit circle...
atan2
- computes arc tangent, using signs to determine quadrantsHyperbolic functions
sinh
- computes hyperbolic sinecosh
- computes hyperbolic cosinetanh
- computes hyperbolic tangentasinh
- computes hyperbolic arc sineacosh
- computes hyperbolic arc cosineatanh
- computes hyperbolic arc tangentError and gamma functions
erf
- computes error functionError function
In mathematics, the error function is a special function of sigmoid shape which occurs in probability, statistics and partial differential equations...
erfc
- computes complementary error functionlgamma
- computes natural logarithm of the gamma functionGamma function
In mathematics, the gamma function is an extension of the factorial function, with its argument shifted down by 1, to real and complex numbers...
tgamma
- computes gamma functionNearest integer floating point operations
ceil
- returns the nearest integer not less than the given valuefloor
- returns the nearest integer not greater than the given valuetrunc
- returns the nearest integer not greater in magnitude than the given valueround
, lround
, llround
- returns the nearest integer, rounding away from zero in halfway casesnearbyint
- returns the nearest integer using current rounding moderint
, lrint
, llrint
- returns the nearest integer using current rounding mode with exception if the result differsFloating point manipulation functions
frexp
- decomposes a number into significand and a power of 2ldexp
- multiplies a number by 2 raised to a powermodf
- decomposes a number into integer and fractional partsscalbn
, scalbln
- multiplies a number by FLT_RADIX raised to a powernextafter
, nexttoward
- returns next representable floating point value towards the given valuecopysign
- copies the sign of a floating point valueClassification
fpclassify
- categorizes the given floating point valueisfinite
- checks if the given number has finite valueisinf
- checks if the given number is infiniteisnan
- checks if the given number is NaNisnormal
- checks if the given number is normalsignbit
- checks if the given number is negativeFloating point environment
C99 adds several functions and types for fine-grained control of floating point computations. The additional functions and types are defined infenv.h
header (cfenv
in C++C++
C++ is a statically typed, free-form, multi-paradigm, compiled, general-purpose programming language. It is regarded as an intermediate-level language, as it comprises a combination of both high-level and low-level language features. It was developed by Bjarne Stroustrup starting in 1979 at Bell...
).
-
- clears exceptions
-
- stores current floating-point environment
-
- stores current status flags
-
- retrieves current rounding direction
-
- saves current floating-point environment and clears all exceptions
-
- raises a floating-point exceptions
-
- sets current floating-point environment
-
- sets current status flags
-
- sets current rounding direction
-
- tests whether certain exceptions have been raised
-
- restores floating-point environment, but keep current exceptions
-
- sets the precision mode
Complex numbers
C99 adds a new_Complex
keyword that provides support for complex numbers. Any floating point type can be modified with _Complex
. In that case, a variable of such type contains a pair of floating point numbers and in such a way defines a complex number. C++ does not provide complex numbers in backwards compatible way. As an alternative, can be used.All operations on complex numbers are defined in
complex.h
header.Basic operations
- computes absolute value
- computes argument of a complex number
Arg (mathematics)In mathematics, arg is a function operating on complex numbers . It gives the angle between the line joining the point to the origin and the positive real axis, shown as in figure 1 opposite, known as an argument of the point In mathematics, arg is a function operating on complex numbers...- computes imaginary part of a complex number
- computes real part of a complex number
- computes complex conjugate
Complex conjugateIn mathematics, complex conjugates are a pair of complex numbers, both having the same real part, but with imaginary parts of equal magnitude and opposite signs...- computes complex projection into the Riemann sphere
Riemann sphereIn mathematics, the Riemann sphere , named after the 19th century mathematician Bernhard Riemann, is the sphere obtained from the complex plane by adding a point at infinity...
Exponentiation operations
- computes complex exponential
Exponential functionIn mathematics, the exponential function is the function ex, where e is the number such that the function ex is its own derivative. The exponential function is used to model a relationship in which a constant change in the independent variable gives the same proportional change In mathematics,...- computes complex logarithm
LogarithmThe logarithm of a number is the exponent by which another fixed value, the base, has to be raised to produce that number. For example, the logarithm of 1000 to base 10 is 3, because 1000 is 10 to the power 3: More generally, if x = by, then y is the logarithm of x to base b, and is written...- computes complex square root
Square rootIn mathematics, a square root of a number x is a number r such that r2 = x, or, in other words, a number r whose square is x...- computes complex power
ExponentiationExponentiation is a mathematical operation, written as an, involving two numbers, the base a and the exponent n...
Trigonometric operations
- computes complex sine
SineIn mathematics, the sine function is a function of an angle. In a right triangle, sine gives the ratio of the length of the side opposite to an angle to the length of the hypotenuse.Sine is usually listed first amongst the trigonometric functions....- computes complex cosine
- computes complex tangent
- computes complex arc sine
- computes complex arc cosine
- computes complex arc tangent
Hyperbolic operations
- computes complex hyperbolic sine
- computes complex hyperbolic cosine
- computes complex hyperbolic tangent
- computes complex hyperbolic arc sine
- computes complex hyperbolic arc cosine
- computes complex hyperbolic arc tangent
Type-generic functions
The headertgmath.h
defines a type-generic macro for each mathematical function, so that the same function name can be used to call functions accepting different types of the arguments.Random number generation
The headerstdlib.h
(cstdlib
in C++) defines several functions that can be used for statistically random number generationrand
- generates a pseudo-random numbersrand
- initializes a pseudo-random number generatorExternal links
- Dinkumware math.h reference, a reference of all
math.h
functions - C++ reference for math functions inherited from C