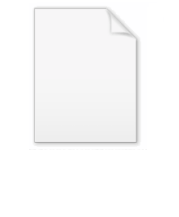
Common operator notation
Encyclopedia
In programming languages, common operator notation is one way of notating mathematical expressions as a linear sequence of tokens, or operator
s.
In this model, tokens are divided into two classes: operators and operands.
Operands are mathematical objects upon which the operators operate. These include number
s such as 3 or 1001, truth values such as true or false, structures such as vectors, or any other mathematical object. One special type of operand is the parenthesis group. An expression enclosed in parentheses is evaluated recursively and treated, for operator association purposes, as a single operand.
Each operator is given a position, precedence, and an associativity. The precedence is a number, and operator precedence is usually ordered with the corresponding number order, although some implementations give higher precedences lower numbers. In cases in which two operators of different precedences compete for the same operand, the operator with the higher precedence wins. For example, '×' has a higher precedence than '+', so 3+4×5 = (3+(4×5)), not ((3+4)×5).
Operator position indicates where, in the sequence, the operator appears. In terms of operator position, an operator may be prefix, postfix, or infix. A prefix operator immediately precedes its operand, as in "−x". A postfix operator immediately succeeds its operand, as in "x!". An infix operator exists between its left and right operands, as in "A + B".
Operator associativity
, loosely speaking, describes what operators are allowed to associate before associating with the operator in question. In those cases in which operators of equal precedence compete for common operands, operator associativity describes the order of operator association. An infix operator can be left-associative, right-associative, or non-associative. A prefix or postfix operator can be either associative or non-associative. If an operator is left-associative, the operators are applied in left-to-right order. The arithmetic operators '+', '−', '×', and '÷', for example, are all left-associative. That is,

If an operator is right-associative, the operators are applied in right-to-left order. In the Java programming language
, the assignment operator "=" is right-associative. That is, the Java statement "a = b = c;" is equivalent to "(a = (b = c));". It first assigns the value of c to
b, then assigns the value of b to a. An operator which is non-associative cannot compete for operands with operators of equal precedence. For example, in Prolog
, the infix operator ":-" is non-associative, so constructs such as "a :- b :- c" constitute syntax errors. There may nonetheless be a use for such constructs as "a =|> b =|> c".
A prefix or postfix operator is associative if and only if it may compete for operands with operators of equal precedence. The unary prefix operators '+', '−', and 'sin', for example, are all associative prefix operators. The unary postfix operator '!' and, in the C
language, the post-increment and post-decrement operators
"++" and "--" are examples of associative postfix operators. When more than one associative prefix or postfix operator of equal precedence precedes or succeeds an operand, the operators closer to the operand associate first. For example, −sin x = −(sin(x)) and, in C
, the expression "x++--" is equivalent to "(x++)--". When prefix and postfix operators of equal precedence coexist, the order of association is undefined.
Note that, contrary to popular belief, prefix and postfix operators do not necessarily have higher precedence than all infix operators. For example, if the prefix operator 'sin' is given a precedence between that of '+' and '×',

not

or

The rules for expression evaluation are simple:
Note that an infix operator need not be binary. C
, for example, has a ternary infix operator "?:
".
Examples:
Operator (programming)
Programming languages typically support a set of operators: operations which differ from the language's functions in calling syntax and/or argument passing mode. Common examples that differ by syntax are mathematical arithmetic operations, e.g...
s.
In this model, tokens are divided into two classes: operators and operands.
Operands are mathematical objects upon which the operators operate. These include number
Number
A number is a mathematical object used to count and measure. In mathematics, the definition of number has been extended over the years to include such numbers as zero, negative numbers, rational numbers, irrational numbers, and complex numbers....
s such as 3 or 1001, truth values such as true or false, structures such as vectors, or any other mathematical object. One special type of operand is the parenthesis group. An expression enclosed in parentheses is evaluated recursively and treated, for operator association purposes, as a single operand.
Each operator is given a position, precedence, and an associativity. The precedence is a number, and operator precedence is usually ordered with the corresponding number order, although some implementations give higher precedences lower numbers. In cases in which two operators of different precedences compete for the same operand, the operator with the higher precedence wins. For example, '×' has a higher precedence than '+', so 3+4×5 = (3+(4×5)), not ((3+4)×5).
Operator position indicates where, in the sequence, the operator appears. In terms of operator position, an operator may be prefix, postfix, or infix. A prefix operator immediately precedes its operand, as in "−x". A postfix operator immediately succeeds its operand, as in "x!". An infix operator exists between its left and right operands, as in "A + B".
Operator associativity
Operator associativity
In programming languages and mathematical notation, the associativity of an operator is a property that determines how operators of the same precedence are grouped in the absence of parentheses...
, loosely speaking, describes what operators are allowed to associate before associating with the operator in question. In those cases in which operators of equal precedence compete for common operands, operator associativity describes the order of operator association. An infix operator can be left-associative, right-associative, or non-associative. A prefix or postfix operator can be either associative or non-associative. If an operator is left-associative, the operators are applied in left-to-right order. The arithmetic operators '+', '−', '×', and '÷', for example, are all left-associative. That is,

