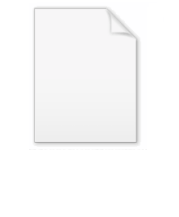
Hello world program examples
Encyclopedia
The Hello world program
is a simple computer program
that prints (or displays) the string "Hello World". It is typically one of the simplest programs possible in almost all computer languages, and often used as first program to demonstrate a programming language. As such it can be used to quickly compare syntax differences between various programming languages. The following is a list of canonical hello world programs in many programming languages.
trace ("Hello World!");
or (if you want it to show on the stage)
package com.example
{
import flash.text.TextField;
import flash.display.Sprite;
public class Greeter extends Sprite
{
public function Greeter
{
var txtHello:TextField = new TextField;
txtHello.text = "Hello World";
addChild(txtHello);
}
}
}
with Ada.Text_Io;
procedure Hello_World is
begin
Ada.Text_Io.Put_Line("Hello World!");
end;
Display("Hello, World!");
or (if you want to draw it to the background surface)
DrawingSurface *surface = Room.GetDrawingSurfaceForBackground;
surface.DrawString(0, 100, Game.NormalFont, "Hello, World!");
surface.Release;
print("Hello world!")
The output file is 22 bytes.
14 bytes are taken by "Hello, world!$
Written by Stewart Moss - May 2006
This is a .COM file so the CS and DS are in the same segment
I assembled and linked using TASM
tasm /m3 /zn /q hello.asm
tlink /t hello.obj
.model tiny
.code
org 100h
main proc
mov ah,9 ; Display String Service
mov dx,offset hello_message ; Offset of message (Segment DS is the right segment in .COM files)
int 21h ; call DOS int 21h service to display message at ptr ds:dx
retn ; returns to address 0000 off the stack
; which points to bytes which make int 20h (exit program)
hello_message db 'Hello, World!$'
main endp
end main
This program displays "Hello, World!" in a windows messagebox and then quits.
Written by Stewart Moss - May 2006
Assemble using TASM 5.0 and TLINK32
The ouput EXE is standard 4096 bytes long.
It is possible to produce really small windows PE exe files, but that
is outside of the scope of this demo.
.486p
.model flat,STDCALL
include win32.inc
extrn MessageBoxA:PROC
extrn ExitProcess:PROC
.data
HelloWorld db "Hello, World!",0
msgTitle db "Hello world program",0
.code
Start:
push MB_ICONQUESTION + MB_APPLMODAL + MB_OK
push offset msgTitle
push offset HelloWorld
push 0
call MessageBoxA
push 0
call ExitProcess
ends
end Start
BEGIN { print "Hello world!" }
PRINT "Hello world!"
@ECHO off
ECHO Hello World!
PAUSE
@ECHO on
+++++ +++++ initialize counter (cell #0) to 10
[ use loop to set the next four cells to 70/100/30/10
> +++++ ++ add 7 to cell #1
> +++++ +++++ add 10 to cell #2
> +++ add 3 to cell #3
> + add 1 to cell #4
<<<< - decrement counter (cell #0)
]
> ++ . print 'H'
> + . print 'e'
+++++ ++ . print 'l'
. print 'l'
+++ . print 'o'
> ++ . print ' '
<< +++++ +++++ +++++ . print 'W'
> . print 'o'
+++ . print 'r'
----- - . print 'l'
----- --- . print 'd'
> + . print '!'
> . print '\n'
int main(void)
{
printf("Hello world\n");
return 0;
}
int main
{
std::cout << "Hello World!" << std::endl;
return 0;
}
using System;
class ExampleClass
{
static void Main(string[] args)
{
Console.WriteLine("Hello, world!");
}
}
variables.greeting = "Hello, world!";
WriteOutput( variables.greeting );
CFML Tags:
#variables.greeting#
(println "Hello, world!")
GUI version:
(javax.swing.JOptionPane/showMessageDialog nil "Hello, world!")
IDENTIFICATION DIVISION.
PROGRAM-ID. HELLO-WORLD.
PROCEDURE DIVISION.
DISPLAY 'Hello, world'.
STOP RUN.
import std.stdio;
void main
{
writeln("Hello World!");
}
main
{
print('Hello World!');
}
{$APPTYPE CONSOLE}
begin
Writeln('Hello, world!');
end.
io:format("~s~n", ["hello, world"])
printfn "Hello World!"
program hello
print *, "Hello World"
end program hello
str='Hello World'
//Using the show_message function:
show_message(str);
//Using the draw_text function:
draw_text(320,240,str);
package main
import "fmt"
func main {
fmt.Println("Hello World!")
}
println "Hello World!"
main = print "Hello World!"
"Hello world!" println
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello world!");
}
}
document.write('Hello world!');
or
alert('Hello world!');
or
console.log('Hello world!');
or (when using node.js)
var util=require('util');
util.puts('Hello world!');
(print "Hello world!")
print("Hello World!")
