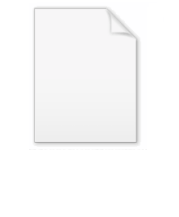
Dispatch table
Encyclopedia
In computer science
, a dispatch table is a table of pointers to functions or method
s. Use of such a table is a common technique when implementing late binding
in object-oriented programming
.
, using a hash to store references to code (also known as function pointer
s).
Running this Perl program as
programming language
s that support virtual methods, the compiler
will automatically create a dispatch table for each object of a class
containing virtual methods. This table is called a virtual method table
or vtable, and every call to a virtual method is dispatched through the vtable.
Computer science
Computer science or computing science is the study of the theoretical foundations of information and computation and of practical techniques for their implementation and application in computer systems...
, a dispatch table is a table of pointers to functions or method
Method (computer science)
In object-oriented programming, a method is a subroutine associated with a class. Methods define the behavior to be exhibited by instances of the associated class at program run time...
s. Use of such a table is a common technique when implementing late binding
Late binding
Late binding is a computer programming mechanism in which the method being called upon an object is looked up by name at runtime. This is informally known as duck typing or name binding....
in object-oriented programming
Object-oriented programming
Object-oriented programming is a programming paradigm using "objects" – data structures consisting of data fields and methods together with their interactions – to design applications and computer programs. Programming techniques may include features such as data abstraction,...
.
Perl implementation
The following shows one way to implement a dispatch table in PerlPerl
Perl is a high-level, general-purpose, interpreted, dynamic programming language. Perl was originally developed by Larry Wall in 1987 as a general-purpose Unix scripting language to make report processing easier. Since then, it has undergone many changes and revisions and become widely popular...
, using a hash to store references to code (also known as function pointer
Function pointer
A function pointer is a type of pointer in C, C++, D, and other C-like programming languages, and Fortran 2003. When dereferenced, a function pointer can be used to invoke a function and pass it arguments just like a normal function...
s).
#define the table using one anonymous code-ref and one named code-ref
my %dispatch = (
"-h" => sub { return "hello\n"; },
"-g" => \&say_goodbye
);
sub say_goodbye {
return "goodbye\n";
}
#fetch the code ref from the table, and invoke it
my $sub = $dispatch{$ARGV[0]};
print $sub ? $sub-> : "unknown argument\n";
Running this Perl program as
perl greet -h
will produce "hello", and running it as perl greet -g
will produce "goodbye".Virtual method tables
In object-orientedObject-oriented programming
Object-oriented programming is a programming paradigm using "objects" – data structures consisting of data fields and methods together with their interactions – to design applications and computer programs. Programming techniques may include features such as data abstraction,...
programming language
Programming language
A programming language is an artificial language designed to communicate instructions to a machine, particularly a computer. Programming languages can be used to create programs that control the behavior of a machine and/or to express algorithms precisely....
s that support virtual methods, the compiler
Compiler
A compiler is a computer program that transforms source code written in a programming language into another computer language...
will automatically create a dispatch table for each object of a class
Class (computer science)
In object-oriented programming, a class is a construct that is used as a blueprint to create instances of itself – referred to as class instances, class objects, instance objects or simply objects. A class defines constituent members which enable these class instances to have state and behavior...
containing virtual methods. This table is called a virtual method table
Virtual method table
A virtual method table, virtual function table, dispatch table, or vtable, is a mechanism used in a programming language to support dynamic dispatch ....
or vtable, and every call to a virtual method is dispatched through the vtable.