If an operator is right-associative, the operators are applied in right-to-left order. In the Java programming language
Java (programming language)
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems' Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities...
, the assignment operator "=" is right-associative. That is, the Java statement "a = b = c;" is equivalent to "(a = (b = c));". It first assigns the value of c to
b, then assigns the value of b to a. An operator which is non-associative cannot compete for operands with operators of equal precedence. For example, in Prolog
Prolog
Prolog is a general purpose logic programming language associated with artificial intelligence and computational linguistics.Prolog has its roots in first-order logic, a formal logic, and unlike many other programming languages, Prolog is declarative: the program logic is expressed in terms of...
, the infix operator ":-" is non-associative, so constructs such as "a :- b :- c" constitute syntax errors. There may nonetheless be a use for such constructs as "a =|> b =|> c".
A prefix or postfix operator is associative if and only if it may compete for operands with operators of equal precedence. The unary prefix operators '+', '−', and 'sin', for example, are all associative prefix operators. The unary postfix operator '!' and, in the C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
language, the post-increment and post-decrement operators
"++" and "--" are examples of associative postfix operators. When more than one associative prefix or postfix operator of equal precedence precedes or succeeds an operand, the operators closer to the operand associate first. For example, −sin x = −(sin(x)) and, in C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
, the expression "x++--" is equivalent to "(x++)--". When prefix and postfix operators of equal precedence coexist, the order of association is undefined.
Note that, contrary to popular belief, prefix and postfix operators do not necessarily have higher precedence than all infix operators. For example, if the prefix operator 'sin' is given a precedence between that of '+' and '×',

not

or

The rules for expression evaluation are simple:
- Treat any sub-expression in parentheses as a single recursively-evaluated operand.
- Associate operands with operators of higher precedence before those of lower precedence.
- Among operators of equal precedence, associate operands with operators according to the associativity of the operators.
Note that an infix operator need not be binary. C
C (programming language)
C is a general-purpose computer programming language developed between 1969 and 1973 by Dennis Ritchie at the Bell Telephone Laboratories for use with the Unix operating system....
, for example, has a ternary infix operator "?:
?:
In computer programming, ?: is a ternary operator that is part of the syntax for a basic conditional expression in several programming languages...
".
Examples:
- 1 + 2 + 3 * 4 * 5 + 6 + 7 = ((((1 + 2) + ((3 * 4) * 5)) + 6) + 7)
- 4 + -x + 3 = ((4 + (-x)) + 3)
- 4 * 3! = (4 * (3!))
- a++-- = (a++)--
- -3! = -(3!)
Generalizations of Common Operator Notation
The use of operator precedence classes and associativities is just one way. However, it is not the most general way: this model cannot give an operator more precedence when competing with '−' than it can when competing with '+', while still giving '+' and '−' equivalent precedences and associativities. A generalized version of this model (in which each operator can be given independent left and right precedences) can be found at http://compilers.iecc.com/comparch/article/01-07-068.See also
- Operator (programming)Operator (programming)Programming languages typically support a set of operators: operations which differ from the language's functions in calling syntax and/or argument passing mode. Common examples that differ by syntax are mathematical arithmetic operations, e.g...
- Order of operationsOrder of operationsIn mathematics and computer programming, the order of operations is a rule used to clarify unambiguously which procedures should be performed first in a given mathematical expression....
- Relational operatorRelational operatorIn computer science, a relational operator is a programming language construct or operator that tests or defines some kind of relation between two entities. These include numerical equality and inequalities...
- Operators in C and C++Operators in C and C++This is a list of operators in the C and C++ programming languages. All the operators listed exist in C++; the fourth column "Included in C", dictates whether an operator is also present in C...