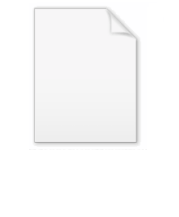
Call site
Encyclopedia
In programming, a call site of a function (subroutine) is a line in the code which calls (or may call, through dynamic dispatch
) a function. A call site passes zero or more arguments to the function, and receives zero or more return values.
function sqr(x)
{
return x * x;
}
// these are two call sites of the function
a = sqr(b);
c = sqr(b);
* (usually) external call.... R13 usually points to a save area for general purpose registers beforehand
* and R1 points to a list of addresses of parameters (if any)
LA R1,=A(B) point to (address of) variable 'B'
L R15,=A(SQR) Load pointer (address constant
) to separately compiled/assembled subroutine
BALR R14,R15 Go to subroutine, which returns - usually at zero displacement on R14
* internal call (usually much smaller overhead and possibly 'known' parameters)
BAL R14,SQR Go to program label and return
Sometimes, as an efficient method of indicating success or otherwise, return may be accomplished by returning at +0 or +4,+8 etc. requiring a small branch table
at the return point - to go directly to process the case (as in HLL
Switch statement
).
BAL R14,SQR Go to program label and return (using offset on R14 as return address)
B FAIL (RET+0) - SOMETHING WRONG
* (RET+4) - O.K.
Conventionally however, a return code is set in R15 (0=OK, 4= failure, or similar ..) but requiring a separate instruction to test R15 or use directly as a branch index.
Dynamic dispatch
In computer science, dynamic dispatch is the process of mapping a message to a specific sequence of code at runtime. This is done to support the cases where the appropriate method can't be determined at compile-time...
) a function. A call site passes zero or more arguments to the function, and receives zero or more return values.
Example
// this is a function definitionfunction sqr(x)
{
return x * x;
}
// these are two call sites of the function
a = sqr(b);
c = sqr(b);
Assembler example
IBM/360 or Z/ArchitectureZ/Architecture
z/Architecture, initially and briefly called ESA Modal Extensions , refers to IBM's 64-bit computing architecture for IBM mainframe computers. IBM introduced its first z/Architecture-based system, the zSeries Model 900, in late 2000. Later z/Architecture systems include the IBM z800, z990, z890,...
* (usually) external call.... R13 usually points to a save area for general purpose registers beforehand
* and R1 points to a list of addresses of parameters (if any)
LA R1,=A(B) point to (address of) variable 'B'
L R15,=A(SQR) Load pointer (address constant
Address constant
In IBM/360 , an address constant or "adcon" is an Assembly language data type whose value refers directly to another value stored elsewhere in the computer memory using its address. An address constant can be one, two, three or four bytes long...
) to separately compiled/assembled subroutine
BALR R14,R15 Go to subroutine, which returns - usually at zero displacement on R14
* internal call (usually much smaller overhead and possibly 'known' parameters)
BAL R14,SQR Go to program label and return
Sometimes, as an efficient method of indicating success or otherwise, return may be accomplished by returning at +0 or +4,+8 etc. requiring a small branch table
Branch table
In computer programming, a branch table is a term used to describe an efficient method of transferring program control to another part of a program using a table of branch instructions. It is a form of multiway branch...
at the return point - to go directly to process the case (as in HLL
High-level programming language
A high-level programming language is a programming language with strong abstraction from the details of the computer. In comparison to low-level programming languages, it may use natural language elements, be easier to use, or be from the specification of the program, making the process of...
Switch statement
Switch statement
In computer programming, a switch, case, select or inspect statement is a type of selection control mechanism that exists in most imperative programming languages such as Pascal, Ada, C/C++, C#, Java, and so on. It is also included in several other types of languages...
).
BAL R14,SQR Go to program label and return (using offset on R14 as return address)
B FAIL (RET+0) - SOMETHING WRONG
* (RET+4) - O.K.
Conventionally however, a return code is set in R15 (0=OK, 4= failure, or similar ..) but requiring a separate instruction to test R15 or use directly as a branch index.
See also
- Function inlining
- subroutineSubroutineIn computer science, a subroutine is a portion of code within a larger program that performs a specific task and is relatively independent of the remaining code....