Print["Hello World!"]
disp('Hello World!')
echo -a Hello World!
int main(void)
{
printf("Hello, World!\n");
return 0;
}
print_endline "Hello world!"
:
server = Server.one_page_server("Hello", ( -> <>Hello world!>))
program HelloWorld;
begin
WriteLn('Hello world!');
end.
print "Hello World.";
Or
use v5.10;
say 'Hello World.';
echo "Hello, world";
?>
SET SERVEROUTPUT ON;
BEGIN
DBMS_OUTPUT.PUT_LINE('Hello world!');
END;
/
Write-Host "Hello world!"
main :- write('Hello world!'), nl.
print 'Hello World'
print("Hello World")
"Hello, World!"
Running this program produces
(printf "Hello, World!\n")
A "hello world" web server
using Racket's
(define (start request) (response/xexpr '(html (body "Hello World"))))}
<< "Hello World!" MSGBOX >>
puts "Hello world!"
object HelloWorld extends App {
println("Hello world!")
}
(display "Hello World")
echo Hello World
OutText ("Hello World!");
Outimage;
End;
Transcript show: 'Hello, world!'.
SELECT 'Hello world!' FROM DUAL -- DUAL is a standard table in Oracle.
SELECT 'Hello world!' -- This will work in SQL Server.
MsgBox "Hello, world!"
Module Module1
Sub Main
Console.WriteLine("Hello, world!")
End Sub
End Module
'non-console example:
Class Form1
Public Sub Form1_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
MsgBox("Hello, world!")
End Sub
End Class
Hello world program
A "Hello world" program is a computer program that outputs "Hello world" on a display device. Because it is typically one of the simplest programs possible in most programming languages, it is by tradition often used to illustrate to beginners the most basic syntax of a programming language, or to...
is a simple computer program
Computer program
A computer program is a sequence of instructions written to perform a specified task with a computer. A computer requires programs to function, typically executing the program's instructions in a central processor. The program has an executable form that the computer can use directly to execute...
that prints (or displays) the string "Hello World". It is typically one of the simplest programs possible in almost all computer languages, and often used as first program to demonstrate a programming language. As such it can be used to quickly compare syntax differences between various programming languages. The following is a list of canonical hello world programs in many programming languages.
ActionScript 3.0
trace ("Hello World!");
or (if you want it to show on the stage)
package com.example
{
import flash.text.TextField;
import flash.display.Sprite;
public class Greeter extends Sprite
{
public function Greeter
{
var txtHello:TextField = new TextField;
txtHello.text = "Hello World";
addChild(txtHello);
}
}
}
ADA
with Ada.Text_Io;
procedure Hello_World is
begin
Ada.Text_Io.Put_Line("Hello World!");
end;
Adventure Game Studio Script
Display("Hello, World!");
or (if you want to draw it to the background surface)
DrawingSurface *surface = Room.GetDrawingSurfaceForBackground;
surface.DrawString(0, 100, Game.NormalFont, "Hello, World!");
surface.Release;
Algol 68
print("Hello world!")
Assembly Language - x86 DOS
The output file is 22 bytes.
14 bytes are taken by "Hello, world!$
Written by Stewart Moss - May 2006
This is a .COM file so the CS and DS are in the same segment
I assembled and linked using TASM
tasm /m3 /zn /q hello.asm
tlink /t hello.obj
.model tiny
.code
org 100h
main proc
mov ah,9 ; Display String Service
mov dx,offset hello_message ; Offset of message (Segment DS is the right segment in .COM files)
int 21h ; call DOS int 21h service to display message at ptr ds:dx
retn ; returns to address 0000 off the stack
; which points to bytes which make int 20h (exit program)
hello_message db 'Hello, World!$'
main endp
end main
Assembly Language - x86 Windows 32 bit
This program displays "Hello, World!" in a windows messagebox and then quits.
Written by Stewart Moss - May 2006
Assemble using TASM 5.0 and TLINK32
The ouput EXE is standard 4096 bytes long.
It is possible to produce really small windows PE exe files, but that
is outside of the scope of this demo.
.486p
.model flat,STDCALL
include win32.inc
extrn MessageBoxA:PROC
extrn ExitProcess:PROC
.data
HelloWorld db "Hello, World!",0
msgTitle db "Hello world program",0
.code
Start:
push MB_ICONQUESTION + MB_APPLMODAL + MB_OK
push offset msgTitle
push offset HelloWorld
push 0
call MessageBoxA
push 0
call ExitProcess
ends
end Start
AWK
BEGIN { print "Hello world!" }
BASIC
PRINT "Hello world!"
Batch File
@ECHO off
ECHO Hello World!
