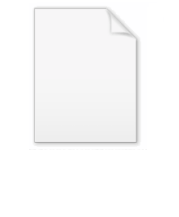
Clamping (graphics)
Encyclopedia
In computer graphics, clamping is the process of limiting a position to an area. Unlike wrapping
, clamping merely moves the point to the nearest available value.
To put clamping into perspective, pseudocode
for clamping is:
function clamp(X, Min, Max:Real):Real;
if X > Max then
X := Max;
if X < Min then
X := Min;
return X;
In JavaScript
:
function clamp(value, min, max) {
return Math.min(Math.max(value, min), max);
};
A faster one in JavaScript
using ternary operators
:
function clamp(value, min, max) {
return value < min ? min : value > max ? max : value;
}
C and C++: (int
can be changed into any other datatype):
int clamp(int X, int Min, int Max)
{
if( X > Max )
X = Max;
else if( X < Min )
X = Min;
return X;
}
or
int clamp(int X, int Min, int Max) {
return ( X > Max ) ? Max : ( X < Min ) ? Min : X;
}
or
int clamp(int X, int Min, int Max)
{
if ( X > Max ) return Max;
if ( X < Min ) return Min;
return X;
}
or, using templates to cover all data types (C++ only)
template<typename T>
T clamp(T Value, T Min, T Max)
{
return (Value < Min)? Min : (Value > Max)? Max : Value;
}
is the placing of a detail inside a polygon—for example, a bullethole on a wall. It can also be used with wrapping
to create a variety of effects.
Can also mean clamped to a certain value. For example, in OpenGL, the glClearColor takes a GLclampf value which is a gl float value 'clamped' to the range [0,1].
Wrapping (graphics)
In computer graphics, wrapping is the process of limiting a position to an area. A good example of wrapping is wallpaper, a single pattern repeated indefinitely over a wall...
, clamping merely moves the point to the nearest available value.
To put clamping into perspective, pseudocode
Pseudocode
In computer science and numerical computation, pseudocode is a compact and informal high-level description of the operating principle of a computer program or other algorithm. It uses the structural conventions of a programming language, but is intended for human reading rather than machine reading...
for clamping is:
function clamp(X, Min, Max:Real):Real;
if X > Max then
X := Max;
if X < Min then
X := Min;
return X;
In JavaScript
JavaScript
JavaScript is a prototype-based scripting language that is dynamic, weakly typed and has first-class functions. It is a multi-paradigm language, supporting object-oriented, imperative, and functional programming styles....
:
function clamp(value, min, max) {
return Math.min(Math.max(value, min), max);
};
A faster one in JavaScript
JavaScript
JavaScript is a prototype-based scripting language that is dynamic, weakly typed and has first-class functions. It is a multi-paradigm language, supporting object-oriented, imperative, and functional programming styles....
using ternary operators
?:
In computer programming, ?: is a ternary operator that is part of the syntax for a basic conditional expression in several programming languages...
:
function clamp(value, min, max) {
return value < min ? min : value > max ? max : value;
}
C and C++: (int
Integer (computer science)
In computer science, an integer is a datum of integral data type, a data type which represents some finite subset of the mathematical integers. Integral data types may be of different sizes and may or may not be allowed to contain negative values....
can be changed into any other datatype):
int clamp(int X, int Min, int Max)
{
if( X > Max )
X = Max;
else if( X < Min )
X = Min;
return X;
}
or
int clamp(int X, int Min, int Max) {
return ( X > Max ) ? Max : ( X < Min ) ? Min : X;
}
or
int clamp(int X, int Min, int Max)
{
if ( X > Max ) return Max;
if ( X < Min ) return Min;
return X;
}
or, using templates to cover all data types (C++ only)
template<typename T>
T clamp(T Value, T Min, T Max)
{
return (Value < Min)? Min : (Value > Max)? Max : Value;
}
Uses
One of the many uses of clamping in computer graphics3D computer graphics
3D computer graphics are graphics that use a three-dimensional representation of geometric data that is stored in the computer for the purposes of performing calculations and rendering 2D images...
is the placing of a detail inside a polygon—for example, a bullethole on a wall. It can also be used with wrapping
Wrapping (graphics)
In computer graphics, wrapping is the process of limiting a position to an area. A good example of wrapping is wallpaper, a single pattern repeated indefinitely over a wall...
to create a variety of effects.
Can also mean clamped to a certain value. For example, in OpenGL, the glClearColor takes a GLclampf value which is a gl float value 'clamped' to the range [0,1].