PAUSE
@ECHO on
brainfuck
+++++ +++++ initialize counter (cell #0) to 10
[ use loop to set the next four cells to 70/100/30/10
> +++++ ++ add 7 to cell #1
> +++++ +++++ add 10 to cell #2
> +++ add 3 to cell #3
> + add 1 to cell #4
<<<< - decrement counter (cell #0)
]
> ++ . print 'H'
> + . print 'e'
+++++ ++ . print 'l'
. print 'l'
+++ . print 'o'
> ++ . print ' '
<< +++++ +++++ +++++ . print 'W'
> . print 'o'
+++ . print 'r'
----- - . print 'l'
----- --- . print 'd'
> + . print '!'
> . print '\n'
C
- include
int main(void)
{
printf("Hello world\n");
return 0;
}
C++
- include
int main
{
std::cout << "Hello World!" << std::endl;
return 0;
}
C#
using System;
class ExampleClass
{
static void Main(string[] args)
{
Console.WriteLine("Hello, world!");
}
}
CFML (ColdFusion)
CF Script:variables.greeting = "Hello, world!";
WriteOutput( variables.greeting );
CFML Tags:
Clojure
Console version:(println "Hello, world!")
GUI version:
(javax.swing.JOptionPane/showMessageDialog nil "Hello, world!")
COBOL
IDENTIFICATION DIVISION.
PROGRAM-ID. HELLO-WORLD.
PROCEDURE DIVISION.
DISPLAY 'Hello, world'.
STOP RUN.
D
import std.stdio;
void main
{
writeln("Hello World!");
}
Dart
main
{
print('Hello World!');
}
Delphi
begin
Writeln('Hello, world!');
end.
Erlang
io:format("~s~n", ["hello, world"])
F#
printfn "Hello World!"
Fortran
program hello
print *, "Hello World"
end program hello
Game Maker Language
str='Hello World'
//Using the show_message function:
show_message(str);
//Using the draw_text function:
draw_text(320,240,str);
Go
package main
import "fmt"
func main {
fmt.Println("Hello World!")
}
Groovy
println "Hello World!"
Haskell
main = print "Hello World!"
Io
"Hello world!" println
Java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello world!");
}
}
JavaScript
document.write('Hello world!');
or
alert('Hello world!');
or
console.log('Hello world!');
or (when using node.js)
var util=require('util');
util.puts('Hello world!');
Lisp
(print "Hello world!")
Lua
print("Hello World!")
Mathematica
Print["Hello World!"]
MATLAB
disp('Hello World!')
mIRC Script
echo -a Hello World!
ObjectiveC
- import
int main(void)
{
printf("Hello, World!\n");
return 0;
}
OCaml
print_endline "Hello world!"
Opa
A "hello world" web serverWeb server
Web server can refer to either the hardware or the software that helps to deliver content that can be accessed through the Internet....
:
server = Server.one_page_server("Hello", ( -> <>Hello world!>))
Pascal
program HelloWorld;
begin
WriteLn('Hello world!');
end.
Perl 5
print "Hello World.";
Or
use v5.10;
say 'Hello World.';
PHP
echo "Hello, world";
?>
PL/SQL
SET SERVEROUTPUT ON;
BEGIN
DBMS_OUTPUT.PUT_LINE('Hello world!');
END;
/
PowerShell
Write-Host "Hello world!"
Prolog
main :- write('Hello world!'), nl.
Python 2
print 'Hello World'
Python 3
print("Hello World")
Racket
Trivial "hello world" program:- lang racket
"Hello, World!"
Running this program produces
"Hello, World!"
. More direct version:- lang racket
(printf "Hello, World!\n")
A "hello world" web server
Web server
Web server can refer to either the hardware or the software that helps to deliver content that can be accessed through the Internet....
using Racket's
web-server/insta
language:- lang web-server/insta
(define (start request) (response/xexpr '(html (body "Hello World"))))}
RPL
<< "Hello World!" MSGBOX >>
RTL/2
TITLE Hello World;
LET NL=10;
EXT PROC(REF ARRAY BYTE) TWRT;
ENT PROC INT RRJOB;
TWRT("Hello World!#NL#");
RETURN(1);
ENDPROC;
Ruby
puts "Hello world!"
Scala
object HelloWorld extends App {
println("Hello world!")
}
Scheme
(display "Hello World")
Shell
echo Hello World
Simula
BeginOutText ("Hello World!");
Outimage;
End;
Smalltalk
Transcript show: 'Hello, world!'.
SQL
SELECT 'Hello world!' FROM DUAL -- DUAL is a standard table in Oracle.
SELECT 'Hello world!' -- This will work in SQL Server.
Visual Basic
MsgBox "Hello, world!"
Visual Basic .NET
Module Module1
Sub Main
Console.WriteLine("Hello, world!")
End Sub
End Module
'non-console example:
Class Form1
Public Sub Form1_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
MsgBox("Hello, world!")
End Sub
End Class